Use C++ to read data from a text file and create an array of numbers. Use the C++ 'fstream' library functions to open, read and close a text file. You can use the C++ 'iomanip' library to format your output and manage whitespace on your input. • Graduate students must create and write the data to a new text file. 2 Your assignment is to create a program that does the following: a. You will need to create a new file (you can do this in the file system – make it plain text) and call it mydata.txt. b. Put a column of numbers in the text file to read later. Pay attention to type – make them all have decimal points. In repl.it, use the numbers in my file. c. Based on the examples in the slides (L3) and the textbook, write a C++ program, include 'iostream' and 'fstream'. You might need 'iomanip'. d. Your program should read the numbers from the file into an array and print the array to the console in reverse order using a for loop. e. Graduate Students must also print the output to a new text file called output.txt. f. Follow Professional Standards: For details on how programming style, see the Programming Style Guide. Put your name in your program block comment at the top. Use logical variable names and function names – do not make me guess. Indent properly. Greet the user with the name of the program, and your name. Indicate that the program ended normally by saying Bye or thanking the user for using your program. Do not forget the block comments for each function. Use in-line comments as required. g. Optional Challenge for undergrads (required for Graduate Students): i. Print the output to a new text file called output.txt for an extra point.
Use C++ to read data from a text file and create an array of numbers. Use the C++ 'fstream' library functions to open, read and close a text file. You can use the C++ 'iomanip' library to format your output and manage whitespace on your input. • Graduate students must create and write the data to a new text file. 2 Your assignment is to create a program that does the following: a. You will need to create a new file (you can do this in the file system – make it plain text) and call it mydata.txt. b. Put a column of numbers in the text file to read later. Pay attention to type – make them all have decimal points. In repl.it, use the numbers in my file. c. Based on the examples in the slides (L3) and the textbook, write a C++ program, include 'iostream' and 'fstream'. You might need 'iomanip'. d. Your program should read the numbers from the file into an array and print the array to the console in reverse order using a for loop. e. Graduate Students must also print the output to a new text file called output.txt. f. Follow Professional Standards: For details on how programming style, see the Programming Style Guide. Put your name in your program block comment at the top. Use logical variable names and function names – do not make me guess. Indent properly. Greet the user with the name of the program, and your name. Indicate that the program ended normally by saying Bye or thanking the user for using your program. Do not forget the block comments for each function. Use in-line comments as required. g. Optional Challenge for undergrads (required for Graduate Students): i. Print the output to a new text file called output.txt for an extra point.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
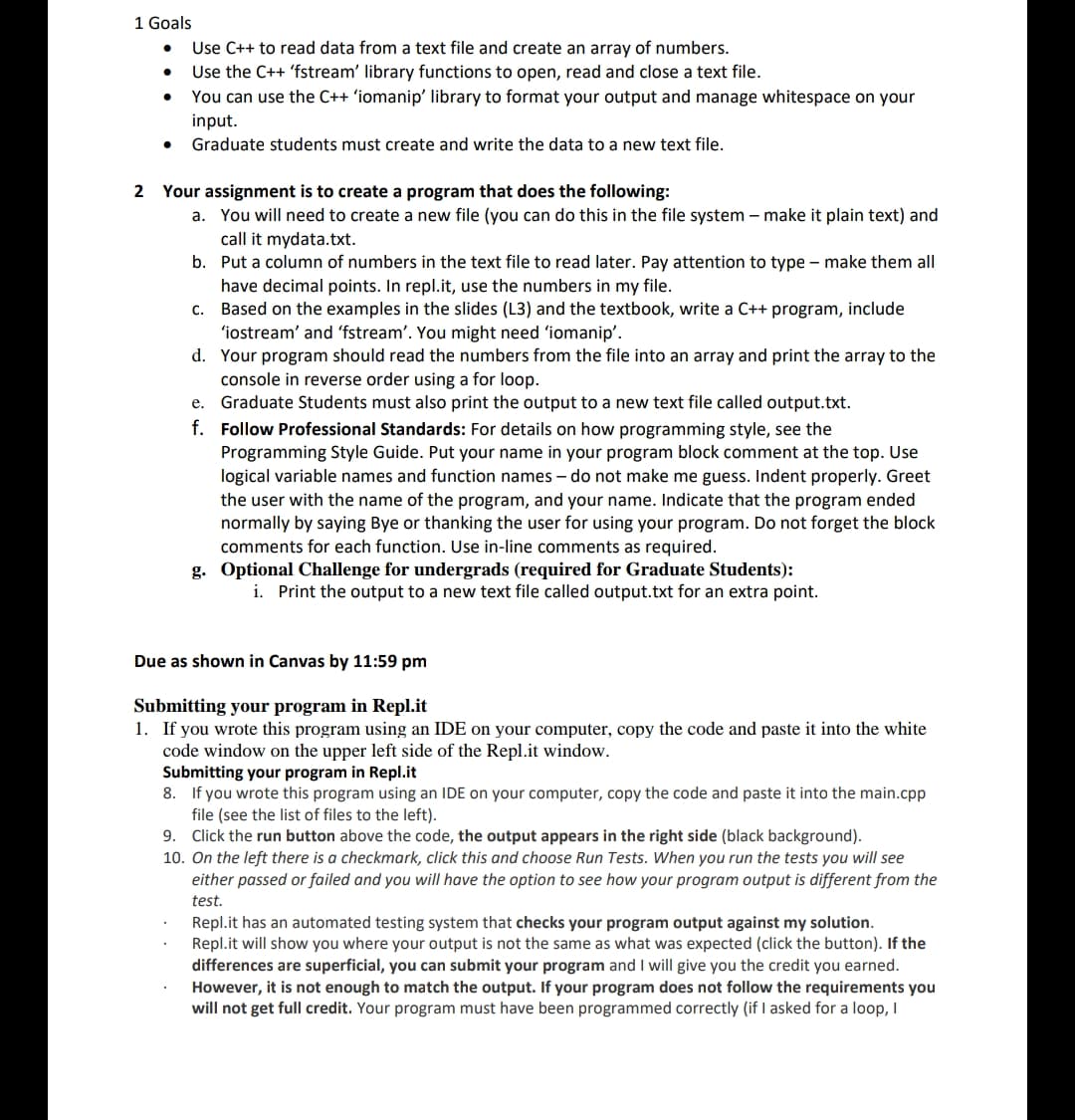
Transcribed Image Text:1 Goals
Use C++ to read data from a text file and create an array of numbers.
