urrent_year = 2021 class Employee: # constructor def __init__(self) -> None: self.employee_no = 0 self.name = "" self.status = "" self.position = "" self.basic_salary = 0 self.year_end_bonus = 0 self.year_hired = 0 # function to get employee date from user def get_data(self): self.employee_no = int(input("Enter employee number: ")) self.name = input("Enter employee name: ") self.status = input("Enter employee name(R/P/C): ") self.position = input("Enter employee position title: ") self.year_hired = int(input("Enter year hired: ")) # function to calculate gross salary based on employee information # and criteria as given in question def calculate_gross_salary(self): rate_per_hour = 0.0 years_of_service = current_year - self.year_hired # rate per hour case 1 if self.status == "C" and years_of_service == 1: rate_per_hour = float(input("Please enter the rate per hour(500-950): ")) while rate_per_hour not in range(500, 951): print("Invalid rate per hour") rate_per_hour = float(input("Please enter the rate per hour(500-950): ")) # rate per hour case 2 elif self.status == "P" and years_of_service in range(2, 4): rate_per_hour = float(input("Please enter the rate per hour(1000-1500): ")) while rate_per_hour not in range(1000, 1501): print("Invalid rate per hour") rate_per_hour = float(input("Please enter the rate per hour(1000-1500): ")) # rate per hour case 3 elif self.status == "C" and years_of_service in range(4, 11): rate_per_hour = float(input("Please enter the rate per hour(1950-2500): ")) while rate_per_hour not in range(1950-2500): print("Invalid rate per hour") rate_per_hour = float(input("Please enter the rate per hour(1950-2500): ")) # rate per hour case 4 elif self.status == "C" and years_of_service > 10: rate_per_hour = float(input("Please enter the rate per hour(3000-5000): ")) while rate_per_hour not in range(3000-5000): print("Invalid rate per hour") rate_per_hour = float(input("Please enter the rate per hour(3000-5000): ")) # calculate the basic salary self.basic_salary = rate_per_hour * 160 # function to calculate the annual salary def calculate_annual_salary(self): self.calculate_gross_salary() return self.basic_salary * 12 # function to calculate the year end bonus # according to criteria as given in question def calculate_year_end_bonus(self): bonus = 0.0 years_of_service = current_year - self.year_hired # case 1 if self.status == "C" and years_of_service == 1: bonus = .1 # case 2 elif self.status == "P" and years_of_service in range(2, 4): bonus = .2 # case 3 elif self.status == "C" and years_of_service in range(4, 11): bonus = .5 # case 4 elif self.status == "C" and years_of_service > 10: bonus = .75 # calculate year end bonus self.year_end_bonus = self.calculate_annual_salary() * bonus # function to display employee data def display(self): self.calculate_year_end_bonus() print("Employee number:", self.employee_no) print("Employee name:", self.name) print("Employee status:", self.status) print("Employee position:", self.position) print("Employee year hired:", self.year_hired) print("Employee basic salary:", self.basic_salary) print("Employee year end bonus:", self.year_end_bonus) # create employee object emp = Employee() # get data from user emp.get_data() # show the employee data emp.display() help me design an output that is similar to a Payslip Form for output validation. Kindly generate three final output based on the employees’ status. (this is only a follow-up
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
current_year = 2021
class Employee:
# constructor
def __init__(self) -> None:
self.employee_no = 0
self.name = ""
self.status = ""
self.position = ""
self.basic_salary = 0
