We will consider the Exam class seen in the Problem of the Lab 02. You are asked to define two more classes that inherits from the Exam class which are MidtermExam and LabExam, and, optionally, you can define Final Exam class. You have the freedom to make the design choices, but we would like to provide you some guidelines to simplify some aspects of your work. You can define a new class named DateTime that will include all members from Exam class concerning the date and time. Then, by composition, link the Exam with DateTime. Move all members specific to a subclass from the superclass to the subclass and keep the more general in the superclass. Move the specific processing from the constructor of the superclass to the constructor of the subclass. If a method performs different processing, which is related to superclass and subclass, redefine (override) the method in the subclass with the specific processing, and keep only the general processing in the superclass's method. Invoke the superclass constructor and methods whenever you need them in subclass. Keep the superclass members with their original access specifiers, unless you have wisely decided to upgrade their visibility. Test all the classes.
We will consider the Exam class seen in the Problem of the Lab 02. You are asked to define two more classes that inherits from the Exam class which are MidtermExam and LabExam, and, optionally, you can define Final Exam class. You have the freedom to make the design choices, but we would like to provide you some guidelines to simplify some aspects of your work. You can define a new class named DateTime that will include all members from Exam class concerning the date and time. Then, by composition, link the Exam with DateTime. Move all members specific to a subclass from the superclass to the subclass and keep the more general in the superclass. Move the specific processing from the constructor of the superclass to the constructor of the subclass. If a method performs different processing, which is related to superclass and subclass, redefine (override) the method in the subclass with the specific processing, and keep only the general processing in the superclass's method. Invoke the superclass constructor and methods whenever you need them in subclass. Keep the superclass members with their original access specifiers, unless you have wisely decided to upgrade their visibility. Test all the classes.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
This is the Exam class mentioned
public class Exam
{
private int day;
private int month;
private int year;
private int hour;
private int minutes;
private double maxMarks;
private final String type;
private final int order;
private static int labExamCounter = 0;
private static int midtermExamCounter = 0;
public static final String LAB_EXAM = "Lab Exam";
public static final String MIDTERM_EXAM = "Midterm Exam";
public static final String FINAL_EXAM = "Final Exam";
public Exam(String type)
{
this (type, 0, 0, 0, 0, 0, 0);
}
public Exam(String type, double maxMarks)
{
this (type, maxMarks, 0, 0, 0, 0, 0);
}
public Exam(String type, double maxMarks, int day, int month, int year)
{
this (type, maxMarks, day, month, year, 0, 0);
}
public Exam(Exam other)
{
this (other.getType(), other.getMaxMarks(), other.getDay(), other.getMonth(), other.getYear(), other.getHour(), other.getMinutes());
}
public Exam(String type, double maxMarks, int day, int month, int year, int hour, int minutes)
{
switch( type ) {
caseLAB_EXAM:
this.order = ++labExamCounter;
this.maxMarks = (maxMarks > 5 && maxMarks <= 10 ? maxMarks : 10 );
break;
caseMIDTERM_EXAM:
this.order = ++midtermExamCounter;
this.maxMarks = (maxMarks > 15 && maxMarks <= 20 ? maxMarks : 20 );
break;
case FINAL_EXAM:
this.order = 0;
this.maxMarks = (maxMarks > 30 && maxMarks <= 40 ? maxMarks : 40 );
break;
default:
this.order = 0;
this.maxMarks = maxMarks;
}
if (day >= 1 && day <= 31)
this.day = day;
if (month >= 1 && month <= 12)
this.month = month;
if (year > 0)
this.year = year;
if (hour >= 0 && hour <= 23)
this.hour = hour;
if (minutes >= 0 && minutes <= 59)
this.minutes = minutes;
this.type = (type != null ? type : "");
}
public String getType()
{
return type;
}
public void setDay(int day)
{
if (day >= 1 && day <= 31)
this.day = day;
}
public int getDay()
{
return day;
}
