Use following main function to test your program. (have to use this main function) int main() { cout << endl; RatNum r1(1,2), r2(1,6), r3(2,5); // test operator overloads cout << "\nInput/Output Stream Operators: " << endl; RatNum r4; cout << "Enter a rational number: "; cin >> r4; cout <
Use following main function to test your program. (have to use this main function) int main() { cout << endl; RatNum r1(1,2), r2(1,6), r3(2,5); // test operator overloads cout << "\nInput/Output Stream Operators: " << endl; RatNum r4; cout << "Enter a rational number: "; cin >> r4; cout <
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
plz help with c++....and keep output same as given and paste indented code plzz
![Use following main function to test your program. (have to use this main function)
int main() {
}
cout << endl;
RatNum r1(1,2), r2(1,6), r3(2,5);
test operator overloads
cout << "\nInput/Output Stream Operators: " << endl;
RatNum r4;
cout << "Enter a rational number: ";
cin >> r4;
cout <<r4 << endl;
cout << "Negation Operation: " << endl;
cout <<-r4 << endl;
// test arithmetic overloads
cout << "\nArithmetic Operators: " << endl;
RatNum r5 r1 + r2;
cout << r1 <<"+"<<r2 << "=" <<r5 << endl;
RatNum r6 r1 r2;
cout << r1 <<"-" << r2 << " = " <<r6 << endl;
RatNum r7 r1 *r2;
cout << r1 <<"*" <<r2 << "="<<r7 << endl;
RatNum r8 r1/r2:
cout <<r1 <<"/" << r2 << " = " << r8 << endl;
// test arithmetic operation chaining
cout << "\nArithmetic Chaining: "<<endl;
RatNum r9 = r5 + r6 r7 * r8;
cout <<r5 << "+"<<r6 <<"-" <<r7 <<"*" << r8 <<" = " << r9 << endl;
// test relational operator overload
cout << "\nRelational Operators: " << endl;
cout << r5 << " == " << r6 <<"? " << (r5==r6) << endl;
cout <<r5 << " != " << r6 <<"? " << (r5!=r6) << endl;
cout <<r5 << ">" << r6 <<"? " << (r5>r6) << endl;
cout <<r5 <<"<" << r6 << "? " << (r5<r6) << endl;
// test subscript overload
cout << "\nSubscript Operator: "<<< endl;
cout <<r5 << "num=" <<r5[1] <<" den=" <<r5[2] << endl;
cout << endl;
return 0;
Output Example
Input/Output Stream Operators:
Enter a rational number: 1 2
1/2
Negation Operation:
-1/2
Arithmetic Operators:
1/2 + 1/6 = 2/3
1/2 1/6 1/3
1/2 1/6 1/12
1/2 1/6 3/1
Arithmetic Chaining:
2/3+1/3 1/12 * 3/1 = 3/4
Relational Operators:
2/3 = 1/3? 0
2/3 = 1/3? 1
2/3
1/3? 1
2/3<1/3? 0
Subscript Operator:
2/3 num=2 den=3](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa31cab6f-599e-46eb-9303-00837fd61b2b%2Fc9a2bd89-8498-412b-a65b-2a6a74646b4d%2Fiujxdp8_processed.png&w=3840&q=75)
Transcribed Image Text:Use following main function to test your program. (have to use this main function)
int main() {
}
cout << endl;
RatNum r1(1,2), r2(1,6), r3(2,5);
test operator overloads
cout << "\nInput/Output Stream Operators: " << endl;
RatNum r4;
cout << "Enter a rational number: ";
cin >> r4;
cout <<r4 << endl;
cout << "Negation Operation: " << endl;
cout <<-r4 << endl;
// test arithmetic overloads
cout << "\nArithmetic Operators: " << endl;
RatNum r5 r1 + r2;
cout << r1 <<"+"<<r2 << "=" <<r5 << endl;
RatNum r6 r1 r2;
cout << r1 <<"-" << r2 << " = " <<r6 << endl;
RatNum r7 r1 *r2;
cout << r1 <<"*" <<r2 << "="<<r7 << endl;
RatNum r8 r1/r2:
cout <<r1 <<"/" << r2 << " = " << r8 << endl;
// test arithmetic operation chaining
cout << "\nArithmetic Chaining: "<<endl;
RatNum r9 = r5 + r6 r7 * r8;
cout <<r5 << "+"<<r6 <<"-" <<r7 <<"*" << r8 <<" = " << r9 << endl;
// test relational operator overload
cout << "\nRelational Operators: " << endl;
cout << r5 << " == " << r6 <<"? " << (r5==r6) << endl;
cout <<r5 << " != " << r6 <<"? " << (r5!=r6) << endl;
cout <<r5 << ">" << r6 <<"? " << (r5>r6) << endl;
cout <<r5 <<"<" << r6 << "? " << (r5<r6) << endl;
// test subscript overload
cout << "\nSubscript Operator: "<<< endl;
cout <<r5 << "num=" <<r5[1] <<" den=" <<r5[2] << endl;
cout << endl;
return 0;
Output Example
Input/Output Stream Operators:
Enter a rational number: 1 2
1/2
Negation Operation:
-1/2
Arithmetic Operators:
1/2 + 1/6 = 2/3
1/2 1/6 1/3
1/2 1/6 1/12
1/2 1/6 3/1
Arithmetic Chaining:
2/3+1/3 1/12 * 3/1 = 3/4
Relational Operators:
2/3 = 1/3? 0
2/3 = 1/3? 1
2/3
1/3? 1
2/3<1/3? 0
Subscript Operator:
2/3 num=2 den=3
![2. Continuous of question 1. Implement operator overloading.
Operator Overloading
a) Implement two unary operator overload functions (-,+,!).
b) Implement four arithmetic operator overload functions (+,-,*,/).
c) Implement six relational operator overload functions (==, !=,>,>=,<,<=).
d) Implement the insertion operator overload function (<<).
e) Implement the extraction operator overload function (>>).
Implement the subscript operator overload function ([]).
Make sure that each function is optimally overloaded for its purpose.
(pick between member, non-member, friend as appropriate)
Notes
Add Rational Numbers
Given a/b + c/d:
Step 1: Find the LCM of b and d.
Step 2: Create a new Rational Number: ((a* (LCM/b) + (c* (LCM/d)) / LCM.
Step 3: Reduce the new Rational Number from step 2.
Step 4: Return the new Rational Number.
Subtract Rational Numbers
Given a/b - c/d:
Step 1: Find the LCM of b and d.
Step 2: Create a new Rational Number: ((a*(LCM/b) - (c* (LCM/d)) / LCM.
Step 3: Reduce the new Rational Number from step 2.
Step 4: Return the new Rational Number.
Multiply Rational Numbers
Given a/b * c/d:
Step 1: Create a new Rational Number: (a*c) / (b*d).
Step 2: Return the new Rational Number.
Divide Rational Numbers
Given a/b * c/d:
Step 1: Create a new Rational Number: (a*c) / (b*d).
Step 2: Return the new Rational Number.
Compare Rational Numbers: greater than
Determine if a/b> c/d:
Step 1: Find the LCM of b and d.
Step 2: If (a*(LCM/b) > (c* (LCM/d) return true, otherwise false.
Compare Rational Numbers: less than
Determine if a/b < c/d:
Step 1: Find the LCM of b and d.
Step 2: If (a*(LCM/b) < (c*(LCM/d) return true, otherwise false.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa31cab6f-599e-46eb-9303-00837fd61b2b%2Fc9a2bd89-8498-412b-a65b-2a6a74646b4d%2Fg53trpm_processed.png&w=3840&q=75)
Transcribed Image Text:2. Continuous of question 1. Implement operator overloading.
Operator Overloading
a) Implement two unary operator overload functions (-,+,!).
b) Implement four arithmetic operator overload functions (+,-,*,/).
c) Implement six relational operator overload functions (==, !=,>,>=,<,<=).
d) Implement the insertion operator overload function (<<).
e) Implement the extraction operator overload function (>>).
Implement the subscript operator overload function ([]).
Make sure that each function is optimally overloaded for its purpose.
(pick between member, non-member, friend as appropriate)
Notes
Add Rational Numbers
Given a/b + c/d:
Step 1: Find the LCM of b and d.
Step 2: Create a new Rational Number: ((a* (LCM/b) + (c* (LCM/d)) / LCM.
Step 3: Reduce the new Rational Number from step 2.
Step 4: Return the new Rational Number.
Subtract Rational Numbers
Given a/b - c/d:
Step 1: Find the LCM of b and d.
Step 2: Create a new Rational Number: ((a*(LCM/b) - (c* (LCM/d)) / LCM.
Step 3: Reduce the new Rational Number from step 2.
Step 4: Return the new Rational Number.
Multiply Rational Numbers
Given a/b * c/d:
Step 1: Create a new Rational Number: (a*c) / (b*d).
Step 2: Return the new Rational Number.
Divide Rational Numbers
Given a/b * c/d:
Step 1: Create a new Rational Number: (a*c) / (b*d).
Step 2: Return the new Rational Number.
Compare Rational Numbers: greater than
Determine if a/b> c/d:
Step 1: Find the LCM of b and d.
Step 2: If (a*(LCM/b) > (c* (LCM/d) return true, otherwise false.
Compare Rational Numbers: less than
Determine if a/b < c/d:
Step 1: Find the LCM of b and d.
Step 2: If (a*(LCM/b) < (c*(LCM/d) return true, otherwise false.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
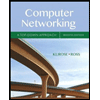
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
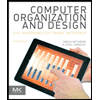
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
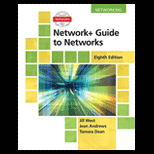
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
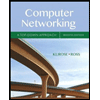
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
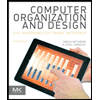
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
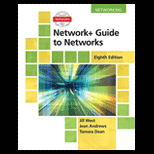
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
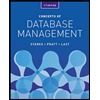
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
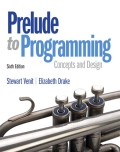
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
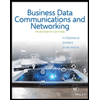
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY