Write a C program for each of the following system calls (one per call) which uses the call in a normal manner. Make sure that your program compiles and executes without error. Show the output from each of the programs. Please check for, and report, any errors. Terminate execution on error (do not continue running if a call fails). a) sbrk b) fork c) sched_getaffinity The last one is a bit tricky. The main page has a lot of useful information on the parameters. The cpu_set_t is an opaque type: You are not meant to manipulate it directly. There are macros in sched.h which will set/clear/test members of the set. The man page for CPU_SET(3) describes these. Of particular interest is CPU_ISSET. The number of cores can be found with sysconf(_SC_NJPROCESSORS_ONLN). This is the number of hyperthreads, not the number of raw cores. It is also the size of the CPU mask. #include #include int main() { long ncores = sysconf(_SC_NPROCESSORS_ONLN); printf ("%d cores found\n", ncores); } The CPU mask is a list of values which are either 1 or 0 depending on whether the given process is allowed to run on that logical core. You can iterate over possible values: for (i = 0; i < ncores; i++) { printf("%d ", CPU_ISSET(i, &mask)); }
Write a C program for each of the following system calls (one per call) which uses the call in a normal manner. Make sure that your program compiles and executes without error. Show the output from each of the programs. Please check for, and report, any errors. Terminate execution on error (do not continue running if a call fails). a) sbrk b) fork c) sched_getaffinity The last one is a bit tricky. The main page has a lot of useful information on the parameters. The cpu_set_t is an opaque type: You are not meant to manipulate it directly. There are macros in sched.h which will set/clear/test members of the set. The man page for CPU_SET(3) describes these. Of particular interest is CPU_ISSET. The number of cores can be found with sysconf(_SC_NJPROCESSORS_ONLN). This is the number of hyperthreads, not the number of raw cores. It is also the size of the CPU mask. #include #include int main() { long ncores = sysconf(_SC_NPROCESSORS_ONLN); printf ("%d cores found\n", ncores); } The CPU mask is a list of values which are either 1 or 0 depending on whether the given process is allowed to run on that logical core. You can iterate over possible values: for (i = 0; i < ncores; i++) { printf("%d ", CPU_ISSET(i, &mask)); }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 31SA
Related questions
Question
please help with this c

Transcribed Image Text:Write a C program for each of the following system calls (one per call) which uses the
call in a normal manner. Make sure that your program compiles and executes without
error. Show the output from each of the programs.
Please check for, and report, any errors. Terminate execution on error (do not continue
running if a call fails).
a) sbrk
b) fork
c) sched_getaffinity
The last one is a bit tricky. The main page has a lot of useful information on the
parameters. The cpu_set_t is an opaque type: You are not meant to manipulate it
directly. There are macros in sched.h which will set/clear/test members of the set. The
man page for CPU_SET(3) describes these. Of particular interest is CPU_ISSET.
The number of cores can be found with sysconf(_SC_NJPROCESSORS_ONLN). This
is the number of hyperthreads, not the number of raw cores. It is also the size of the
CPU mask.
#include <stdio.h>
#include <unistd.h>
int main()
{
long ncores = sysconf(_SC_NPROCESSORS_ONLN);
printf ("%d cores found\n", ncores);
}
The CPU mask is a list of values which are either 1 or 0 depending on whether the
given process is allowed to run on that logical core. You can iterate over possible
values:
for (i = 0; i < ncores; i++)
{
printf("%d ", CPU_ISSET(i, &mask));
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
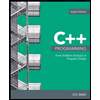
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
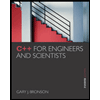
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
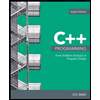
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
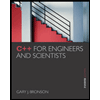
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr