Use a client code to test the course ADT: 1. Declare 2 course instances courseOne, and course Two ("CS 115", 01, 3, "Monday, Wednesday", "04:30 - 05:20", "CL", 126, 8, "Dr. X"). 8 is the maximum course enrolment. 2. Set the values of courseOne to ("CS 210", 02, 3, "Tuesday, Thursday", "10:30 - 11:20", "CL", 128, "Dr. Y"). The maximum course enrollment, which is 5, remains unchanged. 3. Display the values of courseOne and courseTwo. Also, use displayStudentList function to display IDs of students in each course. 4. Write a non-member function named courseCompare that compares two course instances to check if any of the corresponding fields (excluding studentsId) are the same and displays the result of the comparisons. For example, it could print "different course id number", ... "same number of credits", and so on. 5. Prompt user to enter a unique student id and then enroll it in courseOne 6. Use a loop to repeat step 5, 4 more times. 7. Prompt user to enter a unique student id and then enroll it in course Two Use a loop to repeat step 7, 7 more times. 8. 9. Use displayStudentList function to display IDs of students in each course. 10. Display the course status of both instances. 11. Prompt user to enter another unique student id and then try to enroll it in courseOne. If not possible, an error must be displayed indicating the course status. 12. Prompt user to enter another unique student id and then try to enroll it in courseTwo. If not possible, an error must be displayed indicating the course status. Note: in addition to the specifications above, you may also need to add other data fields and (member) functions.
Use a client code to test the course ADT: 1. Declare 2 course instances courseOne, and course Two ("CS 115", 01, 3, "Monday, Wednesday", "04:30 - 05:20", "CL", 126, 8, "Dr. X"). 8 is the maximum course enrolment. 2. Set the values of courseOne to ("CS 210", 02, 3, "Tuesday, Thursday", "10:30 - 11:20", "CL", 128, "Dr. Y"). The maximum course enrollment, which is 5, remains unchanged. 3. Display the values of courseOne and courseTwo. Also, use displayStudentList function to display IDs of students in each course. 4. Write a non-member function named courseCompare that compares two course instances to check if any of the corresponding fields (excluding studentsId) are the same and displays the result of the comparisons. For example, it could print "different course id number", ... "same number of credits", and so on. 5. Prompt user to enter a unique student id and then enroll it in courseOne 6. Use a loop to repeat step 5, 4 more times. 7. Prompt user to enter a unique student id and then enroll it in course Two Use a loop to repeat step 7, 7 more times. 8. 9. Use displayStudentList function to display IDs of students in each course. 10. Display the course status of both instances. 11. Prompt user to enter another unique student id and then try to enroll it in courseOne. If not possible, an error must be displayed indicating the course status. 12. Prompt user to enter another unique student id and then try to enroll it in courseTwo. If not possible, an error must be displayed indicating the course status. Note: in addition to the specifications above, you may also need to add other data fields and (member) functions.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please try using Occam’s razor principle if possible.
