#!/usr/bin/env python3 Suppose we have very large sparse vectors, which contains a lot zeros and double. find a data structure to store them get the dot product of them def vector_to_index_value_list(vector): return [(i, v) for i,v in enumerate(vector) if v != 0.0]
#!/usr/bin/env python3 Suppose we have very large sparse vectors, which contains a lot zeros and double. find a data structure to store them get the dot product of them def vector_to_index_value_list(vector): return [(i, v) for i,v in enumerate(vector) if v != 0.0]
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![#!/usr/bin/env python 3
Suppose we have very large sparse vectors, which contains a lot of
zeros and double.
find a data structure to store them
get the dot product of them
def vector_to_index_value_list(vector):
return [(i, v) for i,v in enumerate(vector) if v != 0.0]
def dot product (iv_list1, iv_list2):
product = 0
p1=len (iv_list1)-1
p2 = len (iv_list2) - 1
while p1 >= 0 and p2 >= 0:
i1, v1 = iv_list1 [p1]
i2, v2 = iv_list2 [p2]
if i1 <i2:
p1=1
elif i2 <i1:
p2 = 1
else:
product+=v1 *v2
p1=1
p2 -= 1
return product
def__test_simple():
print(dot_product(vector_to_index_value_list([1., 2., 3.]),
vector_to_index_value_list([0., 2., 2.))))
# 10
def__test_time():
vector_length = 10
vector_count=1024
nozero_counut = 10
def random_vector():
import random
vector = [0 for _ in range(vector_length)]
for i in random.sample(range(vector_length), nozero_counut):
vector[i] = random.random()
return vector
vectors = [random_vector() for in range(vector_count)]
iv_lists = [vector_to_index_value_list(vector) for vector in vectors]
import time
time_start = time.time()
for i in range(vector_count):
for j in range(i):
dot_product(iv_lists[i], iv_lists[i])
time_end = time.time()
print(time_end - time_start, 'seconds')
if __name__ == '___main__':
_test_simple()
__test_time()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3e38f750-a3b6-41fa-9e5a-77c8be67512c%2Fb2c78341-8e01-4fae-a4be-5b4557afcef1%2Fsm8j3l5_processed.jpeg&w=3840&q=75)
Transcribed Image Text:#!/usr/bin/env python 3
Suppose we have very large sparse vectors, which contains a lot of
zeros and double.
find a data structure to store them
get the dot product of them
def vector_to_index_value_list(vector):
return [(i, v) for i,v in enumerate(vector) if v != 0.0]
def dot product (iv_list1, iv_list2):
product = 0
p1=len (iv_list1)-1
p2 = len (iv_list2) - 1
while p1 >= 0 and p2 >= 0:
i1, v1 = iv_list1 [p1]
i2, v2 = iv_list2 [p2]
if i1 <i2:
p1=1
elif i2 <i1:
p2 = 1
else:
product+=v1 *v2
p1=1
p2 -= 1
return product
def__test_simple():
print(dot_product(vector_to_index_value_list([1., 2., 3.]),
vector_to_index_value_list([0., 2., 2.))))
# 10
def__test_time():
vector_length = 10
vector_count=1024
nozero_counut = 10
def random_vector():
import random
vector = [0 for _ in range(vector_length)]
for i in random.sample(range(vector_length), nozero_counut):
vector[i] = random.random()
return vector
vectors = [random_vector() for in range(vector_count)]
iv_lists = [vector_to_index_value_list(vector) for vector in vectors]
import time
time_start = time.time()
for i in range(vector_count):
for j in range(i):
dot_product(iv_lists[i], iv_lists[i])
time_end = time.time()
print(time_end - time_start, 'seconds')
if __name__ == '___main__':
_test_simple()
__test_time()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
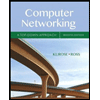
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
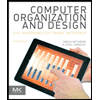
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
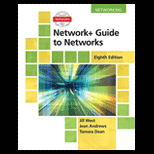
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
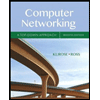
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
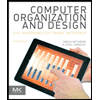
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
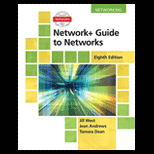
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
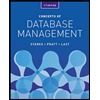
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
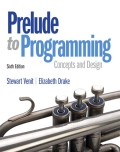
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
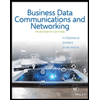
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY