Tripleton ( We call the design pattern "single"-ton because there can only be a single instance of the object. This can be extrapolated to two, three, four, etc., instances as well. In this case, we'll build a tripleton (maximum of three instances). Building a tripleton is exactly the same as a singleton. You need to make the constructor private, and add a public static method called `getInstance to return an instance. In this case, the method will create new instances while it can, and once it has created all three of them, it will return the 3rd instance. This Tripleton class should also have a method called `getInstanceN` to retrieve the Nth instance. Put this Tripleton in a package called tripleton and create some tests in a main method there. Run a test to show that when you create a 4th instance, it is equal to the 3rd instance. University In this part of the lab, we'll implement the observer pattern. In this case, we'll be using a couple deprecated packages in Java (do not use these for real applications, use java.beans) as they make it simpler to understand the pattern. Create a Course class that has two instance variables: a course name, and a Prof object. Add getters for those variables. Next, create the Prof class which extends java.util.Observable and is initialized with a name. It should have a name, room number, a date for the midterm, and a collecton of Students. The Student should implement the Observer interface and is initialized with a name. It should have a name, room number, and a course variable. Add a Secretary class that also implements the Observer interface. It should only have a date as an instance variable that will get set in it's update method later. In the Prof class, create a setter for the midterm date that also sets it's status to changed and notifies its observers with the Date like so: public void setMidterm (Date date) { midterm = date; // see why it is useful to have getters and setters! // we can now notify observers of the change setChanged(); notifyObservers ( date ); Build a similar method for the room number.
Tripleton ( We call the design pattern "single"-ton because there can only be a single instance of the object. This can be extrapolated to two, three, four, etc., instances as well. In this case, we'll build a tripleton (maximum of three instances). Building a tripleton is exactly the same as a singleton. You need to make the constructor private, and add a public static method called `getInstance to return an instance. In this case, the method will create new instances while it can, and once it has created all three of them, it will return the 3rd instance. This Tripleton class should also have a method called `getInstanceN` to retrieve the Nth instance. Put this Tripleton in a package called tripleton and create some tests in a main method there. Run a test to show that when you create a 4th instance, it is equal to the 3rd instance. University In this part of the lab, we'll implement the observer pattern. In this case, we'll be using a couple deprecated packages in Java (do not use these for real applications, use java.beans) as they make it simpler to understand the pattern. Create a Course class that has two instance variables: a course name, and a Prof object. Add getters for those variables. Next, create the Prof class which extends java.util.Observable and is initialized with a name. It should have a name, room number, a date for the midterm, and a collecton of Students. The Student should implement the Observer interface and is initialized with a name. It should have a name, room number, and a course variable. Add a Secretary class that also implements the Observer interface. It should only have a date as an instance variable that will get set in it's update method later. In the Prof class, create a setter for the midterm date that also sets it's status to changed and notifies its observers with the Date like so: public void setMidterm (Date date) { midterm = date; // see why it is useful to have getters and setters! // we can now notify observers of the change setChanged(); notifyObservers ( date ); Build a similar method for the room number.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I really need help on this please . in Java
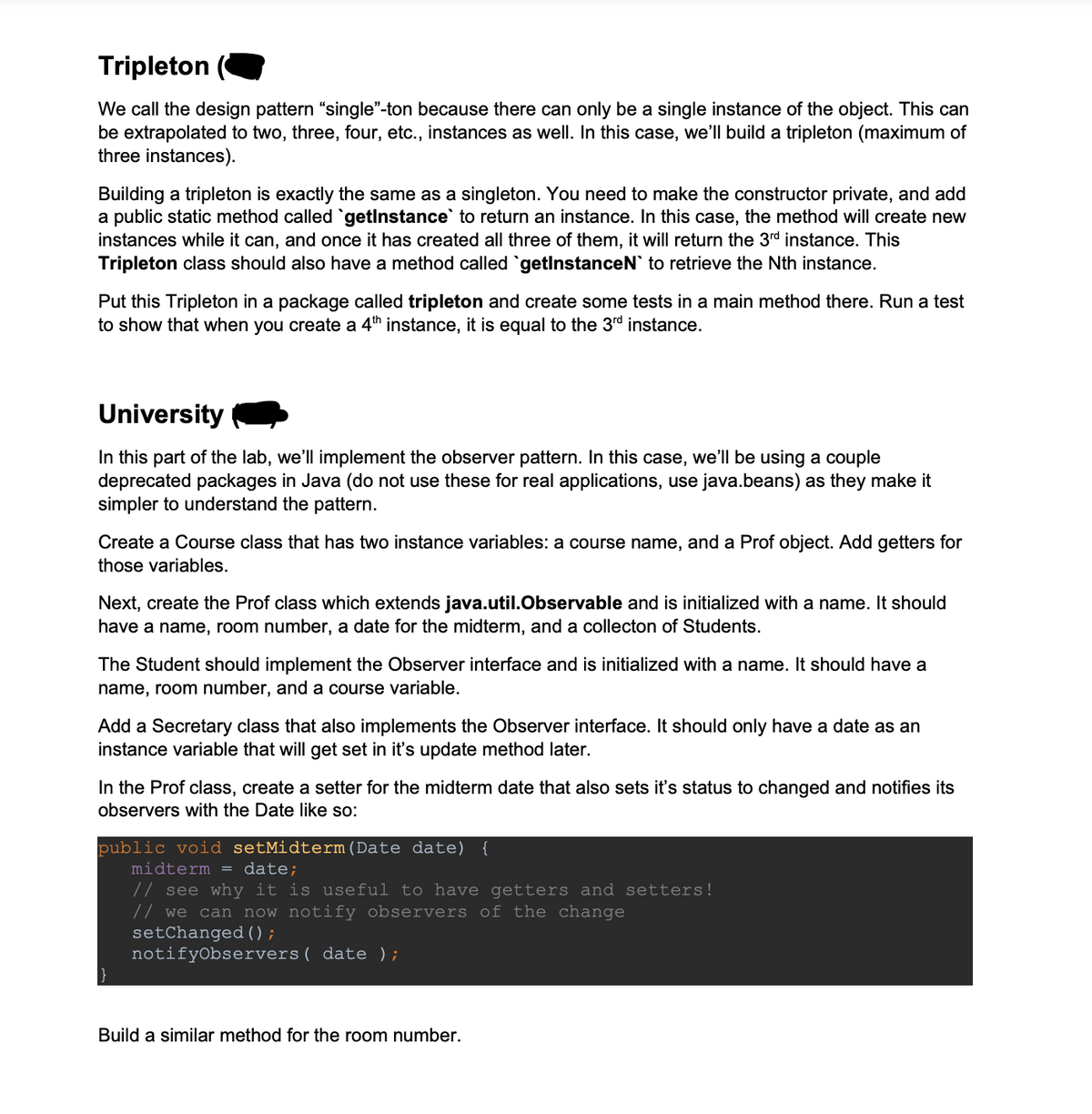
Transcribed Image Text:Tripleton (
We call the design pattern "single"-ton because there can only be a single instance of the object. This can
be extrapolated to two, three, four, etc., instances as well. In this case, we'll build a tripleton (maximum of
three instances).
Building a tripleton is exactly the same as a singleton. You need to make the constructor private, and add
a public static method called `getInstance to return an instance. In this case, the method will create new
instances while it can, and once it has created all three of them, it will return the 3rd instance. This
Tripleton class should also have a method called `getInstanceN` to retrieve the Nth instance.
Put this Tripleton in a package called tripleton and create some tests in a main method there. Run a test
to show that when you create a 4th instance, it is equal to the 3rd instance.
University
In this part of the lab, we'll implement the observer pattern. In this case, we'll be using a couple
deprecated packages in Java (do not use these for real applications, use java.beans) as they make it
simpler to understand the pattern.
Create a Course class that has two instance variables: a course name, and a Prof object. Add getters for
those variables.
Next, create the Prof class which extends java.util.Observable and is initialized with a name. It should
have a name, room number, a date for the midterm, and a collecton of Students.
The Student should implement the Observer interface and is initialized with a name. It should have a
name, room number, and a course variable.
Add a Secretary class that also implements the Observer interface. It should only have a date as an
instance variable that will get set in it's update method later.
In the Prof class, create a setter for the midterm date that also sets it's status to changed and notifies its
observers with the Date like so:
public void setMidterm (Date date) {
midterm = date;
// see why it is useful to have getters and setters!
// we can now notify observers of the change
setChanged();
notifyObservers ( date );
Build a similar method for the room number.
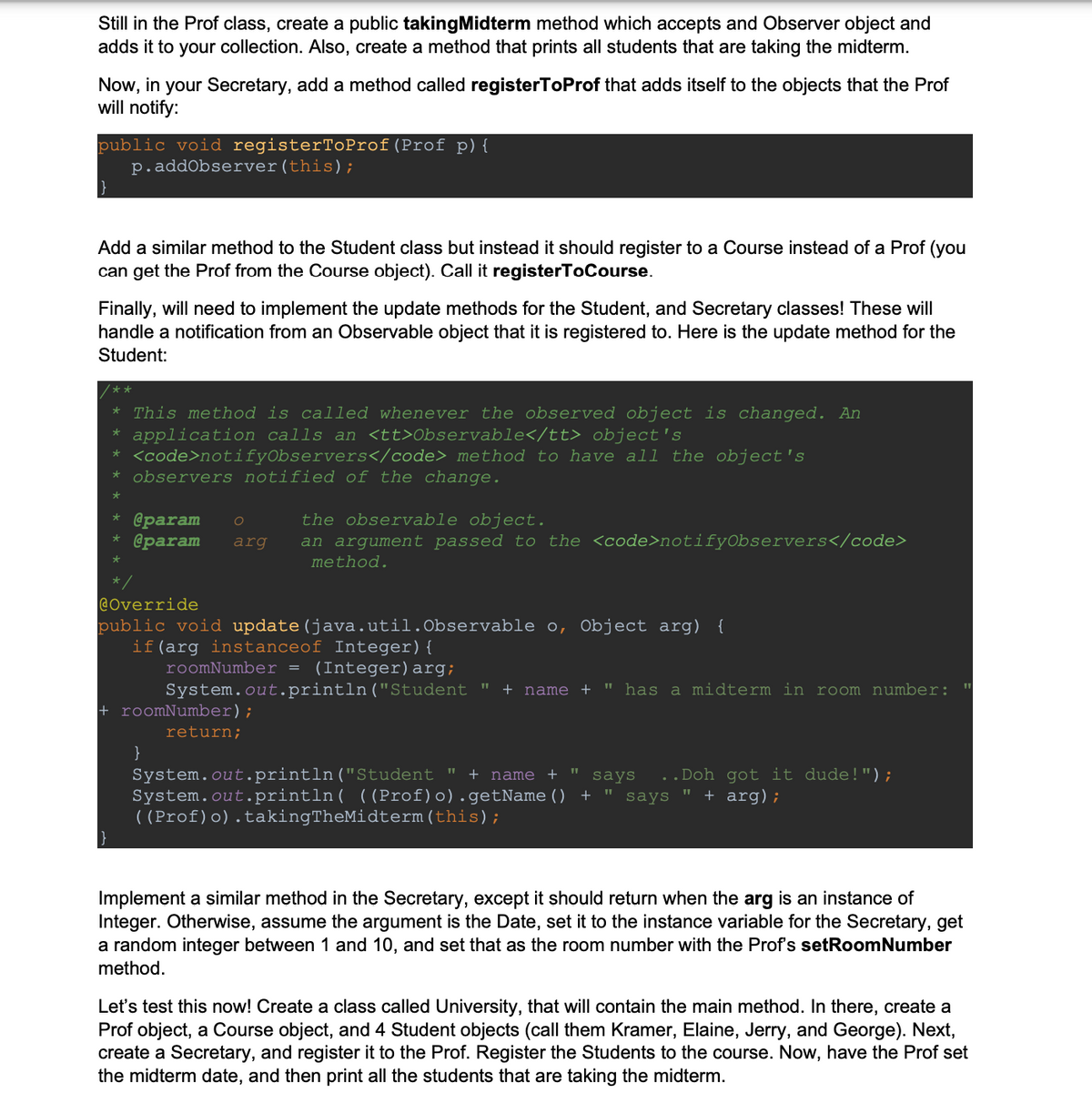
Transcribed Image Text:Still in the Prof class, create a public taking Midterm method which accepts and Observer object and
adds it to your collection. Also, create a method that prints all students that are taking the midterm.
