USE PYTHON THANKS
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
USE PYTHON THANKS
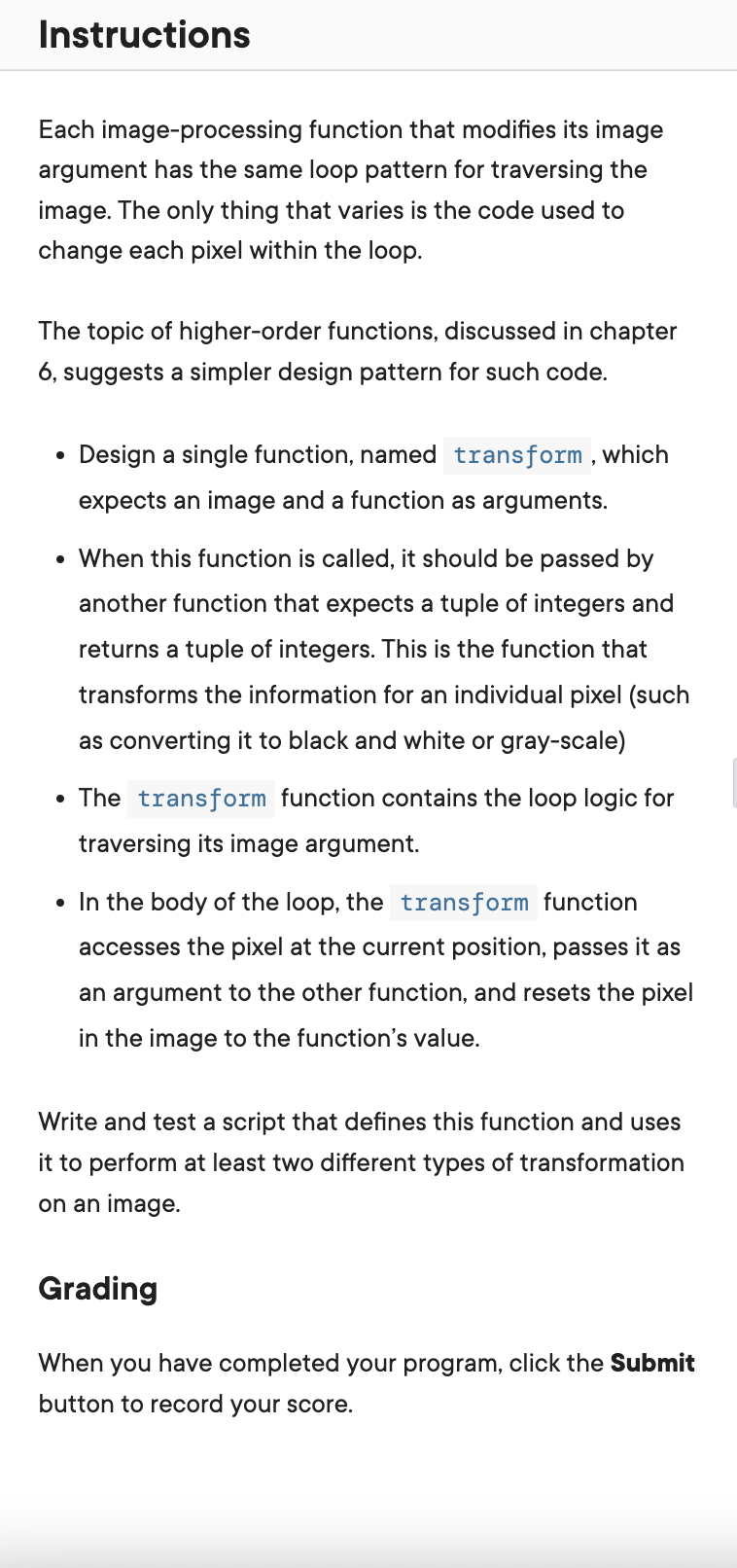
Transcribed Image Text:Instructions
Each image-processing function that modifies its image
argument has the same loop pattern for traversing the
image. The only thing that varies is the code used to
change each pixel within the loop.
The topic of higher-order functions, discussed in chapter
6, suggests a simpler design pattern for such code.
●
Design a single function, named transform, which
expects an image and a function as arguments.
• When this function is called, it should be passed by
another function that expects a tuple of integers and
returns a tuple of integers. This is the function that
transforms the information for an individual pixel (such
as converting it to black and white or gray-scale)
• The transform function contains the loop logic for
traversing its image argument.
• In the body of the loop, the transform function
accesses the pixel at the current position, passes it as
an argument to the other function, and resets the pixel
in the image to the function's value.
Write and test a script that defines this function and uses
it to perform at least two different types of transformation
on an image.
Grading
When you have completed your program, click the Submit
button to record your score.
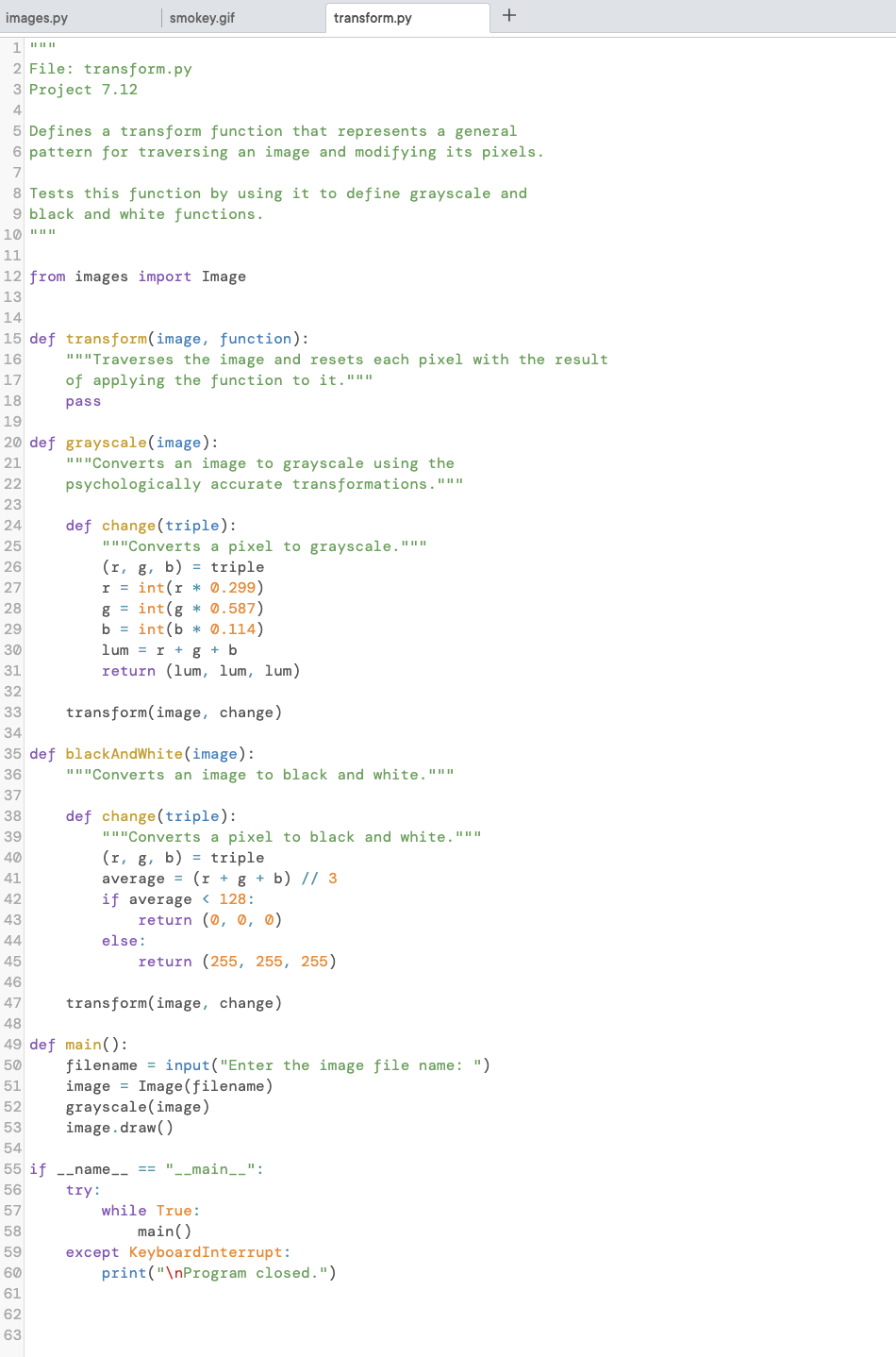
Transcribed Image Text:images.py
1 ***
2 File: transform.py
3 Project 7.12
4
smokey.gif
5 Defines a transform function that represents a general
6 pattern for traversing an image and modifying its pixels.
7
14
15 def transform(image, function):
16
17
18
19
20 def grayscale (image):
21
22
23
24
25
26
27
28
29
30
31
32
33
8 Tests this function by using it to define grayscale and
9 black and white functions.
10 """
11
12 from images import Image
13
62
63
"""Traverses the image and resets each pixel with the result
of applying the function to it."""
pass
"""Converts an image to grayscale using the
psychologically accurate transformations. """
def change (triple):
"""Converts a pixel to grayscale."""
(r, g, b) = triple
r = int(r * 0.299)
g= int(g* 0.587)
b = int(b* 0.114)
lum = r + g + b
return (lum, lum, lum)
transform(image, change)
34
35 def blackAndWhite (image):
36
37
38
39
40
41
42
43
44
45
46
47
48
49 def main():
50
51
52
53
54
55 if __name__ == "__main__":
56
try:
57
58
59
60
61
transform.py
"""Converts an image to black and white."""
def change (triple):
"""Converts a pixel to black and white."""
(r, g, b) = triple
average
(r + g + b) // 3
128:
if average
else:
return (0, 0, 0)
return (255, 255, 255)
transform(image, change)
filename=input("Enter the image file name: ")
image Image (filename)
grayscale (image)
image.draw()
while True:
main()
+
except KeyboardInterrupt:
print("\nProgram closed.")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
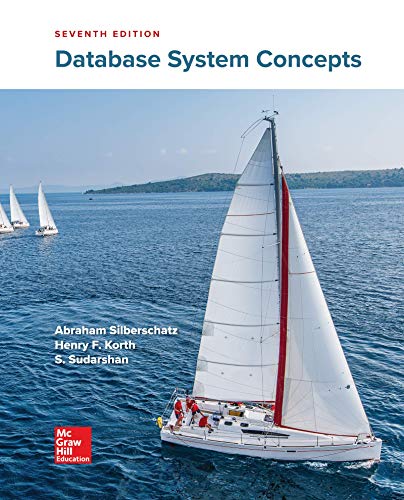
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
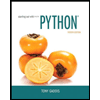
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
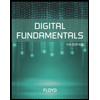
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
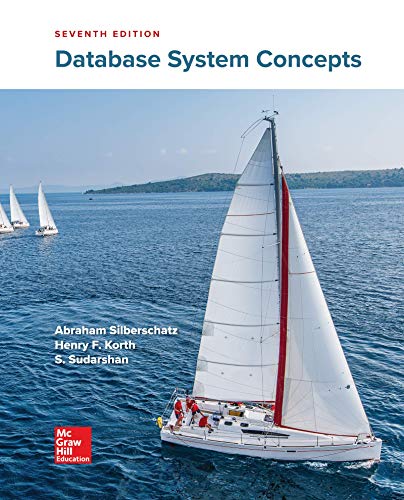
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
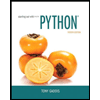
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
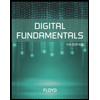
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
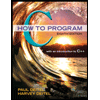
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
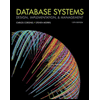
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
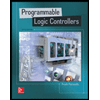
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education