TRANSFORM THE C++ CODE BELOW INTO JAVA CODE. raw code:- #include using namespace std; using namespace std::chrono; //method to find countries between two cities using BFS traversal vector shortestpathusingbfs(map >&graph,string start,string end) { map >::iterator it; //creating a queue to store the cities queue q; q.push(start); //creating a map to store the visited cities map visited; visited[start]=true; //creating a vector to store the path map parent; parent[start]=""; //running a loop till the queue is empty while(!q.empty()) { //dequeing the city string s=q.front(); q.pop(); //checking if the city is the destination it=graph.find(s); if(it!=graph.end()) { //if the city is the destination vector::iterator it1; for(it1=it->second.begin();it1!=it->second.end();it1++) { //checking if the city is already visited if(!visited[*it1]) { visited[*it1]=true; q.push(*it1); parent[*it1]=s; } } } } //creating a vector to store the path vector path; string temp=end; //adding the destination city to the path while(temp!="") { //adding the city to the path path.push_back(temp); temp=parent[temp]; } //reversing the path reverse(path.begin(),path.end()); return path; } //method to find the shortest path between two cities using Dfs traversal vector shortestpathusingdfs(map> &graph, string start, string end, map &visited) { //creating a vector to store the path map>::iterator it; // vector path; //creating a stack to store the cities if (start == end) { path.push_back(start); return path; } visited[start] = true; it = graph.find(start); //checking if the city is present in the graph if (it != graph.end()) { //creating a vector to store the neighbours of the city vector::iterator it1; //running a loop till the vector is empty for (it1 = it->second.begin(); it1 != it->second.end(); it1++) { //checking if the city is already visited if (!visited[*it1]) { //if the city is not visited vector temp = shortestpathusingdfs(graph, *it1, end, visited); if (temp.size() > 0) { //adding the city to the path temp.push_back(start); if (path.size() == 0 || temp.size() < path.size()) { path = temp; } } } } } //returning the path return path; } int main() { //creating a map to store the graph fstream file; file.open("city.txt",ios::in); string s; //creating a map to store the graph map> m; //running a loop till the file is empty while(getline(file,s)) { stringstream ss(s); string city1,city2; ss>>city1>>city2; m[city1].push_back(city2); } file.close(); //creating a map to store the visited cities cout << "Path : " << endl; cout << "DFS: "; map visited; time_point start, end, start1, end1; start = system_clock::now(); //calling the method to find the shortest path using DFS traversal vector ans2 = shortestpathusingdfs(m, "Macau", "USA", visited); end = system_clock::now(); duration elapsed_seconds = end - start; for (int i = ans2.size()-1; i >=0; i--) { cout< ans1 = shortestpathusingbfs(m, "Macau", "USA"); end1 = system_clock::now(); duration elapsed_seconds1 = end1 - start1; for (int i = 0; i < ans1.size(); i++) { cout << ans1[i] << " "; } cout << endl; cout << "Time: "; cout << "DFS=" << elapsed_seconds.count() << " " << endl; cout<<"BFS="<
TRANSFORM THE C++ CODE BELOW INTO JAVA CODE. raw code:- #include using namespace std; using namespace std::chrono; //method to find countries between two cities using BFS traversal vector shortestpathusingbfs(map >&graph,string start,string end) { map >::iterator it; //creating a queue to store the cities queue q; q.push(start); //creating a map to store the visited cities map visited; visited[start]=true; //creating a vector to store the path map parent; parent[start]=""; //running a loop till the queue is empty while(!q.empty()) { //dequeing the city string s=q.front(); q.pop(); //checking if the city is the destination it=graph.find(s); if(it!=graph.end()) { //if the city is the destination vector::iterator it1; for(it1=it->second.begin();it1!=it->second.end();it1++) { //checking if the city is already visited if(!visited[*it1]) { visited[*it1]=true; q.push(*it1); parent[*it1]=s; } } } } //creating a vector to store the path vector path; string temp=end; //adding the destination city to the path while(temp!="") { //adding the city to the path path.push_back(temp); temp=parent[temp]; } //reversing the path reverse(path.begin(),path.end()); return path; } //method to find the shortest path between two cities using Dfs traversal vector shortestpathusingdfs(map> &graph, string start, string end, map &visited) { //creating a vector to store the path map>::iterator it; // vector path; //creating a stack to store the cities if (start == end) { path.push_back(start); return path; } visited[start] = true; it = graph.find(start); //checking if the city is present in the graph if (it != graph.end()) { //creating a vector to store the neighbours of the city vector::iterator it1; //running a loop till the vector is empty for (it1 = it->second.begin(); it1 != it->second.end(); it1++) { //checking if the city is already visited if (!visited[*it1]) { //if the city is not visited vector temp = shortestpathusingdfs(graph, *it1, end, visited); if (temp.size() > 0) { //adding the city to the path temp.push_back(start); if (path.size() == 0 || temp.size() < path.size()) { path = temp; } } } } } //returning the path return path; } int main() { //creating a map to store the graph fstream file; file.open("city.txt",ios::in); string s; //creating a map to store the graph map> m; //running a loop till the file is empty while(getline(file,s)) { stringstream ss(s); string city1,city2; ss>>city1>>city2; m[city1].push_back(city2); } file.close(); //creating a map to store the visited cities cout << "Path : " << endl; cout << "DFS: "; map visited; time_point start, end, start1, end1; start = system_clock::now(); //calling the method to find the shortest path using DFS traversal vector ans2 = shortestpathusingdfs(m, "Macau", "USA", visited); end = system_clock::now(); duration elapsed_seconds = end - start; for (int i = ans2.size()-1; i >=0; i--) { cout< ans1 = shortestpathusingbfs(m, "Macau", "USA"); end1 = system_clock::now(); duration elapsed_seconds1 = end1 - start1; for (int i = 0; i < ans1.size(); i++) { cout << ans1[i] << " "; } cout << endl; cout << "Time: "; cout << "DFS=" << elapsed_seconds.count() << " " << endl; cout<<"BFS="<
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
TRANSFORM THE C++ CODE BELOW INTO JAVA CODE.
raw code:-
#include<bits/stdc++.h> using namespace std; using namespace std::chrono; //method to find countries between two cities using BFS traversalExpert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
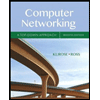
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
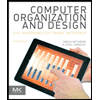
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
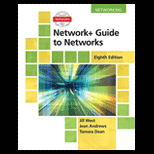
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
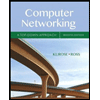
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
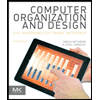
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
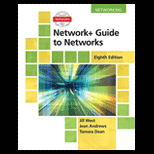
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
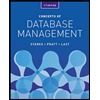
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
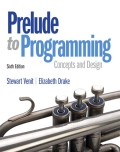
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
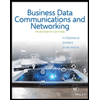
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY