Tractors Modification of the Tractor project. Make a copy of your testTractor Package name it testLoader and make the following modifications and additions. Let us create a class hierarchy for tractors to use in a fleet management system. All of your classes should be in separate files within your project package. Name your package testLoader. Modify the base class named Tractor. The Tractor class will have a static attribute called Company. All tractors are owned by the same company. Create a class named Loader that inherits from Tractor. Loaders had a Bucket Size (1-5) the Bucket Size effects the RentalProfit by an additional $100-$500. You will need to Override the RentalProfit method to perform this calculation correctly. Create a fully parameterized constructor that uses a reference to super(). Override the toString to contain the base tractor information plus the Bucket Size option. Create a class called Harvester. Harvesters are must be rented for at least 20 days. Override the setter for Rental days to reflect this. Harvesters may have a tow behind trailer option (1-3) that adds $1000-$3000 to the RentalProfit() calculation. Create a fully parameterized constructor that uses a reference to super(). Override the toString to contain the base tractor information plus the trailer option. In your test drivers main() method create a Tractor, Loader and a Harvester. Using the zero parameter contructor. Then use the set methods to set all attributes. Also create a Tractor, Loader and Harvester using the parameterized contructor. Set the company name to your name. Use the toString() methods to display the information for each tractor. Be sure to display the rentalProfit() calculation in your test driver. Create a zip file for your package
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Tractors
Modification of the Tractor project. Make a copy of your testTractor Package name it
testLoader and make the following modifications and additions.
Let us create a class hierarchy for tractors to use in a fleet management system. All of your
classes should be in separate files within your project package. Name your package
testLoader.
Modify the base class named Tractor. The Tractor class will have a static attribute called
Company. All tractors are owned by the same company.
Create a class named Loader that inherits from Tractor. Loaders had a Bucket Size (1-5) the
Bucket Size effects the RentalProfit by an additional $100-$500. You will need to Override the
RentalProfit method to perform this calculation correctly. Create a fully parameterized
constructor that uses a reference to super(). Override the toString to contain the base tractor
information plus the Bucket Size option.
Create a class called Harvester. Harvesters are must be rented for at least 20 days. Override
the setter for Rental days to reflect this. Harvesters may have a tow behind trailer option (1-3)
that adds $1000-$3000 to the RentalProfit() calculation. Create a fully parameterized
constructor that uses a reference to super(). Override the toString to contain the base tractor
information plus the trailer option.
In your test drivers main() method create a Tractor, Loader and a Harvester. Using the zero
parameter contructor. Then use the set methods to set all attributes. Also create a Tractor,
Loader and Harvester using the parameterized contructor. Set the company name to your
name. Use the toString() methods to display the information for each tractor. Be sure to
display the rentalProfit() calculation in your test driver.
Create a zip file for your package
public class testTractor {
public static void main(String[] args) {
// Creates an object
Tractor t1=new Tractor();
// sets the parametrs using gettrs
t1.setID("A12V");
t1.setRentalDays(10);
t1.setRentalRate(345.5);
// prints the values
System.out.println(t1);
// creates ana object
Tractor t2 =new Tractor("3424DS",342.5,32);
System.out.println("Tractor VIN: " + t2.getID() + "\nRental Days: " + t2.getRentalDays() + "\nRental Rate: " + t2.getRentalRate()+"\n");
// creates another object
Tractor t3=new Tractor();
// invalid values
t3.setID("ACD234");
t3.setRentalDays(-5);
t3.setRentalRate(-456.5);
System.out.println(t3);
}
}
public class Tractor {
private String ID;
private double rentalRate;
private int rentalDays;
Tractor() {
this.ID = "00";
this.rentalDays = 0;
this.rentalRate = 0;
}
}
Tractor(String ID, double rentalRate, int rentalDays) {
this.setID(ID);
this.setRentalDays(rentalDays);
this.setRentalRate(rentalRate);
}
/**
* @return the iD
*/
public String getID() {
return ID;
}
/**
* @return the rentalDays
*/
public int getRentalDays() {
return rentalDays;
}
/**
* @return the rentalRate
*/
public double getRentalRate() {
return rentalRate;
}
/**
* @param iD the iD to set
*/
public void setID(String iD) {
this.ID = iD;
}
/**
* @param rentalDays the rentalDays to set
*/
public void setRentalDays(int rentalDays) {
if (rentalDays < 0) {
return;
}
this.rentalDays = rentalDays;
}
/**
* @param rentalRate the rentalRate to set
*/
public void setRentalRate(double rentalRate) {
if (rentalRate < 0) {
return;
}
this.rentalRate = rentalRate;
}
public double RentalProfit() {
return rentalRate * rentalDays;
}
@Override
public String toString() {
return "Tractor VIN: " + ID + "\nRental Days: " + rentalDays + "\nRental Rate: " + rentalRate+"\n";
}
}

Answer:
program:
· public class testTractor {
public static void main(String[] args) {
// Creates an object
Tractor t1=new Tractor();
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

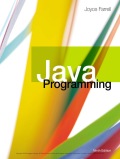
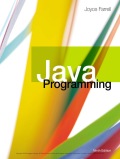