Write a C program to create the double arrays A and B, using the gen rand mat function.
Write a C

Program Approach:
- Including a necessary header file
- Declaring a method to generate a random floating-point number from min to max
- Declaring the main method
- Declaring two arrays A and B
- Declaring integer variable i
- Using for loop to store value in the array
- Display the message
- Using for loop to generating a random value
- Display the value
Program:
//including necessary header file
#include <stdio.h>
#include <stdlib.h>
//declaring a method to generate a random floating point number from min to max
double gen_rand_num(double min, double max)
{
double range = (max - min);
double num = RAND_MAX / range;
return min + (rand() / num);
}
//declaring main method
int main(){
//declaring two array A and B
double A[10],B[10];
//declaring integer variable i
int i;
//using for loop to store value in array
for(i=0;i<10;i++){
A[i] = gen_rand_num(1,100);
B[i] = gen_rand_num(1,100);
}
//display the message
printf("Random generated double array 1:\n");
//using for loop to generating random value
for(i=0;i<10;i++)
//display the values
printf("%f ",A[i]);
//display the message
printf("\nRandom generated double array 2:\n");
//using for loop to generating random value
for(i=0;i<10;i++)
//display the value
printf("%f ",B[i]);
//return the value
return 0;
}
Step by step
Solved in 3 steps with 1 images

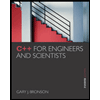
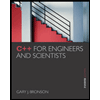