Use following LinkedList code as a reference, add Find the average data values of the linked list. Find the node with largest key, and then delete the node. (Note, you must return the node, not just the largest key)Test this operations in the Main method. (display the average of the data values of the linked list, the largest key, the linked list before and after deleting the node with the largest key. Show screenshot import java.util.*; public class LinkedList { public Node header; public LinkedList() { header = null; } public final Node Search(int key) { Node current = header; while (current != null && current.item != key) { current = current.link; } return current; } public final void Append(int newItem) { Node newNode = new Node(newItem); newNode.link = header; header = newNode; } public final Node Remove() { Node x = header; if (header != null) { header = header.link; } return x; } public final Node searchPrevious(int key) { if (header == null) { return header; } else { Node current = header; while (!(current.link == null) && (current.link.item != key)) { current = current.link; } return current; } } public final void Insert(int newItem, int preKey) { Node current; Node newNode = new Node(newItem); current = Search(preKey); if (current == null) { System.out.println("there is no such preKey!"); } else { newNode.link = current.link; current.link = newNode; } }
- Use following LinkedList code as a reference, add Find the average data values of the linked list. Find the node with largest key, and then delete the node. (Note, you must return the node, not just the largest key)Test this operations in the Main method. (display the average of the data values of the linked list, the largest key, the linked list before and after deleting the node with the largest key. Show screenshot
import java.util.*;
public class LinkedList
{
public Node header;
public LinkedList()
{
header = null;
}
public final Node Search(int key)
{
Node current = header;
while (current != null && current.item != key)
{
current = current.link;
}
return current;
}
public final void Append(int newItem)
{
Node newNode = new Node(newItem);
newNode.link = header;
header = newNode;
}
public final Node Remove()
{
Node x = header;
if (header != null)
{
header = header.link;
}
return x;
}
public final Node searchPrevious(int key)
{
if (header == null)
{
return header;
}
else
{
Node current = header;
while (!(current.link == null) && (current.link.item != key))
{
current = current.link;
}
return current;
}
}
public final void Insert(int newItem, int preKey)
{
Node current;
Node newNode = new Node(newItem);
current = Search(preKey);
if (current == null)
{
System.out.println("there is no such preKey!");
}
else
{
newNode.link = current.link;
current.link = newNode;
}
}
public final void Delete(int key)
{
if (header == null) // The list is empty!
{
System.out.println("The list is empty!");
}
else
{
if (header.item == key) // header to be deleted.
{
header = header.link;
}
else
{
Node p = searchPrevious(key);
if (p.link == null)
{
System.out.println("There is no such item!");
}
else
{
p.link = p.link.link;
}
}
}
}
public final void ShowLinkedList()
{
if (header == null)
System.out.println("The list is empty!");
else
{
Node current = header;
System.out.printf("%1$s->", current.item);
while (!(current.link == null))
{
current = current.link;
System.out.printf("%1$s->", current.item);
}
System.out.printf("null");
System.out.println();
}
}
public final void PrintList()
{
if (header == null)
{
System.out.println("The list is empty!");
}
else
{
Node current = header;
System.out.println(current.item);
while (!(current.link == null))
{
current = current.link;
System.out.println(current.item);
}
}
}
}

// ----------- LinkedList.java ------------
class Node {
public int item;
public Node link;
Node(int num) {
item = num;
link = null;
}
}
public class LinkedList {
public Node header;
public LinkedList() {
header = null;
}
public final Node Search(int key) {
Node current = header;
while (current != null && current.item != key) {
current = current.link;
}
return current;
}
public final void Append(int newItem) {
Node newNode = new Node(newItem);
newNode.link = header;
header = newNode;
}
public final Node Remove() {
Node x = header;
if (header != null) {
header = header.link;
}
return x;
}
public final Node searchPrevious(int key)
{
if (header == null)
}
return header;
}
else
{
Node current = header;
while (!(current.link = = null) && (current.link.item ! = key))
{
current = current.link;
}
return current;
}
}
public final void Insert(int newItem, int preKey) {
Node current;
Node newNode = new Node(newItem);
current = Search(preKey);
if (current == null)
{
System.out.println("there is no such preKey!");
}
else
{
newNode.link = current.link;
current.link = newNode;
}
}
public final void Delete(int key)
{
if (header == null) // The list is empty!
{
System.out.println("The list is empty!");
}
else
{
if (header.item == key) // header to be deleted.
{
header = header.link;
}
else
{
Node p = searchPrevious(key);
if (p.link == null)
{
System.out.println("There is no such item!");
}
else
{
p.link = p.link.link;
}
}
}
}
public final void ShowLinkedList()
{
if (header == null)
System.out.println("The list is empty!");
else
{
Node current = header;
current = current.link;
System.out.printf("%1$s->", current.item);
}
System.out.printf("null");
System.out.println();
}
}
public final void PrintList()
{
if (header == null)
{
System.out.println("The list is empty!");
}
else
{
Node current = header;
System.out.println(current.item);
while (!(current.link == null))
{
current = current.link;
System.out.println(current.item);
}
}
}
// method getAverage() that returns the average of Node values
// in linked list.
public double getAverage()
{
//if header is null then average will be 0.
if(header == null)
{
return 0;
}
//to store average and sum of node values
double avg = 0.0;
double sum = 0;
//store header in current node.
Node current = header;
//count is the number of nodes in linked list.
int count = 0;
//till last node.
while (current ! = null)
{
//add current node value to the sum variable
sum += current.item;
//move to next node.
//increment the count of nodes.
count++;
}
//average is teh division of sum / number of nodes.
avg = sum / count;
//return the average.
return avg;
}
//function that returns the largest Node in the list.
public Node getLargestNode()
{
//if header is full then there is no Largest Node.
if(header == null)
{
return null;
}
//to store current node while processing.
Node current = header;
//set largest node value of item as the header node value.
Node largest = new Node9current.item);
//till end of last node.
while (current!=null)
{
//if current node value is greater than the largest item value.
if(current.item > largest.item)
{
//then largest item value will be current node value.
largest.item = current.item;
}
//move to next node.
current = current.link;
}
//return the largest node.
return largest;
}
}
//--------linkedListapplication.java--------
//calss that tests all the functionalities of LibkedList clas.
{
//crate linked list object
LinkedList list = new LinkedList();
//add values using Append() method
list.Append(5);
list.Append(1);
list.Append(2);
list.Append(3);
list.Append(4);
//display linked list.
System.out.println("\nLinked List After insertion using Append:()------");
list.ShowLinkedList();
//insert using Insert(10,3) which inserts 10 after key 3.
System.out.println("\nInserting 10 at previous key 3 using Insert() method");
list.Insert(10,3);
System.out.println("\nLinked List After insertion using Insert()------");
list.ShowLinkedList();
//call getAverage() and store the result
double avg = list.getAverage();
System.out.println(String.format("\nAverage of Linked List values using getAverage() method : %2f",avg));
//call getLargestNode() and store the result
Node largest = list.getLargestNode();
System.out.println("\nLargest value in Linked list:" +largest.item);
System.out.println('\nLinked List before deleting largest node: "+largest.item);
list.Printlist();
//remove the largest item value in linked list.
list.Delete(largest.item);
System.out.println("\n Linked List After Deleting Largest Node Value using Delete() method:
"+largest.item+"------");
list.ShowLinkedList();
System.out.println("\nLinked List before performing Remove() method");
list.ShowLinkedList();
//call Remove() method to remove head from linked list.
list.Remove();
System.out.println("\nLinked List After performing Remove() method");
list.ShowLinkedList();
}
}
Step by step
Solved in 2 steps with 2 images

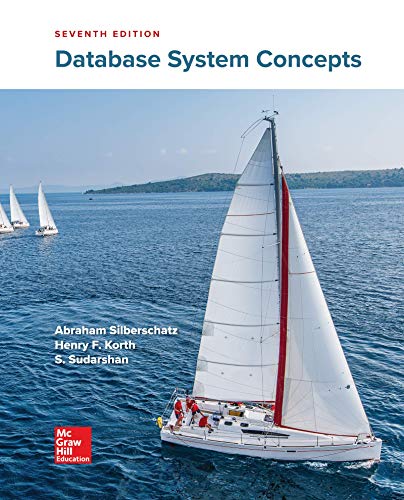
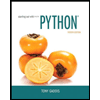
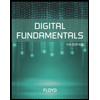
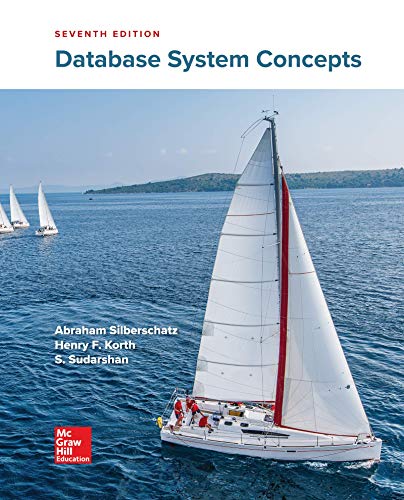
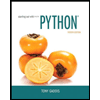
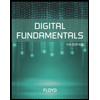
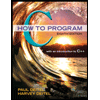
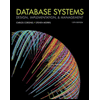
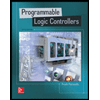