C++ Create a class using only 1 file For each class create these features: A private variable that can only be accessed by that class. A protected variable that can only be accessed by that class or children of that class. Getter and Setter functions for accessing the private data of the class. A Constructor that outputs the name of the class whenever it is used to instantiate or construct a class object. Example : "Class A object constructed." Comments that describe exactly how the class is structured and what are it's features (superclass, subclass, overloading, etc). Add any variables or functions you deem necessary, but be sure to describe how they work using comments and the couts as required below These are the classes: Multi-level Classes consisting of 3 classes (A, B, and C, with A as top superclass). In class A, add a set of public overloading functions for printing out different data types. Example: print(int param), print(double param), and print(string param Multiple Classes consisting of 2 new classes (C and D as superclasses and E as subclass). Class C is the same class as in the Multi-level classes above. Hierarachical Classes consisting of 2 new classes (D as the superclass with E, F, and G as subclasses). Classes D and E are the same classes as described in the Multiple Classes above Function main() should show off all the features of the classes including: Use "cout" to describe exactly what is going on so that I don't have to guess and can easily grade it. Example: "Object a has been instantiated from class A." "Observe how function overloading works in a class A object..." "Observe how function overloading works in a class B object, a child of class A..." "See how private_x can be changed directly..." "See how private_x can be changed by object g..." "Object c has been instantiated."
C++ Create a class using only 1 file For each class create these features: A private variable that can only be accessed by that class. A protected variable that can only be accessed by that class or children of that class. Getter and Setter functions for accessing the private data of the class. A Constructor that outputs the name of the class whenever it is used to instantiate or construct a class object. Example : "Class A object constructed." Comments that describe exactly how the class is structured and what are it's features (superclass, subclass, overloading, etc). Add any variables or functions you deem necessary, but be sure to describe how they work using comments and the couts as required below These are the classes: Multi-level Classes consisting of 3 classes (A, B, and C, with A as top superclass). In class A, add a set of public overloading functions for printing out different data types. Example: print(int param), print(double param), and print(string param Multiple Classes consisting of 2 new classes (C and D as superclasses and E as subclass). Class C is the same class as in the Multi-level classes above. Hierarachical Classes consisting of 2 new classes (D as the superclass with E, F, and G as subclasses). Classes D and E are the same classes as described in the Multiple Classes above Function main() should show off all the features of the classes including: Use "cout" to describe exactly what is going on so that I don't have to guess and can easily grade it. Example: "Object a has been instantiated from class A." "Observe how function overloading works in a class A object..." "Observe how function overloading works in a class B object, a child of class A..." "See how private_x can be changed directly..." "See how private_x can be changed by object g..." "Object c has been instantiated."
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++
Create a class using only 1 file
- For each class create these features:
- A private variable that can only be accessed by that class.
- A protected variable that can only be accessed by that class or children of that class.
- Getter and Setter functions for accessing the private data of the class.
- A Constructor that outputs the name of the class whenever it is used to instantiate or construct a class object.
- Example : "Class A object constructed."
- Comments that describe exactly how the class is structured and what are it's features (superclass, subclass, overloading, etc).
- Add any variables or functions you deem necessary, but be sure to describe how they work using comments and the couts as required below
These are the classes:
- Multi-level Classes consisting of 3 classes (A, B, and C, with A as top superclass).
- In class A, add a set of public overloading functions for printing out different data types.
- Example: print(int param), print(double param), and print(string param
- In class A, add a set of public overloading functions for printing out different data types.
- Multiple Classes consisting of 2 new classes (C and D as superclasses and E as subclass).
- Class C is the same class as in the Multi-level classes above.
- Hierarachical Classes consisting of 2 new classes (D as the superclass with E, F, and G as subclasses).
- Classes D and E are the same classes as described in the Multiple Classes above
- Function main() should show off all the features of the classes including:
- Use "cout" to describe exactly what is going on so that I don't have to guess and can easily grade it.
- Example:
- "Object a has been instantiated from class A."
- "Observe how function overloading works in a class A object..."
- "Observe how function overloading works in a class B object, a child of class A..."
- "See how private_x can be changed directly..."
- "See how private_x can be changed by object g..."
- "Object c has been instantiated."
- "Object a has been instantiated from class A."
- Example:
- Use "cout" to describe exactly what is going on so that I don't have to guess and can easily grade it.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
Using that code, add this features
- Multi-level Classes consisting of 3 classes (A, B, and C, with A as top superclass).
- Use polymorphism for one function, get_description().
- Mark object the get_desciption() function in class C as "override final" so that polymorphism stops at class C.
- Multiple Classes consisting of 2 new classes (C and D as superclasses and E as subclass).
- Class C is the same class as in the Multi-level classes above.
- Make superclass D an Abstract Class by declaring a virtual function within the class equal to zero, "= 0".
- Hierarachical Classes consisting of 2 new classes (D as the superclass with E, F, and G as subclasses).
- Classes D and E are the same classes as described in the Multiple Classes above.
- A Friend Function declared in class F, called "get_private_f()".
- Create a private variable, "private_f" and give it a value for the friend function to access.
- Have class G use a public static variable, "object_num" which increments every time the class G is instantiated, thus counting the amount of objects constructed by class G.
- Do this by incrementing the "object_num" variable within the constructor of class G.
- Also be sure you have the memory space reserved for object_num.
- Also within class G, have a private static variable, "private_x" with public static functions get_x() and set_x() used as getter and setter functions for the static variable private_x.
For each class also create these features:
- A Constructor that outputs the name of the class whenever it is used to instantiate a class object (like F f;).
- A Destructor that outputs the name of the class.
- Comments that describe exactly how the class is structured and what are it's features (superclass, subclass, virtual, polymorphism, abstract, static variables/functions, etc.).
- Add any variables or functions you deem necessary, but be sure to describe how they work using comments and the couts as required below.
- Function main() should show off all the features of the classes including:
- Show first how the static variable, private_x can have a value and be changed before any classes are instantiated.
- Use "cout" to describe exactly what is going on so that I don't have to guess and can easily grade it. If I have to hunt for features, the grade will be lower.
- Examples:
- "The static variable, private_x is first given the value 6 before any objects are instantiated."
- "Here is how private_x can be changed before any objects are instantiated..."
- "See how private_x can be changed directly..."
- "See how private_x can be changed by object g..."
- "Object c has been instantiated."
- "Object g has been instantiated and now class G has constructed 3 objects via the static variable, object_num."
- "The get_description function in class C is the final function to override
- "Watch how the Friend Function in class F can access data from private_f..."
- "Class D cannot construct an object because it is an Abstract class, yet it passes on the function, _____() through hierarchical inheritance to its subclasses E, F and G...."
- Examples:
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
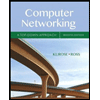
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
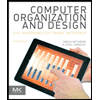
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
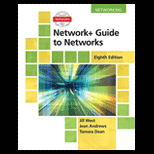
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
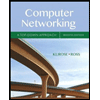
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
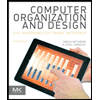
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
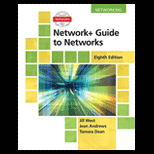
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
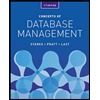
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
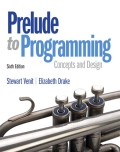
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
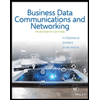
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY