This will be a menu-driven program. The menu should loðk IIKE IS. Menu ==== 1. Play rock-paper-scissors 2. Play Yahtzee 3. Exit Enter your choice: Specs for the menu 1. The program should continue to run until the user chooses #3 from the menu. 2. If the user enters a non-valid menu choice (for example, 5) your program should print an error message that explains (in a complete sentence) what happened. It should keep running, though. 3. You should clear the screen each time before printing the menu. To do that, use this code: cout << "Press to continue" « endl; cin.get (); system ("cls"); 4. The menu should use a switch statement to process the user's input. Play rock-paper-scissors specs Two players choose either rock, paper, or scissors and compare their choices. rock beats scissors (rock breaks or dulls scissors) b. scissors beats paper (scissors cut paper) 1. a. C. paper bears rock (paper covers rock) Ask the user for the number of game wins it will take to win a match; this must be an odd number. Use a loop to validate user input to make sure s/he enters an odd number. Now your program will generate the choices for two players by using a random number generator to simulate the players' "choices" (of rock, paper, or scissors). Review random numbers in section 3.9 in the text. 4. Make sure you "seed" the random number generator as shown in Program 3-25. 5. You will be printing out each game in the match and telling the score after each game. A match is one or more games. Suppose the user entered that it will take 3 game wins to win the match. The output would look similar to this: 2. 3. game #1 playerl : rock player2 : scissors playerl = 1, player2 = 0 game #2 player 1 : paper player 2 : paper playerl = 1, player2 = 0 Notice this game was a tie, so the scores didn't change дame #3 player 1 : paper player 2: scissors playerl = 1, player2 = 1
This will be a menu-driven program. The menu should loðk IIKE IS. Menu ==== 1. Play rock-paper-scissors 2. Play Yahtzee 3. Exit Enter your choice: Specs for the menu 1. The program should continue to run until the user chooses #3 from the menu. 2. If the user enters a non-valid menu choice (for example, 5) your program should print an error message that explains (in a complete sentence) what happened. It should keep running, though. 3. You should clear the screen each time before printing the menu. To do that, use this code: cout << "Press to continue" « endl; cin.get (); system ("cls"); 4. The menu should use a switch statement to process the user's input. Play rock-paper-scissors specs Two players choose either rock, paper, or scissors and compare their choices. rock beats scissors (rock breaks or dulls scissors) b. scissors beats paper (scissors cut paper) 1. a. C. paper bears rock (paper covers rock) Ask the user for the number of game wins it will take to win a match; this must be an odd number. Use a loop to validate user input to make sure s/he enters an odd number. Now your program will generate the choices for two players by using a random number generator to simulate the players' "choices" (of rock, paper, or scissors). Review random numbers in section 3.9 in the text. 4. Make sure you "seed" the random number generator as shown in Program 3-25. 5. You will be printing out each game in the match and telling the score after each game. A match is one or more games. Suppose the user entered that it will take 3 game wins to win the match. The output would look similar to this: 2. 3. game #1 playerl : rock player2 : scissors playerl = 1, player2 = 0 game #2 player 1 : paper player 2 : paper playerl = 1, player2 = 0 Notice this game was a tie, so the scores didn't change дame #3 player 1 : paper player 2: scissors playerl = 1, player2 = 1
Chapter5: Looping
Section: Chapter Questions
Problem 12E
Related questions
Question
Instructions for the assignment can be found in the picture linked to this post. The
Program 3.25
// This program demonstrates random numbers.
#include <iostream>
#include <cstdlib> // For rand and srand
#include <ctime> // For the time function
using namespace std;
int main()
{
// Get the system time.
unsigned seed = time(0);
// Seed the random number generator.
srand(seed);
// Display three random numbers.
cout << rand() << endl;
cout << rand() << endl;
cout << rand() << endl;
return 0;
}
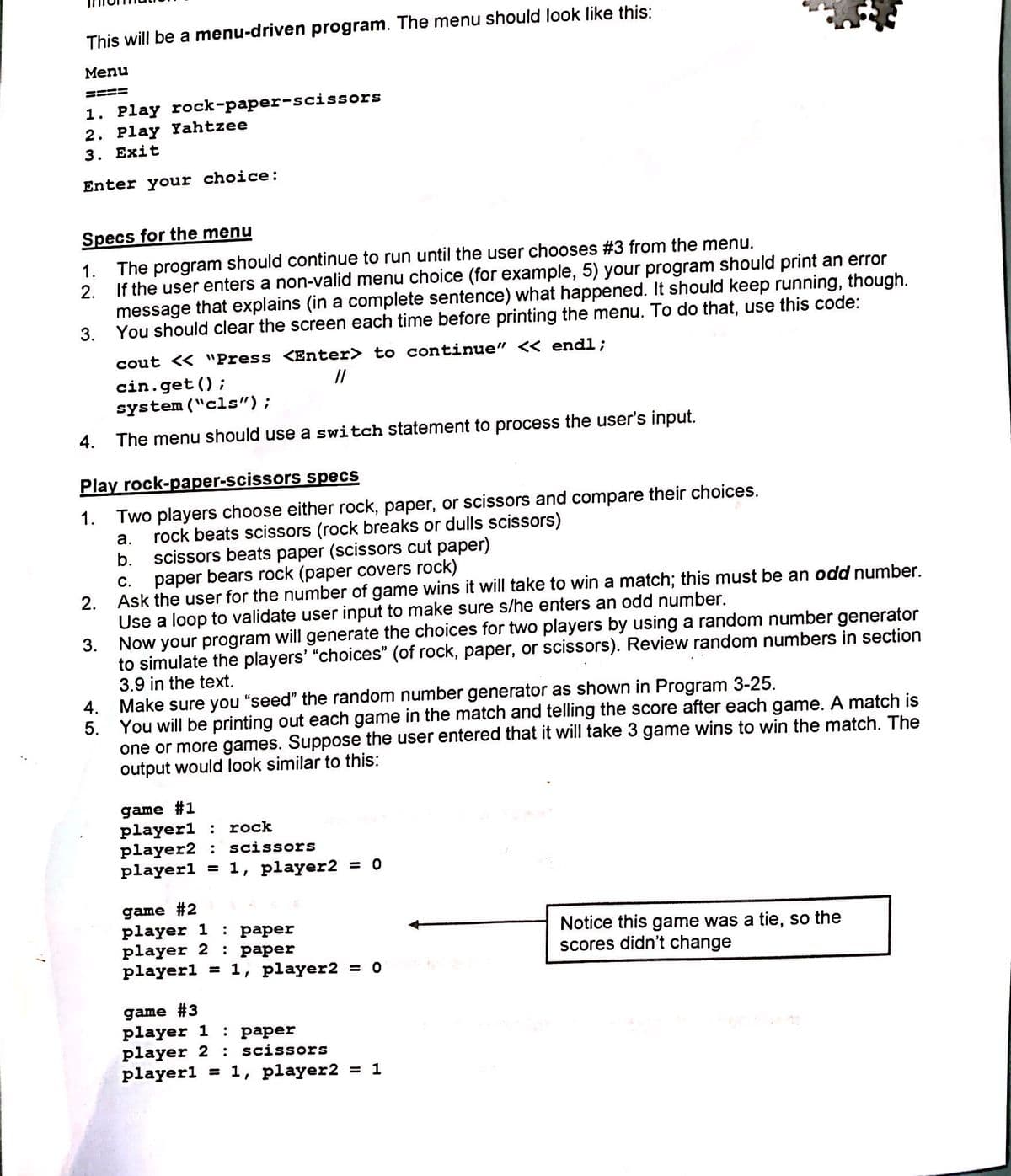
Transcribed Image Text:This will be a menu-driven program. The menu should look like this:
Menu
====
1. Play rock-paper-scissors
2. Play Yahtzee
3. Еxit
Enter your choice:
Specs for the menu
1. The program should continue to run until the user chooses #3 from the menu.
If the user enters a non-valid menu choice (for example, 5) your program should print an error
message that explains (in a complete sentence) what happened. It should keep running, though.
3. You should clear the screen each time before printing the menu. To do that, use this code:
2.
cout <« "Press <Enter> t o continue" << endl;
//
cin.get () ;
system ("cls");
4. The menu should use a switch statement to process the user's input.
Play rock-paper-scissors specs
1. Two players choose either rock, paper, or scissors and compare their choices.
rock beats scissors (rock breaks or dulls scissors)
а.
scissors beats paper (scissors cut paper)
b.
С.
paper bears rock (paper covers rock)
Ask the user for the number of game wins it will take to win a match; this must be an odd number.
Use a loop to validate user input to make sure s/he enters an odd number.
Now your program will generate the choices for two players by using a random number generator
to simulate the players' "choices" (of rock, paper, or scissors). Review random numbers in section
3.9 in the text.
2.
3.
4. Make sure you "seed" the random number generator as shown in Program 3-25.
5. You will be printing out each game in the match and telling the score after each game. A match is
one or more games. Suppose the user entered that it will take 3 game wins to win the match. The
output would look similar to this:
game #1
playerl :
player2 : scissors
playerl
rock
= 1, player2 = 0
game #2
player 1
player 2
playerl = 1, player2 = 0
: рарer
: paper
Notice this game was a tie, so the
scores didn't change
game #3
player 1
player 2
playerl = 1, player2
: раper
: scissors
%3D
= 1
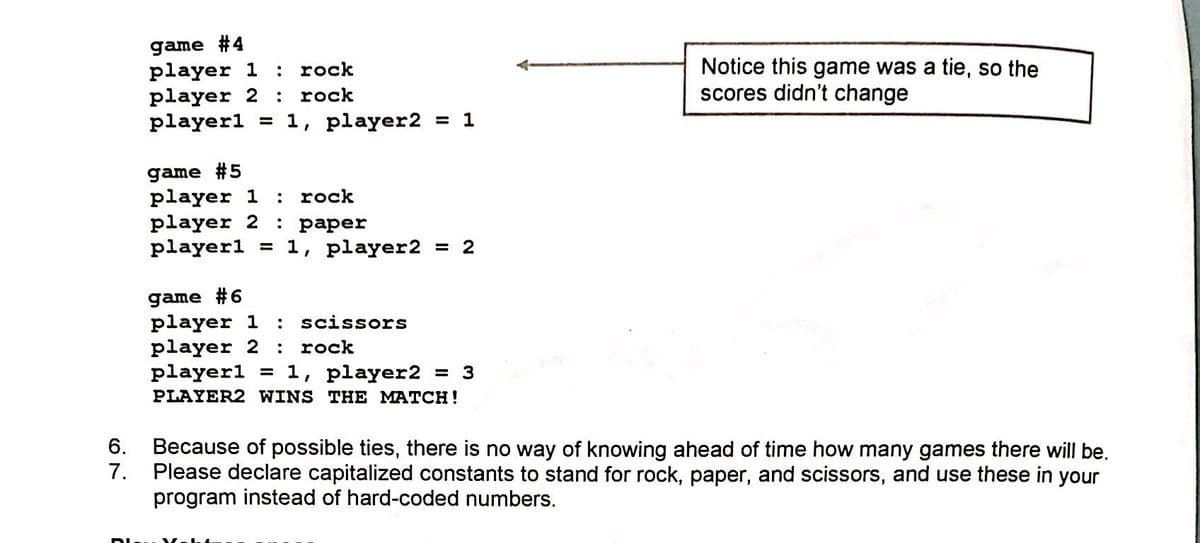
Transcribed Image Text:game #4
player 1
player 2
playerl
Notice this game was a tie, so the
scores didn't change
: rock
:
rock
= 1, player2 = 1
game #5
player 1
player 2
playerl = 1, player2 = 2
:
rock
: paper
game #6
player 1
player 2
playerl = 1, player2
PLAYER2 WINS THE MATCH!
scissors
rock
= 3
6.
Because of possible ties, there is no way of knowing ahead of time how many games there will be.
7.
Please declare capitalized constants to stand for rock, paper, and scissors, and use these in your
program instead of hard-coded numbers.
Dlu Y
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
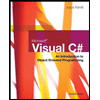
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
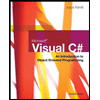
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,