Using Value and Reference Parameters In Visual Studio Code under labactivity8_1 folder, create a new file main.cpp. Open the C++ source file main.cpp in the text editor and copy the source code below. /** * @file WRITE FILE NAME * @author WRITE STUDENT NAME(S) * @brief Using value and reference parameters. This program * uses a function to swap the values in two variables. * @date WRITE DATE TODAY * */ #include using namespace std; // Function prototype void swapNums(int number1, int number2); int main() { int num1 = 5, num2 = 7; // Print the two variable values cout << "In main the two numbers are " << num1 << " and " << num2 << endl; // Call a function to swap the values stored // in the two variables swapNums(num1, num2); // Print the same two variable values again cout << "Back in main again the two numbers are " << num1 << " and " << num2 << endl; return 0; } /** * @brief WRITE DESCRIPTION OF THE FUNCTION * * @param number1 DESCRIPTION * @param number2 DESCRIPTION */ void swapNums(int number1, int number2) { // Parameter a receives num1 and parameter b receives num2 // Swap the values that came into parameters a and b int temp = number1; number1 = number2; number2 = temp; // Print the swapped values cout << "In swapNums, after swapping, the two numbers are " << number1 << " and " << number2 << endl; } Update the header comment with the correct information. Read the source code, paying special attention to the swapNums parameters. When the program is run do you think it will correctly swap the two numbers? Compile and run the program to find out. Explain what happened. It swapped the numbers in the function but did not return any values so the numbers won’t get swapped in main.
Lab 8.1 - Using Value and Reference Parameters
-
In Visual Studio Code under labactivity8_1 folder, create a new file main.cpp. Open the C++ source file main.cpp in the text editor and copy the source code below.
/** * @file WRITE FILE NAME * @author WRITE STUDENT NAME(S) * @brief Using value and reference parameters. Thisprogram * uses a function to swap the values in two variables. * @date WRITE DATE TODAY * */ #include <iostream> using namespace std; // Function prototype void swapNums(int number1, int number2); int main() { int num1 = 5, num2 = 7; // Print the two variable values cout << "In main the two numbers are " << num1 << " and " << num2 << endl; // Call a function to swap the values stored // in the two variables swapNums(num1, num2); // Print the same two variable values again cout << "Back in main again the two numbers are " << num1 << " and " << num2 << endl; return 0; } /** * @brief WRITE DESCRIPTION OF THE FUNCTION * * @param number1 DESCRIPTION * @param number2 DESCRIPTION */ void swapNums(int number1, int number2) { // Parameter a receives num1 and parameter b receives num2 // Swap the values that came into parameters a and b int temp = number1; number1 = number2; number2 = temp; // Print the swapped values cout << "In swapNums, after swapping, the two numbers are " << number1 << " and " << number2 << endl; } -
Update the header comment with the correct information.
-
Read the source code, paying special attention to the swapNums parameters. When the program is run do you think it will correctly swap the two numbers? Compile and run the program to find out.
Explain what happened. It swapped the numbers in the function but did not return any values so the numbers won’t get swapped in main.
-
Change the two swapNums parameters to be reference variables. Section 6.13 of your text shows how to do this. You will need to make the change on both the function header and the function prototype. Nothing will need to change in the function call. After making this change, recompile and rerun the program. If you have done this correctly, you should get the following output.
In main the two numbers are 5 and 7 In swapNums, after swapping, the two numbers are 7 and 5 Back in main again the two numbers are 7 and 5Explain what happened this time. The function was passed references to the variables in main. This allows the function to change the contents of the variables in main as it is working on the same variable address in memory.
-
Update the function header comments of swapNums.
-
Commit your code with a message "lab 8.1 completed". Recall the terminal commands:
-
- git add -A
- git commit -m "COMMIT MESSAGE"
-
- Push your code back to the GitHub repo then continue with Lab 8.2.
Lab 8.2 - Three File Structure 1
-
We will keep working with Lab 8.1 program. Create a new file called functions.h. Inside this file, you will have to write the guard code, to ensure the code inside this file is only included once. The guard code is demonstrated below. Copy the code below and paste it inside functions.h.
/** * @file functions.h * @author WRITE STUDENT NAME(S) * @brief Declaration file * @date WRITE DATE TODAY * */ #ifndef FUNCTIONS_H // GUARD CODE #define FUNCTIONS_H // GUARD CODE // INCLUDE THE HEADER FILES NEEDED FOR THE FUNCTIONS TO WORK using namespace std; // PUT FUNCTION PROTOTYPES HERE #endif // GUARD CODE -
Update the header comment with the correct information.
-
From main.cpp, copy and remove the function prototypes, then paste the function prototypes in functions.h.
-
Back in main.cpp, just above using namespace std, you now need to include functions.h. Observe the code below.
#include <iostream> #include "functions.h" using namespace std; -
Create another new file called functions.cpp. This file is where the function definitions are going to be placed. Note, that the first line of code in this file is to include functions.h.
/** * @file functions.cpp * @author WRITE STUDENT NAME(S) * @brief Implementation/Definition file * @date WRITE DATE TODAY * */ #include "functions.h" // PUT FUNCTION DEFINTIONS HERE -
Update the header comment with the correct information.
-
From main.cpp, copy and remove the function definitions, then paste the function definitions in functions.cpp. Observe that you are using cout inside the function, which means you need to include iostream. The right place to include iostream will be inside functions.h.
-
Compile the program. For the executable named Lab8_2 see command below
- Use the compile command g++ -Wall lab8_1.cpp functions.cpp -o Lab8_2
-
Run the program. The program should give you the same result as in Lab 8.1. Understand how this code organization could help in the maintainability of your code. You will see the importance of this type of code organization when you have lots of functions in your C++ project.
-
Commit your code with the message "lab 8.2 completed".

Step by step
Solved in 3 steps

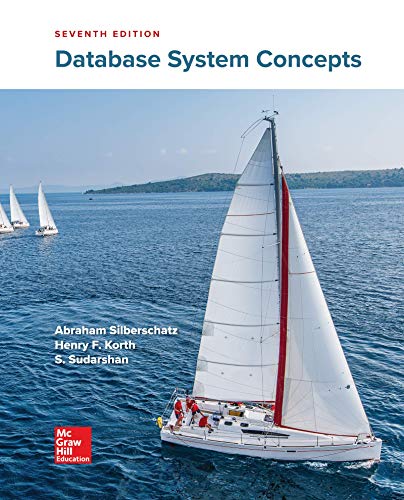
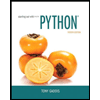
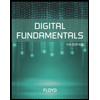
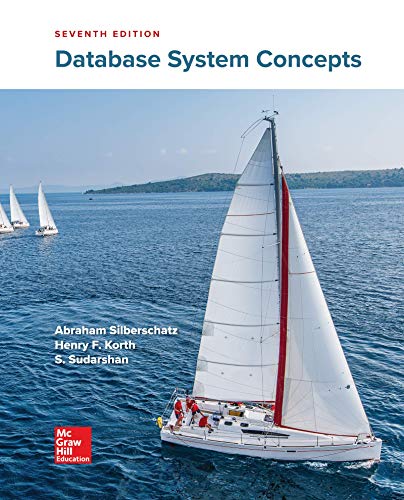
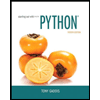
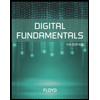
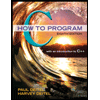
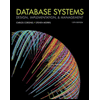
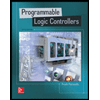