Write a C++ program that helps calculate how long it would take a user to pay off a loan with compound interest. You should prompt the user for the principle amount, how much interest is being charged, and how much they are able to pay back each month. You should assume that all amounts are in dollars and cents, that interest is charged monthly, and that payments are also made monthly. The program should display a table with the following columns: the month number, the amount remaining on the loan, and the total amount already paid. For *each* row, you should: 1. *FIRST* subtract the payment from the amount remaining on the loan. 2. *THEN* add interest based on this new total amount. You should continue to print out rows in the table until either the amount remaining is <= $0 or if 100 months have passed. Then, print out a summary showing the number of months that will elapse and how much total has been paid. If the table stops at 100 months, print out a message telling the user that the loan will take more than 100 months to pay off. As you can see, if you are not careful when taking on loans, it can be costly or even impossible to repay. EXPECTED OUTPUTS Welcome to the compound interest calculator! What is the principle amount you are starting with? 400 What percent interest is compounded per month? 8 // note: 8 is meant to be 8% or 0.08 How much are you able to pay per month? 75 month amount_owed total_paid 0 400.00 0.00 1 351.00 75.00 2 298.08 150.00 3 240.93 225.00 4 179.20 300.00 5 112.54 375.00 6 40.54 450.00 7 -37.22 525.00 It will take you 7 months to fully pay off this debt On a principle of 400.00 you will pay a total of 525.00
Write a C++
The program should display a table with the following columns: the month number, the amount remaining on the loan, and the total amount already paid. For *each* row, you should:
1. *FIRST* subtract the payment from the amount remaining on the loan.
2. *THEN* add interest based on this new total amount.
You should continue to print out rows in the table until either the amount remaining is <= $0 or if 100 months have passed. Then, print out a summary showing the number of months that will elapse and how much total has been paid. If the table stops at 100 months, print out a message telling the user that the loan will take more than 100 months to pay off.
As you can see, if you are not careful when taking on loans, it can be costly or even impossible to repay.
EXPECTED OUTPUTS
Welcome to the compound interest calculator!
What is the principle amount you are starting with? 400
What percent interest is compounded per month? 8 // note: 8 is meant to be 8% or 0.08
How much are you able to pay per month? 75
month amount_owed total_paid
0 400.00 0.00
1 351.00 75.00
2 298.08 150.00
3 240.93 225.00
4 179.20 300.00
5 112.54 375.00
6 40.54 450.00
7 -37.22 525.00
It will take you 7 months to fully pay off this debt
On a principle of 400.00 you will pay a total of 525.00

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

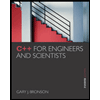
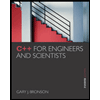