We will be working on a project that designs a class calendarType, so that a client program can use this class to print a calendar for any month starting Jan 1. 1900. An example of the calendar for September 2019 is: September 2019 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 We will develop several classes which will work together to create a calendar. The first of these classes is called dayType which will manipulate a day of the week. The class dayType will store a day, such as Sun for Sunday. The class should be able to perform the following operations on an object of type dayType: a. Set the day. b. Print the day. c. Return the day. d. Return the next day. e. Return the previous day. f. Calculate and return the day by adding certain days to the current day. For example, if the current day is Monday and we add 4 days, the day to be returned is Friday. Similarly, if today is Tuesday and we add 13 days, the day to be returned is Monday. g. Include the appropriate constructors. the UML diagram for the dayType class looks like this:
dayType Class
We will be working on a project that designs a class calendarType, so that a client program can use this class to print a calendar for any month starting Jan 1. 1900.
An example of the calendar for September 2019 is:
September 2019
Sun Mon Tue Wed Thu Fri Sat
1 2 3 4 5 6 7
8 9 10 11 12 13 14
15 16 17 18 19 20 21
22 23 24 25 26 27 28
29 30 31
We will develop several classes which will work together to create a calendar. The first of these classes is called dayType which will manipulate a day of the week. The class dayType will store a day, such as Sun for Sunday. The class should be able to perform the following operations on an object of type dayType:
a. Set the day.
b. Print the day.
c. Return the day.
d. Return the next day.
e. Return the previous day.
f. Calculate and return the day by adding certain days to the current day. For example, if the current day is Monday and we add 4 days, the day to be returned is Friday. Similarly, if today is Tuesday and we add 13 days, the day to be returned is Monday.
g. Include the appropriate constructors.
the UML diagram for the dayType class looks like this:
dayType |
-weekDay: string -daaysOfWeek[7]:string |
+print():void +nextDay():string +prevDay():string +addDay(int nDays):void +setDay(string d):void +getDay():string +dayType(string=“Sunday”) |
The class has a static array to hold the names of the days of the week. Non-static class features (attributes or operations) belong to individual instances of the class (i.e., to an object). Static features belong to the class. Unless otherwise specified, features in a UML class diagram are non-static. The UML diagram denotes static features by underlining the feature.
The constructor uses default parameters so it can serve as the default constructor or as a constructor with parameters.
The header file has been supplied for you. Note the comments in the header file describing the preconditions and post condition for the member functions.
The implementation file is also included and shows how to initialize the static array. You are to complete the implementation file and write a client program to test the class. The client program should do the following:
1. Create a day object using the default constructor and use the print function to display the instance variable. The output should be “Sunday”.
2. Call prevDay() and print. The output should be “Saturday”.
3. Call nextDay() and print. The output should be “Monday”.
4. Create a day object using the constructor with parameters and initialize the object to “Monday”.
5. Use getDay() to display the value of the instance variable (should be “Monday”).
6. Using the second object, call addDays(3) and display the return value (should be “Thursday”).
7. . Use the second object to call addDays(5) and display the return value (should be “Tuesday”).
Label your output so that the user (i.e., me, your instructor) can tell what your program is doing. This client program will serve as your test to ensure that the class is working as it is supposed to. Here is an example of the labeled output:
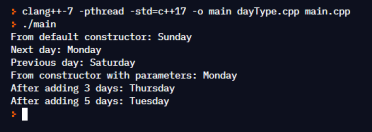

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

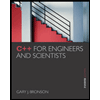
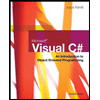
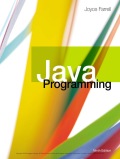
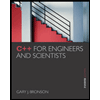
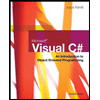
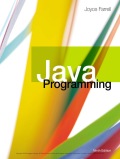