Write a C program (DO NOT USE C++) to do the followings. • Define a node structure that contains an integer data and the next node pointer. • Ask user to enter the total count of nodes (N) in the Linked List. • In a loop, dynamically allocate N nodes one-by-one and add them to the Linked List (unordered) with the rules below: • The value of a node's data should be randomly assigned between 0 and 100. (Use the built-in C functions srand and rand). • If the data is ODD, then the node should be added to the beginning of the linked list. Otherwise it should be added to the end of the linked list. • Program should display the counts of ODD and EVEN numbers. • By looping, program should also display the Linked List as shown below. Sample Screen Output: Enter total count of nodes in Linked List : 20 Count of ODD numbers : 8 Count of EVEN numbers : 12 LINKED LIST : ODD PART: 37 93 87 91 57 67 41 19 EVEN PART: 14 10 56 8 60 16 18 42 38 64 6 60
Write a C program (DO NOT USE C++) to do the followings. • Define a node structure that contains an integer data and the next node pointer. • Ask user to enter the total count of nodes (N) in the Linked List. • In a loop, dynamically allocate N nodes one-by-one and add them to the Linked List (unordered) with the rules below: • The value of a node's data should be randomly assigned between 0 and 100. (Use the built-in C functions srand and rand). • If the data is ODD, then the node should be added to the beginning of the linked list. Otherwise it should be added to the end of the linked list. • Program should display the counts of ODD and EVEN numbers. • By looping, program should also display the Linked List as shown below. Sample Screen Output: Enter total count of nodes in Linked List : 20 Count of ODD numbers : 8 Count of EVEN numbers : 12 LINKED LIST : ODD PART: 37 93 87 91 57 67 41 19 EVEN PART: 14 10 56 8 60 16 18 42 38 64 6 60
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter13: Structures
Section13.4: Linked Lists
Problem 2E
Related questions
Question
100%
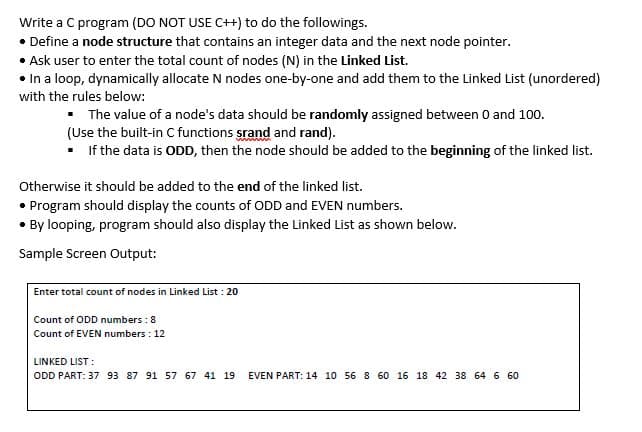
Transcribed Image Text:Write a C program (DO NOT USE C++) to do the followings.
• Define a node structure that contains an integer data and the next node pointer.
• Ask user to enter the total count of nodes (N) in the Linked List.
• In a loop, dynamically allocate N nodes one-by-one and add them to the Linked List (unordered)
with the rules below:
• The value of a node's data should be randomly assigned between 0 and 100.
(Use the built-in C functions srand and rand).
• if the data is ODD, then the node should be added to the beginning of the linked list.
Otherwise it should be added to the end of the linked list.
• Program should display the counts of ODD and EVEN numbers.
• By looping, program should also display the Linked List as shown below.
Sample Screen Output:
Enter total count of nodes in Linked List : 20
Count of ODD numbers : 8
Count of EVEN numbers : 12
LINKED LIST :
ODD PART: 37 93 87 91 57 67 41 19
EVEN PART: 14 10 56 8 60 16 18 42 38 64 6 60
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
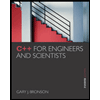
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
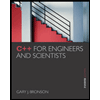
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr