Use the following code which adds and prints elements of a simply linked list, and add a member function that will duplicate nonzero value nodes in a list. Call your function properly in main and print the list again. #include using namespace std; struct node { int data; node *next; node(int d,node*n=0) { data=d; next=n; } }; class list { node *head; public: list() { head=0; } void print() { for (node *t=head;t!=0;t=t->next) cout<data<<" "; cout<
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Use the following code which adds and prints elements of a simply linked list, and add a member function that will duplicate nonzero value nodes in a list. Call your function properly in main and print the list again.
#include <iostream>
using namespace std;
struct node
{
int data;
node *next;
node(int d,node*n=0)
{ data=d; next=n; }
};
class list
{
node *head;
public:
list() { head=0; }
void print()
{
for (node *t=head;t!=0;t=t->next) cout<<t->data<<" ";
cout<<endl;
}
void add(int el)
{
head=new node(el,head);
}
};
void main()
{
list l;
l.add(0);
l.add(7);
l.add(1);
l.add(0);
l.add(3);
l.print();
}

Step by step
Solved in 3 steps with 1 images

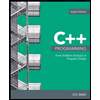
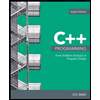