Write a C language function named count that takes a void pointer and int parameter, representing a memory buffer, and a uint8_t parameter representing a byte, and returns an int result. The returned result should be the number of occurrences of the byte in the bufer. For example if the buffer p pointed to the bytes 01 12 01 23, then count(p, 4, 0x01) should return 2.
Q: Consider the following function definition. void mystery(int* x) { // function body } (a) Inside the…
A: Let us see the answer below,
Q: Recreate the following C program in x86-64 and aarch64 assembly manually: #include typedef struct…
A: Below I have provided the assembly code:
Q: Write a program in c language to read multiple DNA sequences from user. Create non returning…
A: #include<stdio.h> int string_len(char*);void reversedna(char*); main() { char str[100];…
Q: Q2. Consider a valid double pointer char** mypointer in a 32-bit system. What is sizeof(*mypointer)?
A: First we should understand what is pointer . Consider that you had created the variable in the c…
Q: Write a C program to find the sum of all non-prime numbers from m and n (including m and n also)…
A: A number is said to be prime number if it has factors 1 and itself
Q: In C In C, strings are implemented by a contiguous sequence of characters, terminated by NUL…
A: A. A. Write your own version of stropy that receives two character pointers and copies the…
Q: int bof(char *str, int size) { char *buffer = (char *) malloc(size); strcpy(buffer, str);…
A:
Q: Write a C++ function, bool ok(int q[]), which takes an array q and returns true if the array…
A: Given: Write a C++ function, bool ok(int q[]), which takes an array q and returns true if the…
Q: Write a Menu Driven C++ program that creates one-dimensional array arr[] and initialize it with…
A: Actually, program is a executable software that runs on a computer.
Q: write a program in C language to count composite numbers from m and n (including m and n also) using…
A: #include<stdio.h>…
Q: Write a single and complete C program to achieve the following: a. Using pointer, take input of ten…
A: The program is written in C Language. Please check the source code and output in the following…
Q: Write a C++ program to read a size of an array (F) and then read the array elements from the user to…
A: Program I) #include <iostream>using namespace std; int main () { int i,F;…
Q: Recreate the following C program in x86-64 and aarch64 assembly manually: #include typedef struct…
A:
Q: ere function f returns the results through address passing. Assuming c is a global complex number…
A: Complex increase is a more troublesome activity to comprehend from either an arithmetical or a…
Q: Define a function in the C++ language that takes a string as the parameter. The value of the string…
A: Given: vector<string>users = {"Sammy", "Ray", "Ponel", "Walley"}; Requirement: Define a…
Q: Complete the missing code at ” ” to implement the function with the variables GPIO_PORT_P1,…
A: Solution:: Let's see the above question answer which is given fill in the blanks.…
Q: Write a Menu Driven C++ program that creates a character array/string by taking input from user and…
A: Program: #include <iostream> #include <string> #include<bits/stdc++.h> using…
Q: Write a C++ code for a function that creates a one-dimensional dynamic array to emulate a…
A: Answer: One-dimensional dynamic array to emulate a two-dimensional array And returns a pointer to…
Q: in c++ Given the string "An elephant." Store it in a char pointer Print on one line the letter on…
A: In step 2, you will get the C++ code. In step 3, you can see the output.
Q: Write a program to count Non-Armstrong numbers from m and n (including m and n also) using the…
A: /******************************************************************************…
Q: Write a program in C to show the basic declaration of pointer. Expected Output : Pointer : Show…
A: - We have to work with the declaration of pointers. - We have to work in c language.
Q: Write a complete C program consisting of a main and a function named MostFreq, which do the…
A: Code: #include <bits/stdc++.h>using namespace std; unordered_map<int, int> hm; void…
Q: Write a program in c languageto find the sum of all non-prime numbers from m and n (including m and…
A: Prime number: A number is said to be a prime number if the number of divisors of the numbers is…
Q: ion called genericSort() that takes in a numeric or integer vector, sorts it, and returns the…
A: We need to define the genericSort() to sort and return the sorted indices as per the given…
Q: Write a program in c that will read an arbitrary numbers of proverbs from the keyboard and store…
A: Purpose of the program: This program will read the number of proverbs required to sort and the…
Q: Write a C++ function that uses pointer notation only to swap the contents of two integer arrays…
A: Program approach:- // using header file. // using namespace function. // num1 pointer to array1 and…
Q: WRITE THE PROGRAM IN C LANGUAGE : Write a program to count Non-Armstrong numbers from m and n…
A: Program: //included header files #include<stdio.h> #include<math.h> //calculate…
Q: Write a program to find the sum of all non-prime numbers from m and n (including m and n also) using…
A: Please find the answer below :
Q: Write a C++ program that gets 5 numbers from the users into a 1D dynamic array. Sort the numbers…
A: Required: Write a C++ program that gets 5 numbers from the users into a 1D dynamic array. Sort the…
Q: 5.1 Accept student’s information into three parallel arrays. The capture of the students’ marks…
A: In the Given solution We are using Dynamic Array "Vector" Just because of in dynamic array here In…
Q: Write a program to find the number of times that a given word occurs in a sentence using pointers.…
A: Write a program to find the number of times that a given word occurs in a sentence using pointers.…
Q: Write a program to find the sum of all non-prime numbers from m and n (including m and n also) using…
A: A positive integer number that has only two factors, 1 and itself is called prime number. To check a…
Q: Write a program to find the sum of all non-prime numbers from m and n (including m and n also) using…
A: #include <stdio.h>int isPrime(int i){ if(i==1) { return 0; } else if(i==2)…
Q: In C++ Write a program that accepts a C-string input from the user and reverses the contents of the…
A: The below program accepts string from user and have 2 pointers head and tail. The program swap those…
Q: Write a single and complete C program to help faculty ‘X’ preparing grades of EEE 103: a. Ask the…
A: #include <stdio.h>#include <stdlib.h>#define maximum_mark 45int main(int argc, char…
Q: Write a program to find the number of times that a given word occurs in a sentence using pointers.…
A: Write a program to find the number of times that a given word occurs in a sentence using pointers.…
Q: Write a function in C language that will take a 2D Array of size 3x3 from the user. Your Program…
A: Here I have made a function named sum that will calculate row and column sum and then will return 0…
Q: Write a C program which is a function printArrayReverse(int* arr, int size) that uses a pointer to…
A: Answer : #include <stdio.h> #define MAX_SIZE 100 struct reverseArray { int…
Q: Write a function in C++ called maximumGap that finds the maximum difference between corresponding…
A: c code: #include<stdio.h> //header file to…
Q: Write a C++ program that adds two numbers of different numeric data types (e.g. integers, float,…
A: write a program for addition of diff numeric data types using overloaded function add() Function…
Q: Write a small library in the C programming language to do Minifloat (Links to an external site.)…
A: SUMMARY: - hence we discussed all the points.
Q: Write a function f which takes three pointers to int variables (a, b, c). Then it should: -Set the…
A: "Since you have asked multiple questions, we will solve the first question for you. If you want any…
Q: define a vector object and initialize it with 3 values and then define an iterator that points to…
A: Below i have written program:
Q: Write a C function arrDivN() that takes one array of integers and three integer numbers (say ar, n1,…
A: Input to the Program : Array = List of integers n1 and n2 are range numbers n3 - Checked for…
Q: Write a C program that uses the following: a main() to read two integer values from the user, val1…
A: The pointers in the C language are the data types that are used to store the values of memory…
Q: Given the following function in C++ language. i. 10 20 30 40 50 60 70 80 90 100 void ValveControl…
A: Program: #include <iostream> using namespace std; void valveControl(int pressure , int…
Q: Write a c++ code that swaps two input integers using pointers. a. In the main function. b. By…
A: As i have read guidelines i can provide answer of only 1 part of questions in case of multiple…
Q: Write a function whose prototype is void exchange(int *p, int *q);that takes two pointers to integer…
A: Write a function whose prototype is
Write a C language function named count that takes a void pointer and int parameter, representing a memory buffer, and a uint8_t parameter representing a byte, and returns an int result. The returned result should be the number of occurrences of the byte in the bufer. For example if the buffer p pointed to the bytes 01 12 01 23, then count(p, 4, 0x01) should return 2.

Program code:
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

- Write a C program that uses the following: a main() to read two integer values from the user, val1 and val2, and prints the returned value from swap().a swap() that uses call by reference (takes the addresses into pointers) to swap values, and prints their values after the swap "num1 = # and num2 = #". This function returns the largest of the two values. If these are equal, it returns their sum.Write a recursive function, displayFiles, that expects a pathname as an argument. The path name can be either the name of a file or the name of a directory. If the pathname refers to a file, its filepath is displayed, followed by its contents, like so: File name: file_path Lorem ipsum dolor sit amet, consectetur adipiscing elit... Otherwise, if the pathname refers to a directory, the function is applied to each name in the directory, like so: Directory name: directory_path File name: file_path1 Lorem ipsum dolor sit amet... File name: file_path2 Lorem ipsum dolor sit amet... ...Write a recursive function, displayFiles, that expects a pathname as an argument. The path name can be either the name of a file or the name of a directory. If the pathname refers to a file, its filepath is displayed, followed by its contents, like so: File name: file_path Lorem ipsum dolor sit amet, consectetur adipiscing elit... Otherwise, if the pathname refers to a directory, the function is applied to each name in the directory, like so: Directory name: directory_path File name: file_path1 Lorem ipsum dolor sit amet... File name: file_path2 Lorem ipsum dolor sit amet... ... # Put your code here import os #module used to interact with operating system def displayFiles(pathname): #recursive function that takes a pathname as argument if (os.path.isdir(pathname)): #checks if specified path (argument) is an existing directory #for item in os.listdir(pathname): for content in os.listdir(pathname): #gets the list of all files and directories in the directory and…
- In C, write a function that gets 3 pointers int* a, int* b, int* c, and rotates the values in their addresses to the left. That is, a gets the value of b, b gets the value of c, and c gets the value of a. void rotate3 (int* a, int* b, int* c); For example, if we have int x=1, y=2, z=3, then after calling rotate3 (&x, &y, &z) we should have x==2, y==3, and z==1. if we have int x=7, y=1, z=6, then after calling rotate3 (&x, &y, &z) we should have x==1, y==6, and z==7.Write a function f which takes three pointers to int variables (a, b, c). Then it should:-Set the first variable to the sum of values of the variables (a + b + c).-Set the second variable to difference between maximum and minimum of values ( max(a,b,c) - min(a,b,c) )-Set the third variable to median of a, b, c.For example:if a = 5, b = 10, c = 2Then f(&a, &b, &c) should:Set a to 17 (that is the sum)Set b to 8 (that is max - min = 10 - 2)Set c to 5 (that is the median of 5, 10 and 2) NOTE: You are already provided a test main which checks your code for evaluation. DO NOT MODIFY IT or your answer will NOT be accepted. #include<stdio.h> // Write your code here... //////////////////////////////////////////// THIS IS THE TEST MAIN FOR YOUR PROGRAM// DO NOT MODIFY IT!!int main() {int a = 1;int b = 2;int c = 3;for (int i = 0; i < 2; i++) {f(&a, &b, &c);printf("%d\n", a);printf("%d\n", b);printf("%d\n", c);}return 0;}//////////////////////////////////////////…Write a function in C++ called maximumGap that finds the maximum difference between corresponding entries in two arrays (that have the same capacity). There are 3 parameters, the 2 arrays and their capacity. If the arrays store 9, 0, 3 and 4, 7, 6 the maximum gap is 7 (between the second elements of the two arrays).
- Write a program to find the number of times that a given word occurs in a sentence using pointers. Read data from standard input. The first line is a single word, which is followed by general text on the second line. Read both up to a new line character, and insert a terminating null before processing. Typically output should be : The word is “ the ”. The sentence is “ the cat on the mat “ . The word occurs 2 times. IN C LANGUAGEWrite a function IN C LANGUAGE that splits the following data read from a file named info.txt and store the data into a struct as show in the example: typedef struct{char name[20];char gender[5];char dateOfAdmission[20];char dateOfBirth [20];char illness [20];char address [20];char bloodType[5]; } information; Abed Mukhles#M#2212019#01012000#Ear Infection#Jenin#O+ Here the values are: name:Abed Mukhles gender:M dateOfAdmission:2212019dateOfBirth:01012000illness:Ear Infectionaddress:JeninbloodType:O+ Same for:Nadia A. Ali#F#01102020#05101970#COVID-19#AlBireh#A-In C In C, strings are implemented by a contiguous sequence of characters, terminated by NUL character, otherwise known as ASCII code 0 (10'). CAPI, provides a function stropy for copying strings. The prototype of the function is given in the following: char* strepy(char* destination, const char* source); A) Write your own version of stropy that receives two character pointers and copies the source string into the destination string, character by character, until it reaches a null character. • The function stops when it reaches the end of the string, in which case it makes sure the destination string is properly terminated. • The function returns the original destination pointer as its return value. • The function assumes that source and destination strings are valid pointers. • In case either source or destination pointers are NULL, the function simply returns NULL. B) Write a small program that demonstrates the use of your version of strepy() function. • Define a character array and…
- Write a program in c languageto find the sum of all non-prime numbers from m and n (including m and n also) using the concept of passing pointers to function. Pass addresses of m, n and sum integers from the main () function to the user defined function: calculate () [Function is not returning any value], and display the sum in the main () function.WRITE THE PROGRAM IN C LANGUAGE : Write a program to countNon-Armstrong numbers fromm and n (including m and nalso) using the concept ofpassing pointers to function.Pass addresses of m, n andcount integers from the main ()function to the user definedfunction: calculate () [Functionis not returning any value], anddisplay the count in the main ()function.Write a program in C++ that reads data from a file. Create dynamic memory according to the data. Now your task is to perform the following task. Row wise Sum Column wise Sum Diagonal wise Sum Example data.txt 4 5 1.6 10.2 33.7 99 20.5 3 44 50 96.1 2 8 9 4 74 50 99 19.1 Output: Sum row wise: 165, 191, 17, 242.1 Sum col wise: 127.6, 120.1, 228.8, 118.1, 20.5 Sum diagonal wise: Not Possible Note: You are restricted to use pointers and your function should be generic as we discussed in class. Avoid memory wastage, memory leakage, dangling pointer. Use regrow or shrink concepts if required.
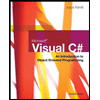
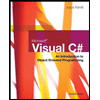