USE the Node, StackArray, StackRef and Stack interface classes PROVIDED BELOW to create code that reads input from the sample Data File PROVIDED to solve PLEASE READ DIRECTIONs VERY CAREFULLY thanks for your help Data File 2 3 4 + + 12 6 / 4 * 4 + 2 2 3 3 * * * 7 5 / 5 + 17 - 4 3 - 5 6 + 4 * - 1 1 + 3 * 4 - 10 ^ public class Node
USE the Node, StackArray, StackRef and Stack interface classes PROVIDED BELOW to create code that reads input from the sample Data File PROVIDED to solve
PLEASE READ DIRECTIONs VERY CAREFULLY
thanks for your help
Data File
2 3 4 + +
12 6 / 4 * 4 +
2 2 3 3 * * *
7 5 / 5 + 17 -
4 3 - 5 6 + 4 * -
1 1 + 3 * 4 - 10 ^
public class Node <T>
{
private T data;
private Node<T> next;
public Node(T data, Node<T> next)
{
this.data = data;
this.next = next;
}
public T getData()
{
return data;
}
public Node<T> getNext()
{
return next;
}
}
import java.util.Stack;
public class StackArray<T> implements Stack<T> {
private T [] elements;
private int top;
private int size;
public StackArray (int size)
{
elements = (T[]) new Object[size];
top = -1;
this.size = size;
}
public boolean empty()
{
return top == -1;
}
public boolean full()
{
return top == size - 1;
}
public boolean push(T el)
{
if (full())
return false;
else
{
top++;
elements[top] = el;
return true;
}
}
public T pop()
{
T el = elements[top];
top--;
return el;
}
}
// Generic Stack Reference Implementation
public class StackRef<T> implements Stack<T>
{
private Node<T> top;
public StackRef (int size)
{
top = null;
}
public StackRef()
{
top = null;
}
public boolean empty()
{
return top == null;
}
public boolean full()
{
return false;
}
public boolean push(T el)
{
Node<T> node = new Node<>(el, top);
top = node;
return true;
}
public T pop()
{
if (empty())
return null;
T el = top.getData();
top = top.getNext();
return el;
}
}
// Generic Stack Interface
public interface Stack<T>
{
public boolean empty();
public boolean full();
public boolean push(T el);
public T pop();
}
import java.util.Scanner;
import java.util.Stack;
import java.util.Set;
import java.io.*;
import java.util.*;
public class RPN {
private static Stack<String> stack;
private static FileReader file;
private static Scanner in;
private static Set<String> operators;
}
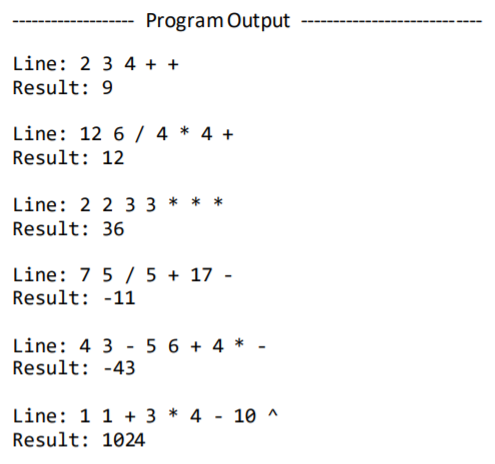
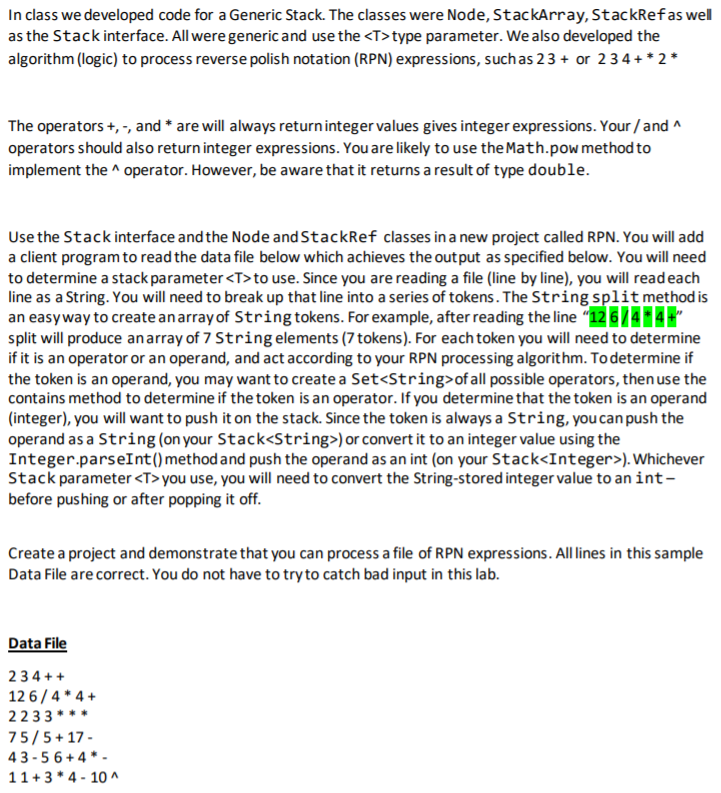

Please follow the link:
https://www.bartleby.com/questions-and-answers/line-2-3-4-result-9-line-12-6-4-4-result-12-line-2-2-3-3-result-36-line-7-5-5-17-result-11-line-4-3-/af88e3f4-c3f8-418b-9dfd-2091a6834bb8
Step by step
Solved in 2 steps

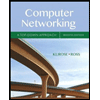
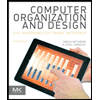
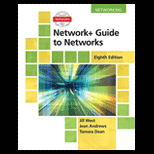
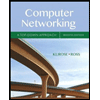
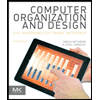
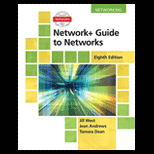
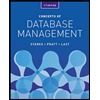
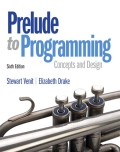
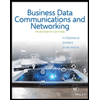