Using the code in part I of this lab (included below): 1. Create a function to INPUT the information for the movie. Pass the empty structure in as a parameter. 2. Create a function to OUTPUT the information in the structure. Pass the filled structure in as a parameter. 3. Place the ENUM definition, STRUCTURE definition, and FUNCTION prototypes in a HEADER FILE (*.h). Remove those definitions from the main program and include your header file in the main program file. DECLARE the STRUCTURE VARIABLE as a LOCAL (not global) variable in the main program. If you like to include the following line of code: system("pause"); Here is my code from the first part of the lab: #include <iostream> #include <string> using namespace std; //enum Ratings enum Ratings { G = 1,PG,PG_13,R,NC_17 }; //structure movie struct Movie { string name; enum Ratings rating; string year_produced; string main_star; char saw; char liked; }; //function to read rating from user void read_rating(Movie &first) { char temp1; int temp; cin >> temp1; temp = temp1 - '0'; switch (temp) { case 1: first.rating = G; break; case 2: first.rating = PG; break; case 3: first.rating = PG_13; break; case 4: first.rating = R; break; case 5: first.rating = NC_17; break; default: cout << "\nPlease enter a valid rating "; read_rating(first); } } //function to display rating of enum type void display_rating(enum Ratings temp) { switch (temp) { case 1: cout << "\nThis movie is rated G: General Audiences\n"; break; case 2: cout << "\nThis movie is rated PG : Parental Guidance Suggested\n"; break; case 3: cout << "\nThis movie is rated PG-13: Parent's Strongly Cautioned\n"; break; case 4: cout << "\nThis movie is rated R: Under 17 requires accompanying adult or adult guardian\n"; break; case 5: cout << "\nThis movie is rated NC-17: No one under 17 and under admitted\n"; break; } } int main() { //create object of Movie Movie first; cout << "\nEnter name of Movie "; //read name of movie cin >> first.name; cout << "\nEnter Rating of movie from the following\n"; cout << "\n1 - G\n2 - PG\n3 - PG-13\n4 - R\n5 - NC-17\n\n"; //read rating of movie read_rating(first); cout << "\nEnter year produced "; //read year produced of movie cin >> first.year_produced; cout << "\nEnter main star of movie "; //read main star of movie cin >> first.main_star; cout << "\nPress Y if you saw the movie else Press N if you didn't saw the movie "; //read if user has saw the movie cin >> first.saw; cout << "\nPress Y if you liked the movie else Press N if you didn't liked the movie "; //read if user liked the movie cin >> first.liked; //print information about movie cout << "\n\nName of movie is " << first.name << endl; cout << "\nThe movie is produced in year: " << first.year_produced << endl; cout << "\nMain star of Movie is " << first.main_star << endl; display_rating(first.rating); if (first.saw == 'Y' || first.saw == 'y') cout << "\nYou saw the movie\n"; else cout << "\nYou did not see the movie\n"; if (first.liked == 'Y' || first.liked == 'y') cout << "\nYou liked the movie\n"; else cout << "\nYou did not like the movie\n"; cout << endl; }
Using the code in part I of this lab (included below):
1. Create a function to INPUT the information for the movie. Pass the empty structure in as a parameter.
2. Create a function to OUTPUT the information in the structure. Pass the filled structure in as a parameter.
3. Place the ENUM definition, STRUCTURE definition, and FUNCTION prototypes in a HEADER FILE (*.h).
Remove those definitions from the main program and include your header file in the main program file.
DECLARE the STRUCTURE VARIABLE as a LOCAL (not global) variable in the main program.
If you like to include the following line of code: system("pause");
Here is my code from the first part of the lab:
#include <string> using namespace std; //enum Ratings enum Ratings { G = 1, }; //structure movie struct Movie { string name; enum Ratings rating; string year_produced; string main_star; char saw; char liked; }; //function to read rating from user void read_rating(Movie &first) { char temp1; int temp; cin >> temp1; temp = temp1 - '0'; switch (temp) { case 1: first.rating = G; break; case 2: first.rating = PG; break; case 3: first.rating = PG_13; break; case 4: first.rating = R; break; case 5: first.rating = NC_17; break; default: cout << "\nPlease enter a valid rating "; read_rating(first); } } //function to display rating of enum type void display_rating(enum Ratings temp) { switch (temp) { case 1: cout << "\nThis movie is rated G: General Audiences\n"; break; case 2: cout << "\nThis movie is rated PG : Parental Guidance Suggested\n"; break; case 3: cout << "\nThis movie is rated PG-13: Parent's Strongly Cautioned\n"; break; case 4: cout << "\nThis movie is rated R: Under 17 requires accompanying adult or adult guardian\n"; break; case 5: cout << "\nThis movie is rated NC-17: No one under 17 and under admitted\n"; break; } } int main() { //create object of Movie Movie first; cout << "\nEnter name of Movie "; //read name of movie cin >> first.name; cout << "\nEnter Rating of movie from the following\n"; cout << "\n1 - G\n2 - PG\n3 - PG-13\n4 - R\n5 - NC-17\n\n"; //read rating of movie read_rating(first); cout << "\nEnter year produced "; //read year produced of movie cin >> first.year_produced; cout << "\nEnter main star of movie "; //read main star of movie cin >> first.main_star; cout << "\nPress Y if you saw the movie else Press N if you didn't saw the movie "; //read if user has saw the movie cin >> first.saw; cout << "\nPress Y if you liked the movie else Press N if you didn't liked the movie "; //read if user liked the movie cin >> first.liked; //print information about movie cout << "\n\nName of movie is " << first.name << endl; cout << "\nThe movie is produced in year: " << first.year_produced << endl; cout << "\nMain star of Movie is " << first.main_star << endl; display_rating(first.rating); if (first.saw == 'Y' || first.saw == 'y') cout << "\nYou saw the movie\n"; else cout << "\nYou did not see the movie\n"; if (first.liked == 'Y' || first.liked == 'y') cout << "\nYou liked the movie\n"; else cout << "\nYou did not like the movie\n"; cout << endl; } |

- Create a function INPUT which takes a structure variable as parameter.
- Pass structure variable using call by reference.
Complete INPUT function:
// Function definition for INPUT
void INPUT(Movie &first)
{
cout << " Enter name of Movie ";
//read name of movie
cin >> first.name;
cout << " Enter Rating of movie from the following ";
cout << " 1 - G 2 - PG 3 - PG-13 4 - R 5 - NC-17 ";
//read rating of movie
read_rating(first);
cout << " Enter year produced ";
//read year produced of movie
cin >> first.year_produced;
cout << " Enter main star of movie ";
//read main star of movie
cin >> first.main_star;
cout << " Press Y if you saw the movie else Press N if you didn't saw the movie ";
//read if user has saw the movie
cin >> first.saw;
cout << " Press Y if you liked the movie else Press N if you didn't liked the movie ";
//read if user liked the movie
cin >> first.liked;
}
- Create a function OUTPUT which takes structure variable as parameter.
- Pass structure variable using call by value.
Complete OUTPUT function:
/Function definition for OUTPUT
void OUTPUT(Movie first)
{
//print information about movie
cout << " Name of movie is " << first.name << endl;
cout << " The movie is produced in year: " << first.year_produced << endl;
cout << " Main star of Movie is " << first.main_star << endl;
display_rating(first.rating);
if (first.saw == 'Y' || first.saw == 'y')
cout << " You saw the movie ";
else
cout << " You did not see the movie ";
if (first.liked == 'Y' || first.liked == 'y')
cout << " You liked the movie ";
else
cout << " You did not like the movie ";
cout << endl;
}
Step by step
Solved in 4 steps with 1 images

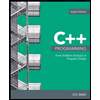
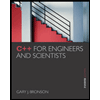
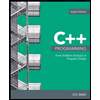
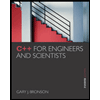