using this c++ code, can u change it a little bit into printing simulating. instead num_minutes 20, it should be change to only 10 person to serve. #include #include #include #include #define NUM_MINUTES 20 #define MIN_MINUTES_PER_ARRIVAL 1 #define MAX_MINUTES_PER_ARRIVAL 4 #define MIN_MINUTES_PER_SERVICE 1 #define MAX_MINUTES_PER_SERVICE 4 static int customerArrivalTimes[NUM_MINUTES]; int main() {
using this c++ code, can u change it a little bit into printing simulating. instead num_minutes 20, it should be change to only 10 person to serve.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <assert.h>
#define NUM_MINUTES 20
#define MIN_MINUTES_PER_ARRIVAL 1
#define MAX_MINUTES_PER_ARRIVAL 4
#define MIN_MINUTES_PER_SERVICE 1
#define MAX_MINUTES_PER_SERVICE 4
static int customerArrivalTimes[NUM_MINUTES];
int main()
{
// This will seed the random number generator.
unsigned int seed = (unsigned)time( NULL );
printf( "seeding with %u\n", seed );
srand( seed );
int nextArrival;
// The value of -1 is reserved to indicate that no customer is currently being serviced.
int nextService = -1;
int longestWait = 0;
int longestQueue = 0;
int queueLength = 0;
int queueStart = 0;
int queueEnd = 0;
// This will use the random number generator to set the arrival time of the first customer.
nextArrival = rand() % (MAX_MINUTES_PER_ARRIVAL + 1 - MIN_MINUTES_PER_ARRIVAL) + MIN_MINUTES_PER_ARRIVAL;
// This simulation will start at minute 0 and end at minute NUM_MINUTES - 1
for ( int i = 0; i < NUM_MINUTES; i++ )
{
//check if the next customer is scheduled to arrive in this minute
if ( nextArrival == i )
{
//update queueLength and test if record has been broken
queueLength++;
if ( queueLength > longestQueue ) longestQueue = queueLength;
//print information message
printf( "%03d:New customer arriving, queue length now: %d.\n", i, queueLength );
//remember the arrival time of the current customer
customerArrivalTimes[queueEnd++] = i;
//set random arrival time for next customer
nextArrival = rand() % (MAX_MINUTES_PER_ARRIVAL + 1 - MIN_MINUTES_PER_ARRIVAL) + MIN_MINUTES_PER_ARRIVAL + i;
//if queue was empty, start servicing the new customer
if ( queueLength == 1 )
{
// The following line will abort the program with an error
// message if nextService is a valid time value (not -1).
// This should not happen if the queue was empty. Therefore,
// this would indicate a bug in the program.
assert( nextService == -1 );
//schedule completion time of next customer service
nextService = rand() % (MAX_MINUTES_PER_SERVICE + 1 - MIN_MINUTES_PER_SERVICE) + MIN_MINUTES_PER_SERVICE + i;
}
}
//check if customer service is scheduled to finish in this minute
if ( nextService == i )
{
//print information message
printf(
"%03d:Customer service completed, total time: %d.\n",
i, i - customerArrivalTimes[queueStart]
);
// This code block will update the variable longestWait if the
// record was broken
if ( longestWait < i - customerArrivalTimes[queueStart] )
{
longestWait = i - customerArrivalTimes[queueStart];
}
//update the starting point and length of the queue
queueStart++;
queueLength--;
// The following line will abort the program with an error message
// if queueLength ever becomes negative (which should never happen
// and would indicate a bug in the program).
assert( queueLength >= 0 );
//if queue is not empty, start servicing the next customer
if ( queueLength != 0 )
{
//schedule completion time of next customer service
nextService = rand() % (MAX_MINUTES_PER_SERVICE + 1 - MIN_MINUTES_PER_SERVICE) + MIN_MINUTES_PER_SERVICE + i;
}
//otherwise indicate that no customer is currently being serviced
else
{
nextService = -1;
}
}
}
printf( "Longest wait: %d\n", longestWait );
printf( "Longest queue: %d\n", longestQueue );
return 0;
}

Step by step
Solved in 2 steps

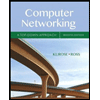
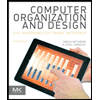
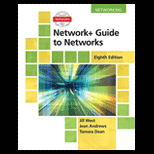
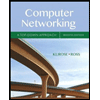
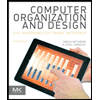
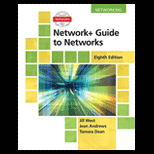
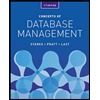
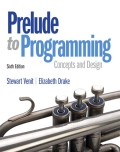
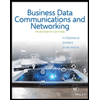