Use the C++ 'fstream' library functions to open, read and close a text file.
You can use the C++ 'iomanip' library to format your output and manage whitespace on your
input.
Graduate students must create and write the data to a new text file.
2 Your assignment is to create a program that does the following:
a. You will need to create a new file (you can do this in the file system – make it plain text) and
call it mydata.txt.
b. Put a column of numbers in the text file to read later. Pay attention to type – make them all
have decimal points. In repl.it, use the numbers in my file.
c. Based on the examples in the slides (L3) and the textbook, write a C++ program, include
'iostream' and 'fstream'. You might need 'iomanip'.
d. Your program should read the numbers from the file into an array and print the array to the
console in reverse order using a for loop.
e. Graduate Students must also print the output to a new text file called output.txt.
f. Follow Professional Standards: For details on how programming style, see the
Programming Style Guide. Put your name in your program block comment at the top. Use
logical variable names and function names – do not make me guess. Indent properly. Greet
the user with the name of the program, and your name. Indicate that the program ended
normally by saying Bye or thanking the user for using your program. Do not forget the block
comments for each function. Use in-line comments as required.
g. Optional Challenge for undergrads (required for Graduate Students):
i. Print the output to a new text file called output.txt for an extra point.
Due as shown in Canvas by 11:59 pm
Submitting your program in Repl.it
1. If you wrote this program using an IDE on your computer, copy the code and paste it into the white
code window on the upper left side of the Repl.it window.
Submitting your program in Repl.it
8. If you wrote this program using an IDE on your computer, copy the code and paste it into the main.cpp
file (see the list of files to the left).
9. Click the run button above the code, the output appears in the right side (black background).
10. On the left there is a checkmark, click this and choose Run Tests. When you run the tests you will see
either passed or failed and you will have the option to see how your program output is different from the
test.
Repl.it has an automated testing system that checks your program output against my solution.
Repl.it will show you where your output is not the same as what was expected (click the button). If the
differences are superficial, you can submit your program and I will give you the credit you earned.
However, it is not enough to match the output. If your program does not follow the requirements you
will not get full credit. Your program must have been programmed correctly (if I asked for a loop, I
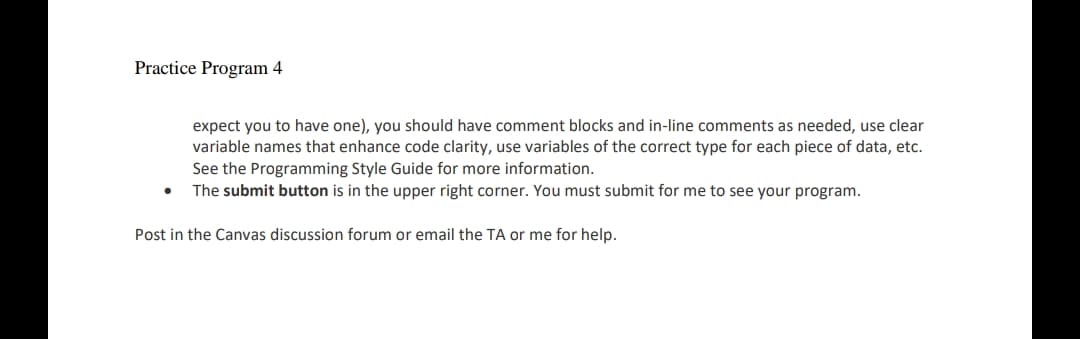
Transcribed Image Text:Practice Program 4
expect you to have one), you should have comment blocks and in-line comments as needed, use clear
variable names that enhance code clarity, use variables of the correct type for each piece of data, etc.
See the Programming Style Guide for more information.
The submit button is in the upper right corner. You must submit for me to see your program.
Post in the Canvas discussion forum or email the TA or me for help.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Recommended textbooks for you
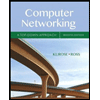
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
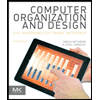
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
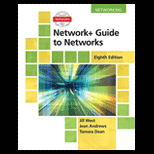
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
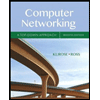
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
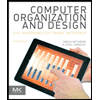
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
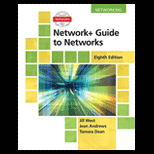
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
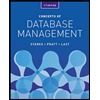
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
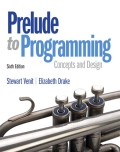
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
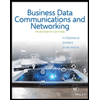
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY