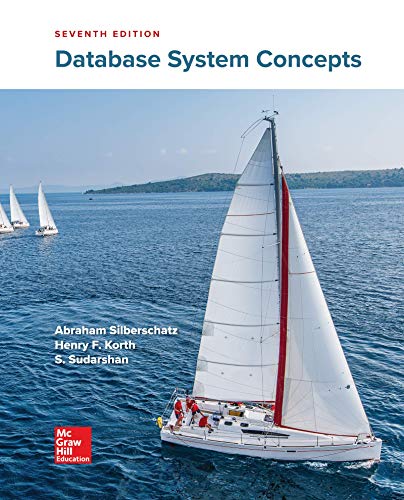
Why won't this line compile?
Main.cpp
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
#include <sstream>
#include "card.h"
#include "deck.h"
#include "hand.h"
using namespace std;
int main()
{
string repeat = "Y";
Deck myDeck;
Hand myHand;
string exchangeCards;
while (repeat == "Y" || repeat == "y")
{
cout << endl;
myHand.newHand(myDeck);
myHand.print();
cout << endl;
cout << "Would you like to exchange any cards? [Y / N]: ";
getline(cin, exchangeCards);
while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, exchangeCards);
}
if(exchangeCards == "Y" || exchangeCards == "y")
{
myHand.exchangeCards(myDeck);
}
cout << endl;
myHand.print();
cout << endl;
myDeck.reset(); // Resets the deck for a new game
cout << "Play again? [Y / N]: ";
getline(cin, repeat);
while (repeat != "Y" && repeat != "y" && repeat != "N" && repeat != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, repeat);
}
}
return 0;
}
Deck.h
#ifndef DECK_H
#define DECK_H
#include <vector>
#include <cstdlib> // srand(), rand()
#include <ctime> // time()
#include "card.h" // Include card header file here
using namespace std;
class Deck
{
public:
Deck();
void resetDeck();
void printUndealtDeck();
void printDealtDeck();
const int getSizeUndealtDeck();
const int getSizeDealtDeck();
Card dealCard(); // Is the dealCard() here an accessor or mutator function???
private:
vector<Card> m_undealtDeck; // Undealt cards
vector<Card> m_dealtDeck; // Dealt cards
};
#endif
hand.h:
#ifndef HAND_H
#define HAND_H
#include <iostream>
#include "card.h" // Include card header file here
#include "deck.h" // Include deck header file here
using namespace std;
const int NUM_CARDS_ON_HAND = 5;
class Hand
{
public:
void newCard(Deck& deck, int location);
void newHand(Deck& deck);
void exchangeCards(Deck& deck);
void print();
private:
Card m_hand[NUM_CARDS_ON_HAND]; // A hand consists of 5 cards
};
#endif
card.h:
#ifndef CARD_H
#define CARD_H
#include <iostream>
#include <string>
using namespace std;
const string pips[] = {"Ace", "Two", "Three", "Four", "Five",
"Six", "Seven", "Eight", "Nine", "Ten",
"Jack", "Queen", "King"};
const string suits[] = {"Hearts", "Spades", "Clubs", "Diamonds"};
class Card
{public:
int get();
void set(int value);
string getPip();
string getSuit();
void print();
private:
int m_cardValue;
};
#endif
Deck.cpp:
#include "deck.h"
Deck::Deck() //--- default class constructor
{ srand(time(0));
for (int i=0; i < 52; i++)
{Card cardInstance;
cardInstance.set(i)
m_undealtDeck.push_back(cardInstance); // should it be .get here()???
}
}
void Deck::reset()
{ while (getSizeDealtDeck() != 0)
{
int last_index = getSizeDealtDeck()-1;
m_undealtDeck.push_back(m_dealtDeck[last_index]);
m_dealtDeck.pop_back();
}
}
Card Deck::dealCard() // --- Deal a single card
{ int random_index = rand() % getSizeUndealtDeck(); // gets random index in undealt deck
Card random_card; // creates a card instance
random_card.set(m_undealtDeck[random_index].get()); // set the random card to the correct value
m_dealtDeck.push_back(random_card); // pushes the random card to the dealt deck
m_undealtDeck[random_index] = m_undealtDeck.back(); // puts the back index value to the random index
m_undealtDeck.pop_back(); // gets rid of the back value
return random_card;
const int Deck::getSizeUndealtDeck() // --- return size of undealt deck
{ return m_undealtDeck.size();
}
const int Deck::getSizeDealtDeck() // --- return size of dealt deck
{ return m_dealtDeck.size();
}
void Deck::printUndealtDeck() // loops through the undealt deck and prints it
{
for (int i=0; i < getSizeUndealtDeck(); i++)
{
m_undealtDeck[i].print();
cout << endl;
}
}
void Deck::printDealtDeck() // loops through the dealt deck
{
for (int i=0; i < getSizeDealtDeck(); i++)
{
m_dealtDeck[i].print();
cout << endl;
}
}
Hand.cpp
#include "pokerHand.h"
void Hand::newCard(Deck& deck, int location)
{
Card new_card = deck.dealCard();
m_hand[location] = new_card;
}
void Hand::newHand(Deck& deck)
{
for (int i=0; i < NUM_CARDS_ON_HAND; i++)
{
Hand::newCard(deck, i);
}
}
void Hand::exchangeCards(Deck& deck)
{
int num_of_exchanges;
cout << "How many cards would you like to exchange (1-5): "; // gets number of cards to be exchanged
cin >> num_of_exchanges;
while (num_of_exchanges > 5 || num_of_exchanges < 1) // validates input
{
cout << "Please enter a number (1-5): ";
cin >> num_of_exchanges;
}
for (int i=0; i < num_of_exchanges; i++) // looping through number of exchanges
{
int position = i; // positioned to be changed is i
if (num_of_exchanges != NUM_CARDS_ON_HAND) // if number of exchanges is less than total hand count
{
cout << "Enter a position in hand of card to exchange: ";
cin >> position; // gets position to be replaced
while (position > 5 || position < 1) // validates input
{
cout << "Please enter a number (1-5): ";
cin >> position;
}
position--;
}
Hand::newCard(deck, position); // replaces card at position
}
}
void Hand::print()
{for (int i=0; i < NUM_CARDS_ON_HAND; i++)
{cout << "Card " << i+1 << " is the ";
m_hand[i].print();
cout << endl;
}}
Card.cpp:
![6:54
* 0 ©
main.cpp - Code:Blocks 20.03
Edit View Seagch Project Build Debug Fortran woSmith Jools Tools+ Plugins DoxyBlocks Settings Help
lobal>
v main(): int
****
S
t here × "deck.cpp x "hand.cpp x *main.cpp x "deck.h × "hand.h x "card.h x "card.cpp x
32
while (exchangeCards !- "y" &6 exchangeCards !- "y" Ss exchangeCards !- "X" 66 exchangeCards !- "n")
33
cout << "Please enter Y or N only: ":
getline (cin, exchangeCards) ;
34
35
36
37
if(exchangeCards == "Y" || exchangeCarda == "y")
38
39
myHand.exchangeCards (myDeck) :
40
41
cout << endl;
42
myHand.print () ;
cout < endl;
myDeck.reset ():
cout « "Play again? [Y / N]: ";
getline (cin, repeat):
while (repeat !- "Y" &G repeat !- "y" 4G repeat !- "N" GG repeat !- "n")
// Resets the deck for a new game
45
cout << "Please enter Y or N only: ";
getline (cin, repeat);
49
50
& others
Cccc X O Build log X
Build messages x CppCheck/Vera++ X CppCheck/Vera+• messages X Cscope X
O Debugger X
2 DoxyBlocks X Fortran info X
t Closed files list X
Thr
de
Line
Message
=== Build file: "no target" in "no project" (compiler: unknown) ===
In funetion 'int main()':
AUsers\Mica...
Users\Mica...
44
error: 'resetDeck' was not declared in this scope
- Build failed: 1 error (s), O varning (s) (0 minute (s), 2 second(s)) ---
sers\Micai\Documents\Assignment2\main.cpp
C/C++
Windows (CR-LF) WINDOWS-125s2 Line 45, Col 25, Pos 1199
Modified Read/Write default
Insert](https://content.bartleby.com/qna-images/question/e6b9d87e-6e1d-4edc-b4b6-dbb55c2a248d/74eed866-e8d6-4509-bd7b-8806294f7ec0/ulfu6o_thumbnail.png)
![6:52
* 0 ©
10
rd.cpp - Code:Blocks 20.03
dit View Seagch Project Build Debug Fortran wSmith Jools Tgols+ Pļugins DoxyBlocks Settings Help
get): void
** *<
S C
ere x "deck.cpp x "hand.cpp x "main.cpp X
"deck.h x "hand.h x "card.h x "card.cpp x
#include "card.h"
void Card::get ()
return m cardvalue;
void Card: :set (int newCardval)
_cardvalue - newCardVal;
string Card::getPip ()
return pips (m_cardValue 13];
string Card: :getSuit ()
return suitS (m_cardvalue / 131;
17
18
void Card: :print ()
int suit_number - m_cardValue / 13;
int pip_number = m_cardValue 13;
cout << pips (pip_number] « " of " « suita(suit_number);
21
22
23
24
Mica\Documents\Assignment2\card.cpp
C/C++
Windows (CR-LF) WINDOWS-1252 Line 5, Col 2, Pos 66
Modified Read/Write default
Insert](https://content.bartleby.com/qna-images/question/e6b9d87e-6e1d-4edc-b4b6-dbb55c2a248d/74eed866-e8d6-4509-bd7b-8806294f7ec0/clgqpj_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Find a line with an error in the following code segment: 1: void displayFile(fstream file) {2: string line;3: while (file >> line) 4: cout << line << endl;5: } Group of answer choices 1 2 3 4arrow_forwardA word Decrambling game using srand() for descrambling these words: "Screen, Programming, Command, Definition." The game should be timed for 1 minute or 30 seconds using the <ctime> library. Should allow for user input to be able to guess...arrow_forwarddemo.cpp #include "Poly.h" #include<iostream> using namespace std; int main() { Poly p1; couble d[3]={1,2,3}: Poly p2(3, d); Poly p3(p2); cout << "Polynomial p1 is: "; p1.display(); cout << "Polynomial p2 is: " << p2 << endl; cout << "Polynomial p3 is: " << p3 << endl; if (p2 == p3) { cout << "p2 and p3 are the same."; } else { cout << "p2 and p3 are different."; } cout << endl; cout << "The coefficient of power 1 of p3 is: " << p2.getCoef(1) << endl; p3.setCoef(1, 99); cout << "p3 after setup is: " << p3 << endl; if (p2 == p3) { cout << "p2 and p3 are the same."; } else { cout << "p2 and p3 are different."; } cout << endl; Poly p4; cout << "Please enter the power and coefficients of Polynomial p4: " << endl; cin >> p4; cout <<…arrow_forward
- task7.c Compare 2 strings function cmp will print out > if s1 is "bigger" than s2, < if s1 is "smaller than s2, = if s1 is "equal" to s2. */ #include <stdio.h> #include <string.h> void cmp(char *s1, char *s2){ return; } int main() { char *string1 = "UNIX rules!"; char *string2 = "Windows drools!"; cmp(string1,string2); return 0; } Start with task7.c, implement the cmp() function. The goal of this function is to compare two strings using the sum of the ASCII value of all the characters in each string. Output of your program should look like this: UNIX rules! is smaller than Windows drools!arrow_forwardAssign secretID with firstName, a space, and lastName. Ex: If firstName is Barry and lastName is Allen, then output is:Barry Allen #include <iostream>#include <string>using namespace std; int main() { string secretID; string firstName; string lastName; cin >> firstName; cin >> lastName; /* Your solution goes here */ cout << secretID << endl; return 0;}arrow_forwardI am trying to compile a poker game: #include <iostream>#include <string>#include <fstream>#include <iomanip>#include <sstream> #include "card.h"#include "deck.h"#include "hand.h" using namespace std; /************************************************************* FunctionName ** Function description ** ** *************************************************************/int main(){string repeat = "Y";Deck myDeck;Hand myHand;string exchangeCards; while (repeat == "Y" || repeat == "y"){cout << endl; myHand.newHand(myDeck);myHand.print();cout << endl; cout << "Would you like to exchange any cards? [Y / N]: ";getline(cin, exchangeCards); while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n"){cout << "Please enter Y or N only: ";getline(cin, exchangeCards); } if(exchangeCards = "Y" || exchangeCards = "y"){myHand.exchangeCards(myDeck);}cout << endl; myHand.print();…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
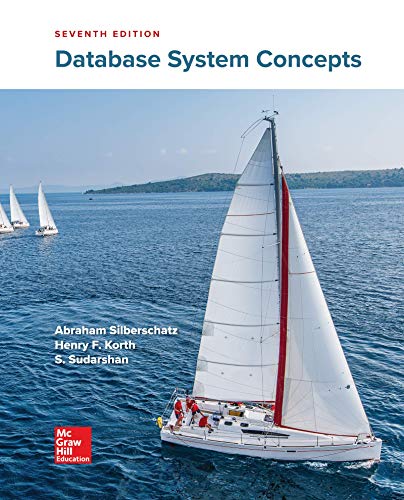
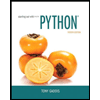
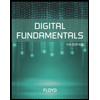
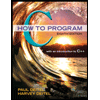
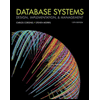
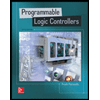