public void setMonth(int month)
{
if (month >= 1 && month <= 12)
this.month = month;
}
public int getMonth()
{
return month;
}
public void setYear(int year)
{
if (year > 0)
this.year = year;
}
public int getYear()
{
return year;
}
public void setDate(int day, int month, int year)
{
this.setDay(day);
this.setMonth(month);
this.setYear(year);
}
public void setHour(int hour)
{
if (hour >= 0 && hour <= 23)
this.hour = hour;
}
public int getHour()
{
return hour;
}
public void setMinutes(int minutes)
{
if (minutes >= 0 && minutes <= 59)
this.minutes = minutes;
}
public int getMinutes()
{
return minutes;
}
public void setTime(int hour, int minutes)
{
this.setHour(hour);
this.setMinutes(minutes);
}
public void setMaxMarks(double maxMarks)
{
switch( this.type ) {
caseLAB_EXAM:
this.maxMarks = (maxMarks > 5 && maxMarks <= 10 ? maxMarks : 10 );
break;
caseMIDTERM_EXAM:
this.maxMarks = (maxMarks > 15 && maxMarks <= 20 ? maxMarks : 20 );
break;
case FINAL_EXAM:
this.maxMarks = (maxMarks > 30 && maxMarks <= 40 ? maxMarks : 40 );
break;
}
}
public double getMaxMarks()
{
return this.maxMarks;
}
public String toString()
{
return String.format("Exam: %s %s (%04.2f marks)\nDate: %d/%d/%d\nTime: %02d:%02d\n",
getType(), (order > 0 ? order : ""), getMaxMarks(), getDay(),
getMonth(), getYear(), getHour(), getMinutes());
}
public static String getExamStats()
{
return LAB_EXAM + ": " + labExamCounter + " exam(s)\n" +
MIDTERM_EXAM + ": " + midtermExamCounter + " exam(s)\n" ;
}
}
and this is the examtest fileimport static java.lang.System.out;
public class ExamTest
{
public static void main(String[] args)
{
Exam exam1 = new Exam(Exam.FINAL_EXAM);
exam1.setDate(13, 8, 1439);
exam1.setTime(8,0);
exam1.setMaxMarks(50);
Exam exam2 = new Exam(Exam.MIDTERM_EXAM, 10);
exam2.setDate(3, 7, 1439);
exam2.setTime(8,30);
Exam exam3 = new Exam(Exam.LAB_EXAM, 12, 18, 6, 1439);
exam3.setTime(8,30);
Exam exam4 = new Exam("Lab Quiz", 2, 28, 5, 1439, 10, 30);
Exam exam5 = new Exam(exam3);
exam5.setDate(10, 7, 1439);
out.println( "exam1:\n" + exam1.toString() );
out.println( "exam2:\n" etc.....}
can it be done in a way of classes as the question asked?
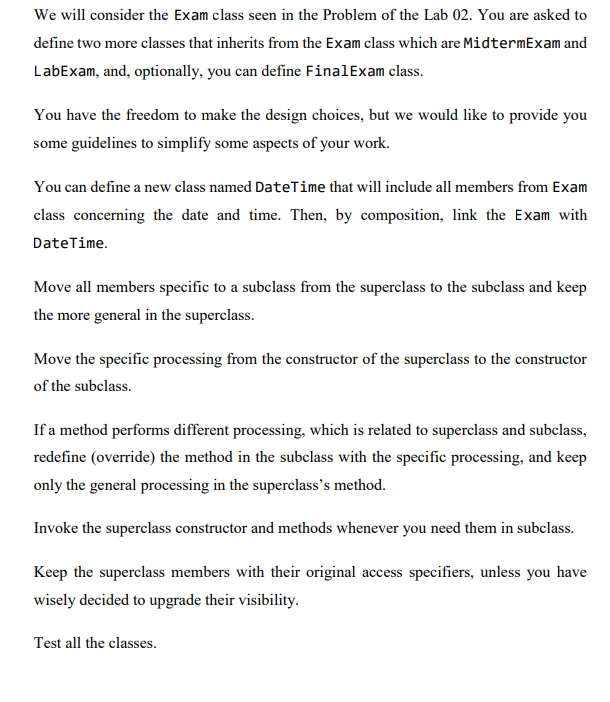
Transcribed Image Text:We will consider the Exam class seen in the Problem of the Lab 02. You are asked to
define two more classes that inherits from the Exam class which are MidtermExam and
LabExam, and, optionally, you can define Final Exam class.
You have the freedom to make the design choices, but we would like to provide you
some guidelines to simplify some aspects of your work.
You can define a new class named DateTime that will include all members from Exam
class concerning the date and time. Then, by composition, link the Exam with
DateTime.
Move all members specific to a subclass from the superclass to the subclass and keep
the more general in the superclass.
Move the specific processing from the constructor of the superclass to the constructor
of the subclass.
If a method performs different processing, which is related to superclass and subclass,
redefine (override) the method in the subclass with the specific processing, and keep
only the general processing in the superclass's method.
Invoke the superclass constructor and methods whenever you need them in subclass.
Keep the superclass members with their original access specifiers, unless you have
wisely decided to upgrade their visibility.
Test all the classes.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
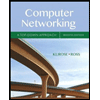
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
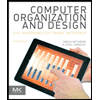
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
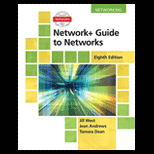
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
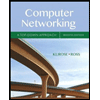
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
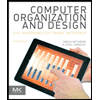
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
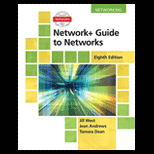
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
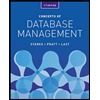
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
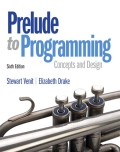
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
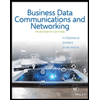
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY