this.pay_rate = pay_rate; //. getter nethod public int getShift() return this.shift; // getter nethod of get pay rate public double getPayRate() //return the pay rate return this.pay_rate; //create main method public class Main public static void nain(String(] args) //create the object of the class Productionworker pw = new Productionworker ("914-A", new Date(), 1, 20.36); System.out.println("Enployee Nunber : " + pw.getEmployeeNumber()); System.out.println("Hiring Date : " + pw.gethiringDate()): System.out.println("Shift : " + pw.getShift()); System.out.println ("Payrate : " + pw.getPayRate());
Please teach me how to fix an error from my JAVA source code.
I have set up the source code with class name "ProductionWorker" and there were a error occured on second page of sceenshot where it is highlighted.
//import the file
import java.util.*;
//create the class
class Employee
{
//create the variable
private String emp_num;
//create the date variable
private Date hiring_date;
// default constructor
public Employee()
{
//assign the emp num.
this.emp_num = "000-A";
//assign the Date
this.hiring_date = new Date();
}
// constructor parameter
public Employee(String emp_num, Date hiring_date)
{
this.emp_num = emp_num;
this.hiring_date = hiring_date;
}
// getter method
public String getEmployeeNumber()
{
return this.emp_num;
}
// call the gethiringdate function
public Date gethiringDate()
{
// return the date
return this.hiring_date;
}
// setter method
public void setEmployeeNumber(String emp_num)
{
//set the emp num.
this.emp_num = emp_num;
}
//set the hiring date
public void sethiringDate(Date hiring_date)
{
//return the hiring date
this.hiring_date = hiring_date;
}
}
//extends the class Employee
class ProductionWorker extends Employee
{
//declare the vairable
private int shift;
private double pay_rate;
// constructor
public ProductionWorker()
{
// call the cinstructor of the super class
super();
this.shift = 1;
this.pay_rate = 0.0;
}
// constructor
public ProductionWorker(String emp_num, Date hiring_date, int shift, double pay_rate)
{
// call the cinstructor of the super class
super(emp_num, hiring_date);
this.shift = shift;
this.pay_rate = pay_rate;
}
// setter method
public void setShift(int shift)
{
//return the shift
this.shift = shift;
}
//create set pay rate
public void setPayRate(double pay_rate)
{
//return the pay rate
this.pay_rate = pay_rate;
}
// getter method
public int getShift()
{
return this.shift;
}
// getter method of get pay rate
public double getPayRate()
{
//return the pay rate
return this.pay_rate;
}
}
//create main method
public class Main
{
public static void main(String[] args)
{
//create the object of the class
ProductionWorker pw = new ProductionWorker("914-A", new Date(), 1, 20.36);
System.out.println("Employee Number : " + pw.getEmployeeNumber());
System.out.println("Hiring Date : " + pw.gethiringDate());
System.out.println("Shift : " + pw.getShift());
System.out.println("Payrate : " + pw.getPayRate());
}
}
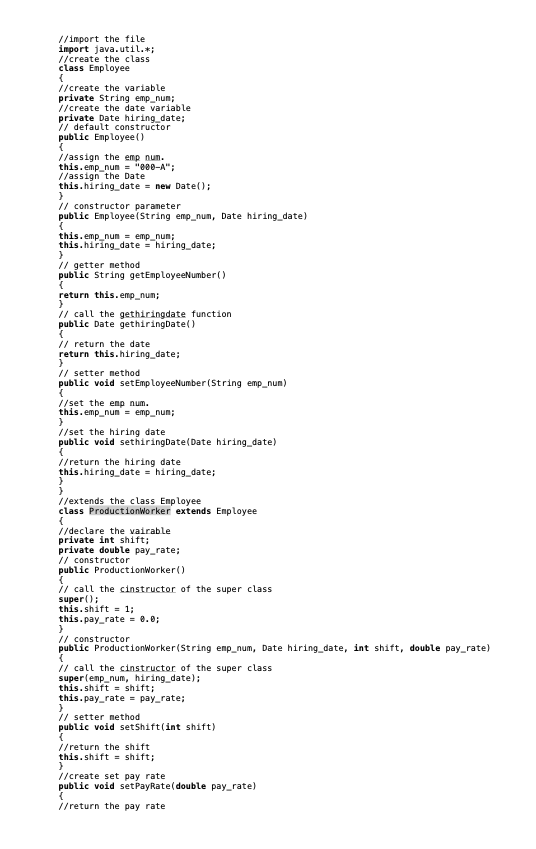
![this.pay_rate = pay_rate;
//. getter nethod
public int getShift()
return this.shift;
// getter nethod of get pay rate
public double getPayRate()
//return the pay rate
return this.pay_rate;
//create main method
public class Main
public static void nain(String(] args)
//create the object of the class
Productionworker pw = new Productionworker ("914-A", new Date(), 1, 20.36);
System.out.println ("Employee Number : " + pw.getEmployeeNumber ());
System.out.println("Hiring Date : " + pw.gethiringDate()):
System.out.println("Shift : " + pw.getShift());
System.out.println ("Payrate : " + pw.getPayRate());](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3e484d43-c7d4-4e91-b441-5ffb05728204%2Fb7915473-2b90-4f5f-8457-b8ed5cf54408%2Fl4z2erw_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

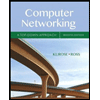
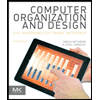
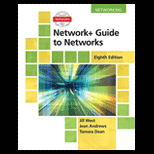
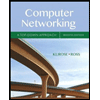
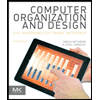
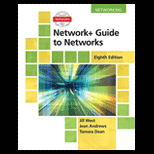
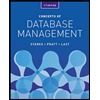
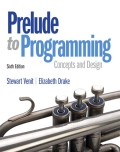
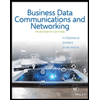