Now, in your Secretary, add a method called registerToProf that adds itself to the objects that the Prof
will notify:
public void registerToProf (Prof p) {
p.addObserver(this);
Add a similar method to the Student class but instead it should register to a Course instead of a Prof (you
can get the Prof from the Course object). Call it registerToCourse.
Finally, will need to implement the update methods for the Student, and Secretary classes! These will
handle a notification from an Observable object that it is registered to. Here is the update method for the
Student:
**
This method is called whenever the observed object is changed. An
* application calls an <tt>Observable</tt> object's
*
<code>notifyObservers</code> method to have all the object's
* observers notified of the change.
*
*
*
@param О
@param
arg
the observable object.
an argument passed to the <code>notifyObservers</code>
method.
@Override
public void update (java.util. Observable o, Object arg) {
if (arg instanceof Integer) {
roomNumber = (Integer) arg;
System.out.println("Student " + name + " has a midterm in room number:
+ roomNumber);
return;
}
System.out.println("Student " + name +
says ..Doh got it dude!");
"
System.out.println( ((Prof)o).getName() + " says + arg);
((Prof)o).takingTheMidterm (this);
Implement a similar method in the Secretary, except it should return when the arg is an instance of
Integer. Otherwise, assume the argument is the Date, set it to the instance variable for the Secretary, get
a random integer between 1 and 10, and set that as the room number with the Prof's setRoomNumber
method.
Let's test this now! Create a class called University, that will contain the main method. In there, create a
Prof object, a Course object, and 4 Student objects (call them Kramer, Elaine, Jerry, and George). Next,
create a Secretary, and register it to the Prof. Register the Students to the course. Now, have the Prof set
the midterm date, and then print all the students that are taking the midterm.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 8 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
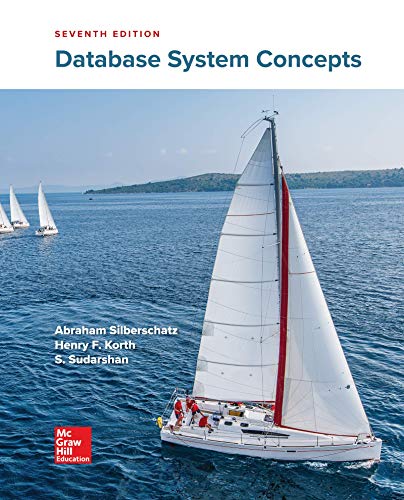
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
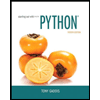
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
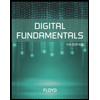
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
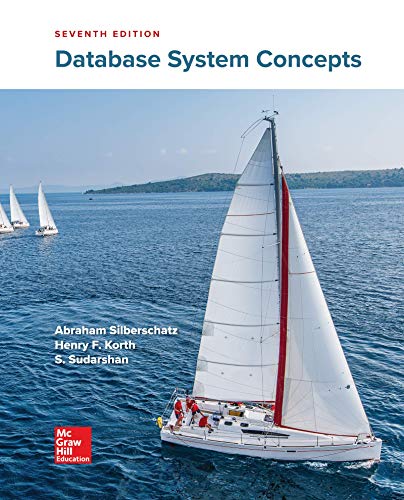
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
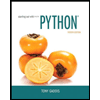
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
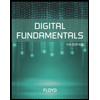
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
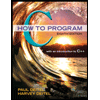
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
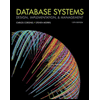
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
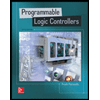
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education