using python "## 9. With Keyword Arguments\n", Now write a function called `series` that allows you to specify a series of integers to be returned in a list. The function should accept keyword arguments as follows:\n", "- `start` Which specifies the start of the series. This is optional. If it is not given, the series should start at zero\n", "- `end` Which specifies the largest value in the series. This is a mandatory argument.\n", "- `step` Which specifies the size of the steps from one number to the next\n", "\n", "Call your function so it produces the following outputs:\n", "\n", "- `[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]`\n", "- `[5, 6, 7, 8, 9, 10]`\n", "- `[0, 2, 4, 6, 8, 10]`\n", "- `[5, 7, 9]`\n", "\n", "Only pass the arguments that are needed each time." "## 10. Generators\n", "\n", "Write a generator that accepts a string as an input and yields each character in the string, one at a time. Initialise the generator and use it to extract the first three characters of the string \"This is my generator\". Print those three characters next to each other. Then use two more lines of code to print the rest of the characters, each on its own line." "## 11. Now something a little more challenging\n", "\n", "If you cube each integer in turn from 10 to one million, you will see that 11 of them produce a result that is a palindrome (the same forwards as backwards). For example, $11 \\times 11 \\times 11 = 1331$.\n", "\n", "Write a single line of code to produce those 11 numbers and their cubes in a list of tuples.\n", "\n", "The best solution can be done in one line of code. Add comments to explain what the code does.\n", "\n", "Shame about 2201, isn't it?!" "## 12. Finally, some JSON and Recursion\n", "\n", "George runs a company. He manages James and Jamila, who each have a small team to manage. In James' team are Jill and Jenny. In Jamila's team are Jewel, Jasmine and Jeremy.\n", "\n", "Create a JSON object in a string variable called `company` where each item has a `name` field and a field called `manages` which contains an array of the people managed by that person. If a person does not manage anybody, they have no field called manages.\n", "\n", "Then convert the JSON string to a dictionary in a variable called `company_dict`.\n", "\n", "Finally, write a recursive function that accepts the dictionary as an argument and lists each person and their level in the organisation, so that George is at level 1, James and Jamila are at 2, and so on. Call the function and print the result. It should look like this:\n", "\n", "`George is at level 1\n", "James is at level 2\n", "Jill is at level 3\n", "Jenny is at level 3\n", "Jamila is at level 2\n", "Jewel is at level 3\n", "Jasmine is at level 3\n", "Jeremey is at level 3`\n", "\n", "The function can either return a string, which you print after it has been called, or it can print its results as it runs and return nothing."
using python "## 9. With Keyword Arguments\n", Now write a function called `series` that allows you to specify a series of integers to be returned in a list. The function should accept keyword arguments as follows:\n", "- `start` Which specifies the start of the series. This is optional. If it is not given, the series should start at zero\n", "- `end` Which specifies the largest value in the series. This is a mandatory argument.\n", "- `step` Which specifies the size of the steps from one number to the next\n", "\n", "Call your function so it produces the following outputs:\n", "\n", "- `[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]`\n", "- `[5, 6, 7, 8, 9, 10]`\n", "- `[0, 2, 4, 6, 8, 10]`\n", "- `[5, 7, 9]`\n", "\n", "Only pass the arguments that are needed each time." "## 10. Generators\n", "\n", "Write a generator that accepts a string as an input and yields each character in the string, one at a time. Initialise the generator and use it to extract the first three characters of the string \"This is my generator\". Print those three characters next to each other. Then use two more lines of code to print the rest of the characters, each on its own line." "## 11. Now something a little more challenging\n", "\n", "If you cube each integer in turn from 10 to one million, you will see that 11 of them produce a result that is a palindrome (the same forwards as backwards). For example, $11 \\times 11 \\times 11 = 1331$.\n", "\n", "Write a single line of code to produce those 11 numbers and their cubes in a list of tuples.\n", "\n", "The best solution can be done in one line of code. Add comments to explain what the code does.\n", "\n", "Shame about 2201, isn't it?!" "## 12. Finally, some JSON and Recursion\n", "\n", "George runs a company. He manages James and Jamila, who each have a small team to manage. In James' team are Jill and Jenny. In Jamila's team are Jewel, Jasmine and Jeremy.\n", "\n", "Create a JSON object in a string variable called `company` where each item has a `name` field and a field called `manages` which contains an array of the people managed by that person. If a person does not manage anybody, they have no field called manages.\n", "\n", "Then convert the JSON string to a dictionary in a variable called `company_dict`.\n", "\n", "Finally, write a recursive function that accepts the dictionary as an argument and lists each person and their level in the organisation, so that George is at level 1, James and Jamila are at 2, and so on. Call the function and print the result. It should look like this:\n", "\n", "`George is at level 1\n", "James is at level 2\n", "Jill is at level 3\n", "Jenny is at level 3\n", "Jamila is at level 2\n", "Jewel is at level 3\n", "Jasmine is at level 3\n", "Jeremey is at level 3`\n", "\n", "The function can either return a string, which you print after it has been called, or it can print its results as it runs and return nothing."
Chapter8: Arrays
Section: Chapter Questions
Problem 5PE
Related questions
Question
using python "## 9. With Keyword Arguments\n", Now write a function called `series` that allows you to specify a series of integers to be returned in a list. The function should accept keyword arguments as follows:\n", "- `start` Which specifies the start of the series. This is optional. If it is not given, the series should start at zero\n", "- `end` Which specifies the largest value in the series. This is a mandatory argument.\n", "- `step` Which specifies the size of the steps from one number to the next\n", "\n", "Call your function so it produces the following outputs:\n", "\n", "- `[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]`\n", "- `[5, 6, 7, 8, 9, 10]`\n", "- `[0, 2, 4, 6, 8, 10]`\n", "- `[5, 7, 9]`\n", "\n", "Only pass the arguments that are needed each time." "## 10. Generators\n", "\n", "Write a generator that accepts a string as an input and yields each character in the string, one at a time. Initialise the generator and use it to extract the first three characters of the string \"This is my generator\". Print those three characters next to each other. Then use two more lines of code to print the rest of the characters, each on its own line." "## 11. Now something a little more challenging\n", "\n", "If you cube each integer in turn from 10 to one million, you will see that 11 of them produce a result that is a palindrome (the same forwards as backwards). For example, $11 \\times 11 \\times 11 = 1331$.\n", "\n", "Write a single line of code to produce those 11 numbers and their cubes in a list of tuples.\n", "\n", "The best solution can be done in one line of code. Add comments to explain what the code does.\n", "\n", "Shame about 2201, isn't it?!" "## 12. Finally, some JSON and Recursion\n", "\n", "George runs a company. He manages James and Jamila, who each have a small team to manage. In James' team are Jill and Jenny. In Jamila's team are Jewel, Jasmine and Jeremy.\n", "\n", "Create a JSON object in a string variable called `company` where each item has a `name` field and a field called `manages` which contains an array of the people managed by that person. If a person does not manage anybody, they have no field called manages.\n", "\n", "Then convert the JSON string to a dictionary in a variable called `company_dict`.\n", "\n", "Finally, write a recursive function that accepts the dictionary as an argument and lists each person and their level in the organisation, so that George is at level 1, James and Jamila are at 2, and so on. Call the function and print the result. It should look like this:\n", "\n", "`George is at level 1\n", "James is at level 2\n", "Jill is at level 3\n", "Jenny is at level 3\n", "Jamila is at level 2\n", "Jewel is at level 3\n", "Jasmine is at level 3\n", "Jeremey is at level 3`\n", "\n", "The function can either return a string, which you print after it has been called, or it can print its results as it runs and return nothing."
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
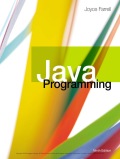
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
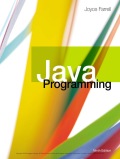
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT