Write a C++ program to print unique words in a file.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write the following
- Write a C++ program to print unique words in a file.
- Write a C++ program to print how many words in a sentence from a file.
- By using student class with member’s variables and member functions, write a C++ program that read student information from text file.
- The following program shows the Operators Overloading for addition(+) operator function, now for the same operators overloading program do the operation for subtraction(-), multiplication(*) and division(/) functions.
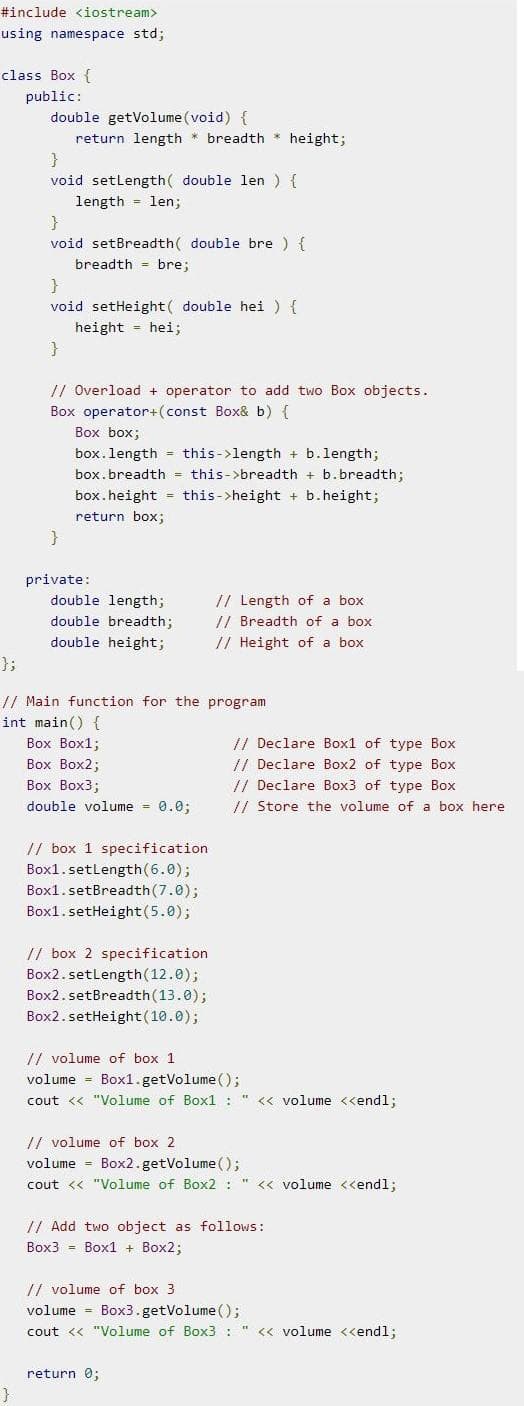
Transcribed Image Text:#include <iostream>
using namespace std;
class Box {
public:
double getVolume (void) {
return length * breadth * height;
}
void setlength( double len ) {
length - len;
}
void setBreadth( double bre ) {
breadth = bre;
}
void setHeight( double hei ) {
height = hei;
}
// Overload + operator to add two Box objects.
Box operator+(const Box& b) {
Box box;
box.length = this->length + b.length;
box.breadth = this->breadth + b.breadth;
box.height = this->height + b.height;
return box;
private:
// Length of a box
// Breadth of a box
// Height of a box
double length;
double breadth;
double height;
};
// Main function for the program
int main() {
// Declare Box1 of type Box
// Declare Box2 of type Box
// Declare Box3 of type Box
// Store the volume of a box here
Box Box1;
Box Box2;
Box Box3;
double volume = 0.0;
// box 1 specification
Box1.setlength(6.0);
Box1.setBreadth(7.0);
Box1.setHeight(5.0);
// box 2 specification
Box2. setlength(12.0);
Box2.setBreadth(13.0);
Box2. setHeight(10.0);
// volume of box 1
volume = Box1.getVolume();
cout << "Volume of Box1 :
<< volume <<endl;
// volume of box 2
volume - Box2.getVolume ();
cout << "Volume of Box2 : " << volume <<endl;
// Add two object as follows:
Box3 - Box1 + Box2;
// volume of box 3
volume = Box3.getVolume ();
cout <« "Volume of Box3 : " <« volume <<endl;
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
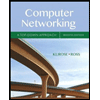
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
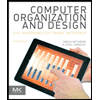
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
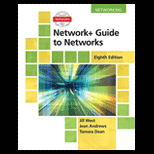
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
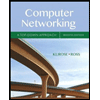
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
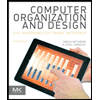
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
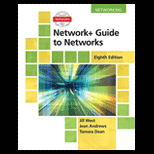
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
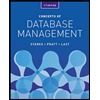
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
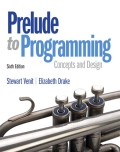
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
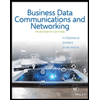
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY