Write a C++ program to read details of invoices from a file and to output invoices which indicate the total cost of each item and the total cost of the invoice together with full details.
Write a C++
Details of an invoice are:
An invoice header with number of items on the invoice and the date of the invoice
For each item, an item code (6 digits), a name, a quantity and a unit cost.
Thus a typical set of lines in the file for invoices might be:
3 2/12/2017
134276 Framis-R 8 7.35
125790 Framis-L 12 15.76
100086 Aglet 395 0.11
2 1/23/2017
135876 Wrench 12 22.50
543287 Henway 4 19.25
The above indicates that there are three items on the first invoice and its date is 2/12/2017, the first item has an item code of 134276 is a Framis-R an order quantity of 8 and a unit price of $7.35. There are two items on the next invoice, etc.
Write a C++ program to read the file (input.txt) with details of invoices and to output to a file an invoice which indicates the total cost of each item and the total cost of the invoice together with full details. The above details might produce a file as follows:
Erie Industrial Supply Corporation
Invoice date: 1/15/2017
134246 Framis-R 8 7.35 58.80
125790 Framis-L 12 15.76 189.12
100086 Aglet 395 0.11 43.45
Total ................291.37
Invoice date: 1/23/2017
135876 Wrench 12 22.50 270.00
543287 Henway 4 19.25 77.00
Total ...............347.00
The functions shall be specified as follows:
Function type : double
Function name : calccost
Operation : Calculates the cost for a single item.
Description : Given the unit price of an item in
dollars and cents and the quantity of
the item, it calculates the total cost in
dollars and cents
Parameters : int quantity
double unitcost
Return : totalCost
--------------------------------------------------------------------------
Function type : double
Function name : acctotal
Operation : Accumulates the total cost of invoice
Description : Given current total invoice cost and
the total cost of an invoice item
calculates the new total invoice cost.
Parameters : double totalCost
double itemCost
Return : newTotalCost
--------------------------------------------------------------------------
Function type : void
Function name : printLine
Operation : Writes a line of the invoice.
Description : Given the item reference number, the name, the
quantity, the unit price and total
price of an item, outputs a line of
the invoice.
Parameters : string itemno
string name
int quantity
double unitCost
double totalCost
--------------------------------------------------------------------------
Function type : void
Function name : printInvoiceHeader
Operation : Writes “Invoice date: “ and the date to the file
Description : Accepts the date and writes Invoice date: followed by the
date into the file.
Parameters : string date
--------------------------------------------------------------------------
Function type : void
Function name : printReportHeader
Operation : Writes “Erie Industrial Supply Corporation” as the first
line of the file
Description : Displays the company name
Parameters : None
--------------------------------------------------------------------------
Function type : void
Function name : printTotal
Operation : Writes “Total ……………………………………………………………………………” followed
by the total and then an endl in the file.
Description : Accepts the total and writes it followed by a line of dots
and then the invoice total and an endl
date into the file.
Parameters : double invoiceTotal
The pseudocode for main would be:
Open output file (Before main, create output file with the line:
ofstream fout;
Create and open input file in main.
Be sure to test to see if you can read the file. If not display a message and end the program.
Read number of items and date. Date can just be a string. This will be the main read loop which will end the program when it encounters end of file
printReportHeader()
while( fin >> items >> date)
totalCost = 0
printInvoiceHeader (date)
Use a for loop (i=0;i<number of items) to read line items:
fin >> item >> name >> quantity >> unitPrice
totalcost = calcCost(quantity, unitPrice)
invoiceCost = accTotal(invoiceCost, totalCost)
printLine(itemno, name, quantity, unitCost, totalCost)
end for
printTotal(invoiceCost)
End main read loop

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

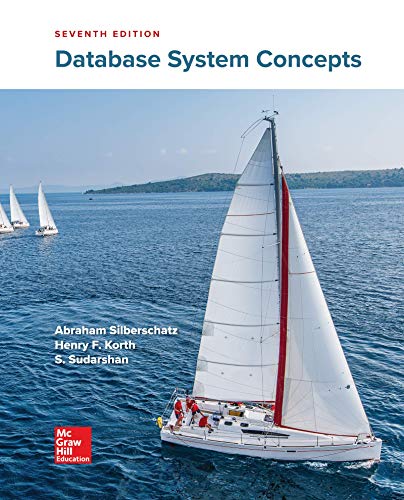
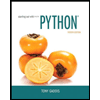
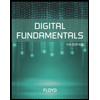
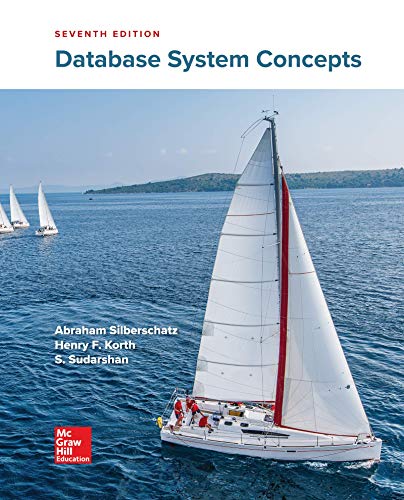
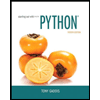
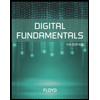
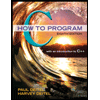
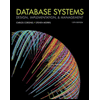
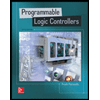