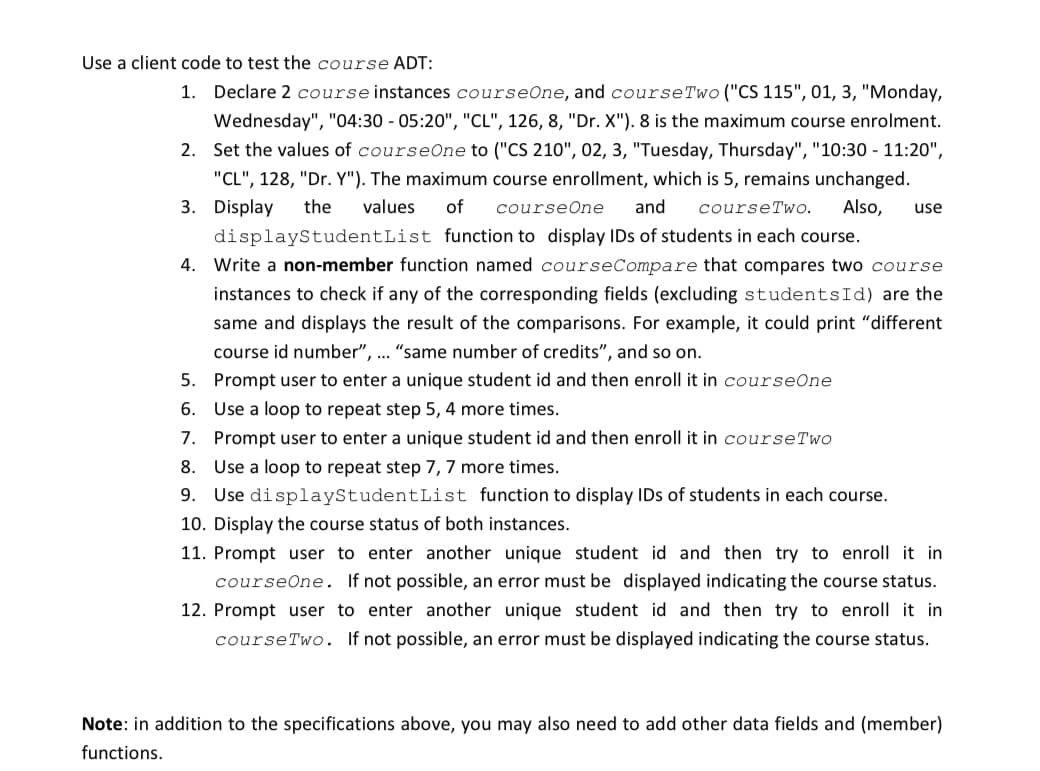
Transcribed Image Text:Use a client code to test the course ADT:
1. Declare 2 course instances courseOne, and course Two ("CS 115", 01, 3, "Monday,
Wednesday", "04:30 - 05:20", "CL", 126, 8, "Dr. X"). 8 is the maximum course enrolment.
2. Set the values of courseOne to ("CS 210", 02, 3, "Tuesday, Thursday", "10:30 - 11:20",
"CL", 128, "Dr. Y"). The maximum course enrollment, which is 5, remains unchanged.
3. Display the values of courseOne and courseTwo. Also, use
displayStudentList function to display IDs of students in each course.
4. Write a non-member function named courseCompare that compares two course
instances to check if any of the corresponding fields (excluding studentsId) are the
same and displays the result of the comparisons. For example, it could print "different
course id number", ... "same number of credits", and so on.
5. Prompt user to enter a unique student id and then enroll it in courseOne
6.
Use a loop to repeat step 5, 4 more times.
7. Prompt user to enter a unique student id and then enroll it in course Two
8. Use a loop to repeat step 7, 7 more times.
9. Use displayStudentList function to display IDs of students in each course.
10. Display the course status of both instances.
11. Prompt user to enter another unique student id and then try to enroll it in
courseOne. If not possible, an error must be displayed indicating the course status.
12. Prompt user to enter another unique student id and then try to enroll it in
course Two. If not possible, an error must be displayed indicating the course status.
Note: in addition to the specifications above, you may also need to add other data fields and (member)
functions.
