Using the structure below, write the C++ codes to enter data into each field and display the output: struct Person { char name[15]; int age; float salary; };
Using the structure below, write the C++ codes to enter data into each field and display the output:
struct Person
{
char name[15];
int age;
float salary;
};

The below given C++ program will obey the following rubrics:
- Importing essential header files.
- Declaring structure name person with attributes name, age and salary.
- In the main method, displaying message to user to enter the name.
- Reading input of name from user.
- Displaying message to user to enter age.
- Reading input of age from user.
- Displaying message to user to enter salary.
- Reading input of salary from user.
- After taking all the inputs from user displaying all the entered data to the user.
Program code:
//importing neccessary header files
#include <iostream>
//using namespace standard
using namespace std;
//defining structure name person
struct Person
{
//declaring name with char data type
char name[15];
//declaring age with integer data type
int age;
//declaring salary with float data type
float salary;
};
//main method
int main()
{
//creating object of person p1
Person p1;
//displaying message to user to enter name
cout << "Enter Full name: ";
// reading input of name from user
cin.get(p1.name, 15);
//displaying message to user to enter age
cout << "Enter age: ";
//reading input of age from user
cin >> p1.age;
//displaying message to user to enter salary
cout << "Enter salary: ";
//reading input of salary
cin >> p1.salary;
//displaying message to user
cout << " Displaying Information." << endl;
//displaying name to user
cout << "Name: " << p1.name << endl;
//displaying age to user
cout <<"Age: " << p1.age << endl;
//displaying salary to user
cout << "Salary: " << p1.salary;
return 0;
}//end of main method
Step by step
Solved in 3 steps with 1 images

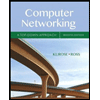
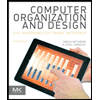
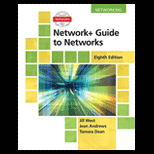
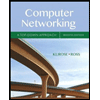
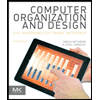
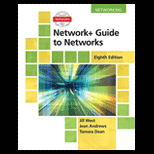
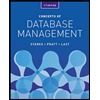
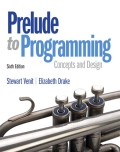
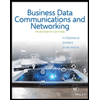