Write a complete Java program, including comments, to do the following: Your program will compute the monthly payment (monPayment - double) on a mortgage of amount principal (integer), with interest rate intRate (double) for numYears (integer) number of years. The formula is written as follows: principal*(intRate/12)*(1+(intRate/12))numYears"12 monPayment = ((1+(intRate/12))rumYears"12 )-1 For example if principal=$100,000, intRate=.07 and numYears=30 years then the monthly payment is $665. Check that your code yields the same number before you go any further. Use the pow function to raise a number to a power. Math.pow(10,5) means 10 to the 5th power. To raise a*b to the power of c*d use Math.pow(a*b, c*d). 1. The program should start by printing a header giving your name, class/section and assignment number. Use a loop to print underscores (_ ) as a horizontal line to add clarity to the report (see sample output below). 2. The program will compute the monthly payment of loans starting at $100,000 up to an including $1,000,000 (in increments of $100,000) at 7 different interest rates (starting at .07 up to and including .1 in increments of .005). The outer loop will run from 100000 to 1000000 and the inner loop from .07 to .1 and the formula for computing the monthly payment will be part of the inner loop 3. Use a printf statement for the column header, allowing 3 decimal places for the interest rate. The total field size for intRate should match what you use for the monthly payment (in the section below) 4. Use printf statements for: (a) the principal having no decimal places and max size of 7 positions and (b) monthly payment containing 2 decimal places and up to 4 positions to the left of the decimal place. Align all numbers to the right and align the monthly payments with the interest rate header above it. The final result should look as follows. Your numbers should exactly match those below. Also, when you copy the output, the font should be Courier New or any other fixed font so that the columns will line up properly. CISC 1115 TR11 Homework 4A Principal Interest Rate 0.080 0.070 0.075 0.085 0.090 0.095 0.100 100000 665.30 699.21 733.76 768.91 804.62 840.85 877.57 200000 1330.60 1398.43 1467.53 1537.83 1609.24 1681.71 1755.14 300000 1995.90 2097.64 2201.29 2306.74 2413.86 2522.56 2632.72 800000 5322.41 5987.71 5593.71 6292.92 5870.12 6603.88 6151.31 6920.23 6436.97 7241.59 6726.83 7567.69 7020.58 7898.15 900000 1000000 6653.01 6992.14 7337.65 7689.14 8046.22 8408.54 8775.72
Write a complete Java program, including comments, to do the following: Your program will compute the monthly payment (monPayment - double) on a mortgage of amount principal (integer), with interest rate intRate (double) for numYears (integer) number of years. The formula is written as follows: principal*(intRate/12)*(1+(intRate/12))numYears"12 monPayment = ((1+(intRate/12))rumYears"12 )-1 For example if principal=$100,000, intRate=.07 and numYears=30 years then the monthly payment is $665. Check that your code yields the same number before you go any further. Use the pow function to raise a number to a power. Math.pow(10,5) means 10 to the 5th power. To raise a*b to the power of c*d use Math.pow(a*b, c*d). 1. The program should start by printing a header giving your name, class/section and assignment number. Use a loop to print underscores (_ ) as a horizontal line to add clarity to the report (see sample output below). 2. The program will compute the monthly payment of loans starting at $100,000 up to an including $1,000,000 (in increments of $100,000) at 7 different interest rates (starting at .07 up to and including .1 in increments of .005). The outer loop will run from 100000 to 1000000 and the inner loop from .07 to .1 and the formula for computing the monthly payment will be part of the inner loop 3. Use a printf statement for the column header, allowing 3 decimal places for the interest rate. The total field size for intRate should match what you use for the monthly payment (in the section below) 4. Use printf statements for: (a) the principal having no decimal places and max size of 7 positions and (b) monthly payment containing 2 decimal places and up to 4 positions to the left of the decimal place. Align all numbers to the right and align the monthly payments with the interest rate header above it. The final result should look as follows. Your numbers should exactly match those below. Also, when you copy the output, the font should be Courier New or any other fixed font so that the columns will line up properly. CISC 1115 TR11 Homework 4A Principal Interest Rate 0.080 0.070 0.075 0.085 0.090 0.095 0.100 100000 665.30 699.21 733.76 768.91 804.62 840.85 877.57 200000 1330.60 1398.43 1467.53 1537.83 1609.24 1681.71 1755.14 300000 1995.90 2097.64 2201.29 2306.74 2413.86 2522.56 2632.72 800000 5322.41 5987.71 5593.71 6292.92 5870.12 6603.88 6151.31 6920.23 6436.97 7241.59 6726.83 7567.69 7020.58 7898.15 900000 1000000 6653.01 6992.14 7337.65 7689.14 8046.22 8408.54 8775.72
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java nested loops

Transcribed Image Text:Write a complete Java program, including comments, to do the following: Your program will compute the
monthly payment (monPayment - double) on a mortgage of amount principal (integer), with interest
rate intRate (double) for numYears (integer) number of years.
The formula is written as follows:
principal*(intRate/12)*(1+(intRate/12))numYears*12
monPayment =
((1+(intRate/12))numYears*12 )-1
For example if principal=$100,000, intRate=.07 and numYears=30 years then the monthly payment is
$665. Check that your code yields the same number before you go any further.
Use the pow function to raise a number to a power. Math.pow(10,5) means 10 to the 5th power. To raise
a*b to the power of c*d use Math.pow(a*b, c*d).
1. The program should start by printing a header giving your name, class/section and assignment number.
Use a loop to print underscores (_ ) as a horizontal line to add clarity to the report (see sample output
below).
2. The program will compute the monthly payment of loans starting at $100,000 up to an including
$1,000,000 (in increments of $100,000) at 7 different interest rates (starting at .07 up to and
including .1 in increments of .005). The outer loop will run from 100000 to 1000000 and the inner loop
from .07 to .1 and the formula for computing the monthly payment will be part of the inner loop
3. Use a printf statement for the column header, allowing 3 decimal places for the interest rate. The total
field size for intRate should match what you use for the monthly payment (in the section below)
4. Use printf statements for: (a) the principal having no decimal places and max size of 7 positions and
(b) monthly payment containing 2 decimal places and up to 4 positions to the left of the decimal place.
Align all numbers to the right and align the monthly payments with the interest rate header above it.
The final result should look as follows. Your numbers should exactly match those below. Also, when
you copy the output, the font should be Courier New or any other fixed font so that the columns
will line up properly.
CISC 1115 TR11
Homework 4A
<YOUR NAME>
Principal
Interest Rate
0.070
0.075
0.080
0.085
0.090
0.095
0.100
100000
665.30
699.21
733.76
768.91
804.62
840.85
877.57
200000
1330.60
1398.43
1467.53
1537.83
1609.24
1681.71
1755.14
300000
1995.90
2097.64
2201.29
2306.74
2413.86
2522.56
2632.72
5322.41
5987.71
800000
5593.71
5870.12
6151.31
6436.97
6726.83
7020.58
900000
6292.92
6603.88
6920.23
7241.59
7567.69
7898.15
1000000
6653.01
6992.14
7337.65
7689.14
8046.22
8408.54
8775.72
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
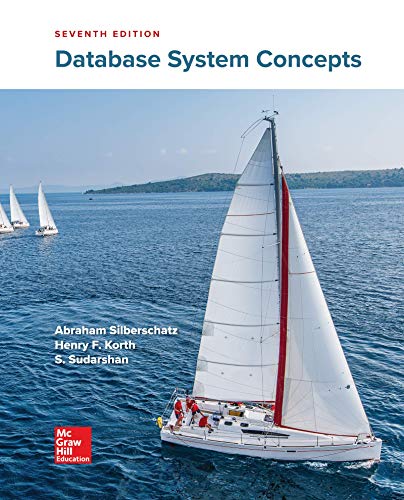
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
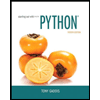
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
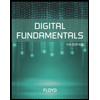
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
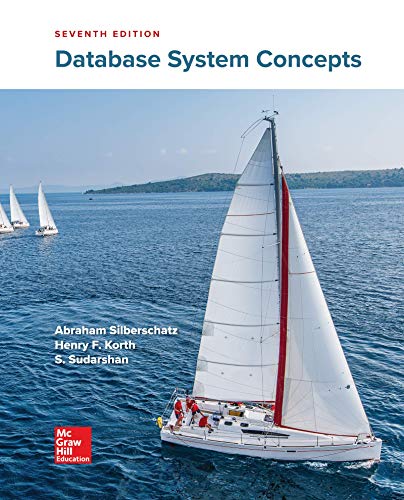
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
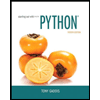
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
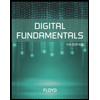
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
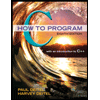
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
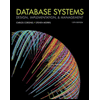
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
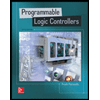
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education