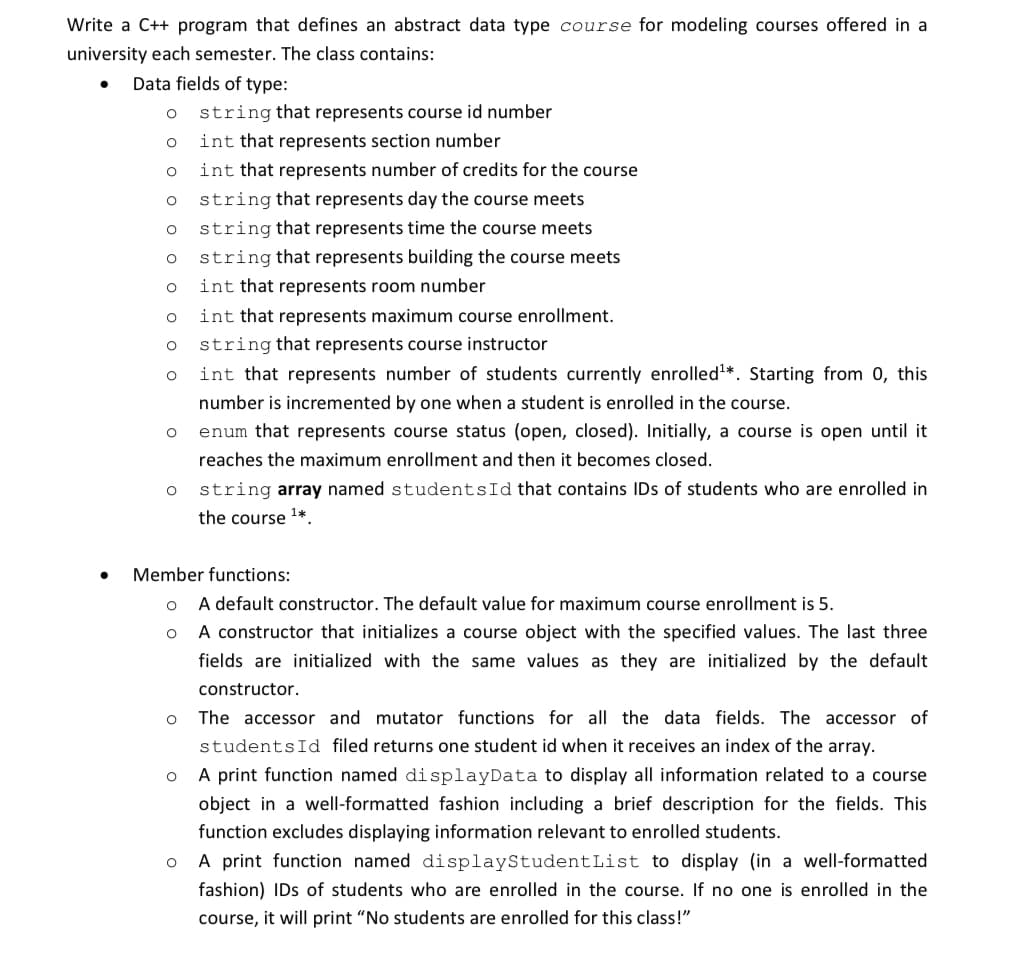
Transcribed Image Text:Write a C++ program that defines an abstract data type course for modeling courses offered in a
university each semester. The class contains:
Data fields of type:
O
O
O
O
O
O
O
O
O
O
O
O
O
O
string that represents course id number
int that represents section number
int that represents number of credits for the course
string that represents day the course meets
string that represents time the course meets.
string that represents building the course meets
int that represents room number
int that represents maximum course enrollment.
Member functions:
O
string that represents course instructor
int that represents number of students currently enrolled¹*. Starting from 0, this
number is incremented by one when a student is enrolled in the course.
enum that represents course status (open, closed). Initially, a course is open until it
reaches the maximum enrollment and then it becomes closed.
string array named students Id that contains IDs of students who are enrolled in
the course ¹*
A default constructor. The default value for maximum course enrollment is 5.
A constructor that initializes a course object with the specified values. The last three
fields are initialized with the same values as they are initialized by the default
constructor.
The accessor and mutator functions for all the data fields. The accessor of
students Id filed returns one student id when it receives an index of the array.
O
A print function named displayData to display all information related to a course
object in a well-formatted fashion including a brief description for the fields. This
function excludes displaying information relevant to enrolled students.
O
A print function named displayStudent List to display (in a well-formatted
fashion) IDs of students who are enrolled in the course. If no one is enrolled in the
course, it will print "No students are enrolled for this class!"
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
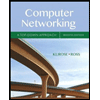
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
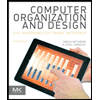
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
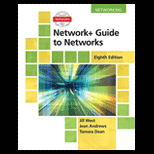
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
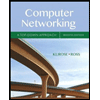
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
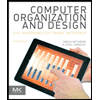
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
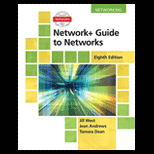
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
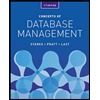
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
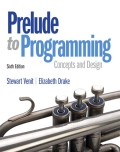
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
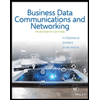
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY