Use java code to complete. Please zoom in if difficult to re
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Use java code to complete. Please zoom in if difficult to read.
![Program 1:
1. Create a class mimicking some of the features of Netflix. You may name it class Netflix.
2. Add the following class variables to it, for example:
a. String array which holds say any 10 Netflix series/movies (fill the array in the
constructor).
b. String array which holds 5 “Continue Watching" items (fill the array in the
constructor).
c. String array which holds Top 5 “Trending" items(fill the array using no-argument or
parameterized constructor).
d. String username
e. String password
f. boolean isAuthenticated. This should be a 'private' class variable.
g. double balance remaining for the user
h. int subscriber since, like subscriber since 2015
3. Add the following class methods to it:
a. A method that asks for the username and password of the user.
Note: Because we do not have any database, just assume all username/password
combinations are valid, so set the isAuthenticated variable to true and print a login
successful message inside this method with the value of isAuthenticated variable.
b. A method that prints Top 5 Trending items in ascending order.
(Use Arrays.sort() library method to quickly sort arrays).
c. A method that prints logged in username, remaining balance and subscriber since
information.
d. A method (like updateContinueWatching(String strValue) which takes a String
parameter and updates the Continue Watching array for the user. So in NetflixDemo
class, take a movie or TV show name as input from the user and pass it to this
updateContinueWatching(value) method. Print the final Continue Watching array.
Note: New value should take first position in the Continue Watching array (index 0).
For example: Say your current Continue Watching array is:
["Ozark", “Narcos", “Schitts Creek", “The Crown", “Better Call Saul"]
And you are now adding “Breaking Bad", then the updated Continue Watching array
should look like this:
["Breaking Bad", “Ozark", “Narcos", "Schitts Creek", “The Crown"]
So, move all items to the right by 1 (and we lose the last item) and put the new value
at index 0.
4. Create another class called NetflixDemo.
a. Create two objects “user1" and "user2" of the Netflix class.
b. Set various class variables for the Netflix class using these two objects.
c. Call various methods of Netflix class using these two objects.
Notes:
1. Use try-catch block to handle any exception that might occur due to user input.
2. Write Javadoc at the top of the classes with author name and brief description of the class.
3. Please submit the java program files and screenshot of the output.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5d2922c3-0bc7-4b62-b2ff-a839126be135%2F48d9e71b-a04b-462c-875f-9c85f2adc3e4%2Fc67vmcs_processed.png&w=3840&q=75)
Transcribed Image Text:Program 1:
1. Create a class mimicking some of the features of Netflix. You may name it class Netflix.
2. Add the following class variables to it, for example:
a. String array which holds say any 10 Netflix series/movies (fill the array in the
constructor).
b. String array which holds 5 “Continue Watching" items (fill the array in the
constructor).
c. String array which holds Top 5 “Trending" items(fill the array using no-argument or
parameterized constructor).
d. String username
e. String password
f. boolean isAuthenticated. This should be a 'private' class variable.
g. double balance remaining for the user
h. int subscriber since, like subscriber since 2015
3. Add the following class methods to it:
a. A method that asks for the username and password of the user.
Note: Because we do not have any database, just assume all username/password
combinations are valid, so set the isAuthenticated variable to true and print a login
successful message inside this method with the value of isAuthenticated variable.
b. A method that prints Top 5 Trending items in ascending order.
(Use Arrays.sort() library method to quickly sort arrays).
c. A method that prints logged in username, remaining balance and subscriber since
information.
d. A method (like updateContinueWatching(String strValue) which takes a String
parameter and updates the Continue Watching array for the user. So in NetflixDemo
class, take a movie or TV show name as input from the user and pass it to this
updateContinueWatching(value) method. Print the final Continue Watching array.
Note: New value should take first position in the Continue Watching array (index 0).
For example: Say your current Continue Watching array is:
["Ozark", “Narcos", “Schitts Creek", “The Crown", “Better Call Saul"]
And you are now adding “Breaking Bad", then the updated Continue Watching array
should look like this:
["Breaking Bad", “Ozark", “Narcos", "Schitts Creek", “The Crown"]
So, move all items to the right by 1 (and we lose the last item) and put the new value
at index 0.
4. Create another class called NetflixDemo.
a. Create two objects “user1" and "user2" of the Netflix class.
b. Set various class variables for the Netflix class using these two objects.
c. Call various methods of Netflix class using these two objects.
Notes:
1. Use try-catch block to handle any exception that might occur due to user input.
2. Write Javadoc at the top of the classes with author name and brief description of the class.
3. Please submit the java program files and screenshot of the output.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 6 images

Recommended textbooks for you
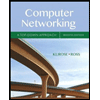
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
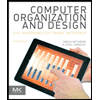
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
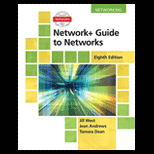
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
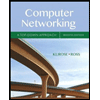
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
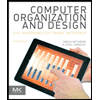
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
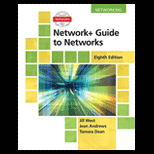
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
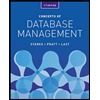
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
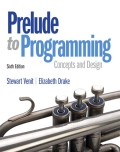
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
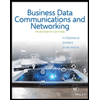
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY