Write a C++ program that searches for "anagrams'' in a dictionary. An anagram is a word obtained by scrambling the letters of some string. For example, the word "pot'' is an anagram of the string "otp." For this solution you must not use recursion. Instead, use the std::next_permutation function in the algorithm library. Here is a downloadable link to the words.txt file, but you must be logged in to a google account for it to show up: https://drive.google.com/file/d/1GSIp0R224wnJUARW1qj1AnBWKO-cZyS8/view?usp=sharing Here is a sample of how the program might work: Please enter a string for an anagram: opt Matching word opt Matching word pot Matching word top Here is another sample of how the program might work: Please enter a string for an anagram: blah No matches found You must write these two functions with the exact same function signature (include case): int loadDictionary(istream &dictfile, vector& dict); Places each string in dictfile into the vector dict. Returns the number of words loaded into dict. int permute(string word, vector& dict, vector& results); Places all the permutations of word, which are found in dict into results. Returns the number of matched words found. For words with double letters, you may find that different permutations match the same word in the dictionary. For example, if you find all the permutations of the string kloo using the algorithm we've discussed you may find that the word look is found twice. The o's in kloo take turns in front. Your program should ensure that matches are unique, in other words, the results array returned from the permute function should have no duplicates. Here is an example program to base off: #include #include #include #include #include #include using namespace std; const int MAXRESULTS = 20; // Max matches that can be found const int MAXDICTWORDS = 30000; // Max words that can be read in int loadDictionary(istream& dictfile, vector& dict); int permute(string word, vector& dict, vector& results); void print(vector& results, int size); int main() { vector results(MAXRESULTS); vector dict(MAXDICTWORDS); ifstream dictfile; // file containing the list of words int nwords; // number of words read from dictionary string word; dictfile.open("words.txt"); if (!dictfile) { cout << "File not found!" << endl; return (1); } nwords = loadDictionary(dictfile, dict); cout << "Please enter a string for an anagram: "; cin >> word; int numMatches = permute(word, dict, results); if (!numMatches) cout << "No matches found" << endl; else print(results, numMatches); } int loadDictionary(istream& dictfile, vector& dict) { } int permute(string word, vector& dict, vector& results) { } void print(vector& results, int size) { } If you have ANY questions, please leave a comment and I will respond as soon as possible
Write a C++ program that searches for "anagrams'' in a dictionary. An anagram is a word obtained by scrambling the letters of some string. For example, the word "pot'' is an anagram of the string "otp." For this solution you must not use recursion. Instead, use the std::next_permutation function in the
Here is a downloadable link to the words.txt file, but you must be logged in to a google account for it to show up: https://drive.google.com/file/d/1GSIp0R224wnJUARW1qj1AnBWKO-cZyS8/view?usp=sharing
Here is a sample of how the program might work:
Please enter a string for an anagram: opt
Matching word opt
Matching word pot
Matching word top
Here is another sample of how the program might work:
Please enter a string for an anagram: blah
No matches found
You must write these two functions with the exact same function signature (include case):
int loadDictionary(istream &dictfile,
Places each string in dictfile into the vector dict. Returns the number of words loaded into dict.
int permute(string word, vector<string>& dict, vector<string>& results);
Places all the permutations of word, which are found in dict into results. Returns the number of matched words found.
For words with double letters, you may find that different permutations match the same word in the dictionary. For example, if you find all the permutations of the string kloo using the algorithm we've discussed you may find that the word look is found twice. The o's in kloo take turns in front. Your program should ensure that matches are unique, in other words, the results array returned from the permute function should have no duplicates.
Here is an example program to base off:
#include <iostream>
#include <fstream>
#include <istream>
#include <string>
#include <algorithm>
#include <vector>
using namespace std;
const int MAXRESULTS = 20; // Max matches that can be found
const int MAXDICTWORDS = 30000; // Max words that can be read in
int loadDictionary(istream& dictfile, vector<string>& dict);
int permute(string word, vector<string>& dict, vector<string>& results);
void print(vector<string>& results, int size);
int main() {
vector<string> results(MAXRESULTS);
vector<string> dict(MAXDICTWORDS);
ifstream dictfile; // file containing the list of words
int nwords; // number of words read from dictionary
string word;
dictfile.open("words.txt");
if (!dictfile) {
cout << "File not found!" << endl;
return (1);
}
nwords = loadDictionary(dictfile, dict);
cout << "Please enter a string for an anagram: ";
cin >> word;
int numMatches = permute(word, dict, results);
if (!numMatches)
cout << "No matches found" << endl;
else
print(results, numMatches);
}
int loadDictionary(istream& dictfile, vector<string>& dict) {
}
int permute(string word, vector<string>& dict, vector<string>& results) {
}
void print(vector<string>& results, int size) {
}
If you have ANY questions, please leave a comment and I will respond as soon as possible

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

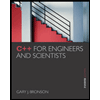
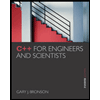