self.year_end_bonus = 0
self.year_hired = 0
# function to get employee date from user
def get_data(self):
self.employee_no = int(input("Enter employee number: "))
self.name = input("Enter employee name: ")
self.status = input("Enter employee name(R/P/C): ")
self.position = input("Enter employee position title: ")
self.year_hired = int(input("Enter year hired: "))
# function to calculate gross salary based on employee information
# and criteria as given in question
def calculate_gross_salary(self):
rate_per_hour = 0.0
years_of_service = current_year - self.year_hired
# rate per hour case 1
if self.status == "C" and years_of_service == 1:
rate_per_hour = float(input("Please enter the rate per hour(500-950): "))
while rate_per_hour not in range(500, 951):
print("Invalid rate per hour")
rate_per_hour = float(input("Please enter the rate per hour(500-950): "))
# rate per hour case 2
elif self.status == "P" and years_of_service in range(2, 4):
rate_per_hour = float(input("Please enter the rate per hour(1000-1500): "))
while rate_per_hour not in range(1000, 1501):
print("Invalid rate per hour")
rate_per_hour = float(input("Please enter the rate per hour(1000-1500): "))
# rate per hour case 3
elif self.status == "C" and years_of_service in range(4, 11):
rate_per_hour = float(input("Please enter the rate per hour(1950-2500): "))
while rate_per_hour not in range(1950-2500):
print("Invalid rate per hour")
rate_per_hour = float(input("Please enter the rate per hour(1950-2500): "))
# rate per hour case 4
elif self.status == "C" and years_of_service > 10:
rate_per_hour = float(input("Please enter the rate per hour(3000-5000): "))
while rate_per_hour not in range(3000-5000):
print("Invalid rate per hour")
rate_per_hour = float(input("Please enter the rate per hour(3000-5000): "))
# calculate the basic salary
self.basic_salary = rate_per_hour * 160
# function to calculate the annual salary
def calculate_annual_salary(self):
self.calculate_gross_salary()
return self.basic_salary * 12
# function to calculate the year end bonus
# according to criteria as given in question
def calculate_year_end_bonus(self):
bonus = 0.0
years_of_service = current_year - self.year_hired
# case 1
if self.status == "C" and years_of_service == 1:
bonus = .1
# case 2
elif self.status == "P" and years_of_service in range(2, 4):
bonus = .2
# case 3
elif self.status == "C" and years_of_service in range(4, 11):
bonus = .5
# case 4
elif self.status == "C" and years_of_service > 10:
bonus = .75
# calculate year end bonus
self.year_end_bonus = self.calculate_annual_salary() * bonus
# function to display employee data
def display(self):
self.calculate_year_end_bonus()
print("Employee number:", self.employee_no)
print("Employee name:", self.name)
print("Employee status:", self.status)
print("Employee position:", self.position)
print("Employee year hired:", self.year_hired)
print("Employee basic salary:", self.basic_salary)
print("Employee year end bonus:", self.year_end_bonus)
# create employee object
emp = Employee()
# get data from user
emp.get_data()
# show the employee data
emp.display()
help me design an output that is similar to a Payslip Form for output validation.
Kindly generate three final output based on the employees’ status.
(this is only a follow-up)

Step by step
Solved in 3 steps with 1 images

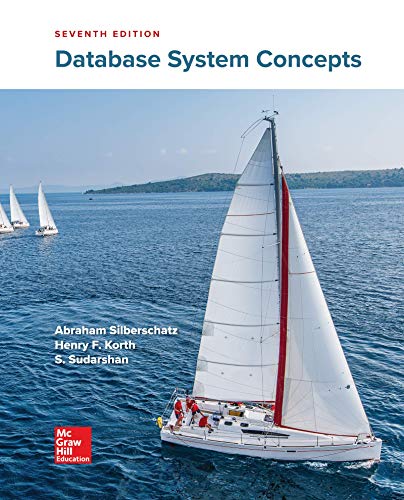
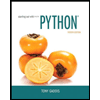
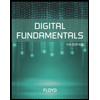
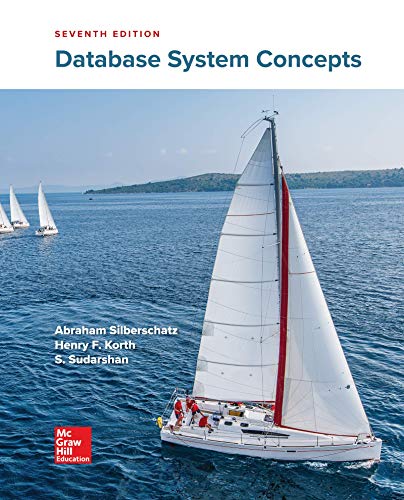
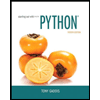
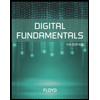
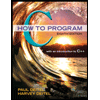
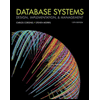
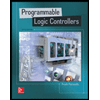