Write a C++ program to check for the not-eligible donor by throwing a custom exception. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class Donor has the following protected variables. Data Type Variable string name int age int weight string bloodGroup
READ THE QUESTION CAREFULLY. AND WHILE CREATING DONOR CLASS MUST INCLUDE EVERY FUNCTION SHOWN IN QUESTION.
----------------------------------------
Write a C++ program to check for the not-eligible donor by throwing a custom exception.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.
The class Donor has the following protected variables.
Data Type | Variable |
string | name |
int | age |
int | weight |
string | bloodGroup |
In the Donor class, define the following function.
Method | Description |
bool validateDonor(Donor donor) |
This method validates donor eligibility. Validate the donor details with the following conditions. 1.Age must be greater than 30 then the donor is eligible. 2.If the age is less than 30 then throw an exception and check the weight should be greater than 44 kg in catch block, If the weight is also less than 44 then the donor is not eligible and rethrow the exception to the main method. If the weight is greater than 44 then the donor is eligible.
Validate the donor to check the donor is eligible or not. |
In the Main method, read the donor details from the user and create corresponding objects.
Input and Output Format:
Refer sample input and output for formatting specifications.
All text in bold corresponds to the input and the rest corresponds to output.
Sample Input and Output 1:
Enter donor details
Enter the name
John
Enter the age
34
Enter the weight
46
Enter the blood group
O+ve
Donor is eligible
Sample Input and Output 2:
Enter donor details
Enter the name
Daniel
Enter the age
25
Enter the weight
50
Enter the blood group
O+ve
InvalidDonorException:Age is not valid
Donor is eligible
Sample Input and Output 3:
Enter donor details
Enter the name
Harry
Enter the age
28
Enter the weight
42
Enter the blood group
B+ve
InvalidDonorException:Both age and weight is not valid
Donor is not eligible
--------STRICTLY USE BELOW TEMPLATE-------
DONOR.CPP
#include <iostream> #include <sstream> using namespace std; class Donor{ protected: string name; int age; int weight; string bloodGroup; public: Donor(){} Donor(string name, int age, int weight,string bloodGroup) { this->name = name; this->age = age; this->weight = weight; this->bloodGroup = bloodGroup; } bool validateDonor(Donor donor) { //Fill your code here } }; |
MAIN.CPP
#include <iostream> #include <sstream> #include "Donor.cpp" using namespace std; int main() { //Fill your code here try { //Fill your code here } catch(const char* c) { //Fill your code here } return 0; } |

Step by step
Solved in 4 steps with 2 images

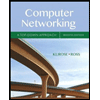
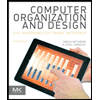
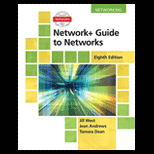
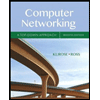
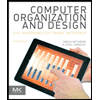
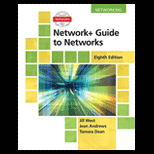
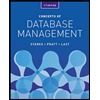
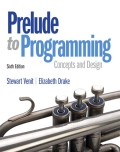
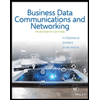