Write a complete Java program which will simulate the playing of a game of dice. The program will do the following: 1. The main method will ask the user to type in two integer values, each in the range from 1 to 6, into variables called diel and die2. Use a boolean method valid to determine whether a die is valid or not. Bad data is to be rejected (except for the special combination you use to end the set of data). For example, if someone types in 3 and -2, the combination is to be rejected. The main method will print the numbers as soon as they are read in. 2. The main method will send these two integer values to a method named outcome. The method will determine the outcome of using these two numbers, according to this scheme: a. If the numbers add up to 5, 7, or 12, then the player wins, and the method should return an indication of this (use some integer to represent a win). b. If the numbers add up to 2, 4, or 11, then the player loses, and the method should return an indication of this (once again, use an integer). c. If the numbers add up to anything else, then the game is a draw and the method should say this (by way of an integer). 5. Finally, the main method will continue wit user types in a special combination (you mu must explain it to the person using Data: 3 2 31 34 36 51 56 55 52 :
Write a complete Java program which will simulate the playing of a game of dice. The program will do the following: 1. The main method will ask the user to type in two integer values, each in the range from 1 to 6, into variables called diel and die2. Use a boolean method valid to determine whether a die is valid or not. Bad data is to be rejected (except for the special combination you use to end the set of data). For example, if someone types in 3 and -2, the combination is to be rejected. The main method will print the numbers as soon as they are read in. 2. The main method will send these two integer values to a method named outcome. The method will determine the outcome of using these two numbers, according to this scheme: a. If the numbers add up to 5, 7, or 12, then the player wins, and the method should return an indication of this (use some integer to represent a win). b. If the numbers add up to 2, 4, or 11, then the player loses, and the method should return an indication of this (once again, use an integer). c. If the numbers add up to anything else, then the game is a draw and the method should say this (by way of an integer). 5. Finally, the main method will continue wit user types in a special combination (you mu must explain it to the person using Data: 3 2 31 34 36 51 56 55 52 :
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
088..
![Assignment # 3
Write a complete Java program which will simulate the playing of a game of dice. The
program will do the following:
into
1. The main method will ask the user to type in two integer values, each in the range from 1 to
variables called diel and die2. Use a boolean method valid to determine whether a die is valid
or not. Bad data is to be rejected (except for the special combination you use to end the set of
data). For example, if someone types in 3 and -9, the combination is to be rejected. The main
method will print the numbers as soon as they are read in.
2. The main method will send these two integer values to a method named outcome. The method
will determine the outcome of using these two numbers, according to this scheme:
a. If the numbers add up to 5, 7, or 12, then the player wins, and the method should return an
indication of this (use some integer to represent a win).
b. If the numbers add up to 2, 4, or 11, then the player loses, and the method should return an
indication of this (once again, use an integer).
c. If the numbers add up to anything else, then the game is a draw and the method should say
this (by way of an integer).
When the method returns to the main method, the main method will use the value returned by the
method to print an appropriate message, describing which of the three cases applies in this
situation.
3. The main method will then add 2 to the value on the first die and 3 to the value on the second die.
However, if the new value would turn out to be more than 6, the new value should be adjusted
to fit the range from 1 to 6. [For example, if the two old values were 5 and 2, the two new
values would be 1 (5+2-6=1) and 5 (2+3=5). If the two old values were 1 and 5, the two
new ones would be 3 (1+2=3) and 2 (5+3-6=2).] The main method should print the two
new values, adjusted if necessary, then call method outcome again with these new values. Once
again, the main method will print the result of the method call.
4. The main method is to keep statistics about the various results. At the very end, print these statistics.
For example, keep track of how many times the original pair of dice gave a winner, a loser, or a
draw. Do the same for the new pair. Keep track of the amount of double winners, double losers,
etc.
5. Finally, the main method will continue with step 1 with a new pair of dice. At step 1, if the
user types in a special combination (you must determine what this combination is, and you
must explain it to the person using the program), the program will halt.
Data: 32
31
34
36
51
56
55
52
Make up a total of about 15-20 games. Make sure that you include each of the three possible
winning sums; make sure that you include each of the three losing sums; be sure to have one set
with two winners, one with two losers, and one with a win and a loss. Also include at least one
set of invalid data. Be sure that the output of your program is clear enough to allow someone to
follow the game. You may want to place all the data in a file.
You may not use any class variables. All values should be passed to methods using
parameters. All output it to go to a file.
Extra Credit:
Investigate the use of Java's built in random number method. In addition to submitting the
above, modify the program so that it plays a predetermined number of games by itself, without
any user intervention.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7bfddd47-a914-4f67-b7e8-f2b18f1a0345%2F548b4ca4-0bb7-4f9d-9f66-bd592d1d76db%2Fipgslie_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Assignment # 3
Write a complete Java program which will simulate the playing of a game of dice. The
program will do the following:
into
1. The main method will ask the user to type in two integer values, each in the range from 1 to
variables called diel and die2. Use a boolean method valid to determine whether a die is valid
or not. Bad data is to be rejected (except for the special combination you use to end the set of
data). For example, if someone types in 3 and -9, the combination is to be rejected. The main
method will print the numbers as soon as they are read in.
2. The main method will send these two integer values to a method named outcome. The method
will determine the outcome of using these two numbers, according to this scheme:
a. If the numbers add up to 5, 7, or 12, then the player wins, and the method should return an
indication of this (use some integer to represent a win).
b. If the numbers add up to 2, 4, or 11, then the player loses, and the method should return an
indication of this (once again, use an integer).
c. If the numbers add up to anything else, then the game is a draw and the method should say
this (by way of an integer).
When the method returns to the main method, the main method will use the value returned by the
method to print an appropriate message, describing which of the three cases applies in this
situation.
3. The main method will then add 2 to the value on the first die and 3 to the value on the second die.
However, if the new value would turn out to be more than 6, the new value should be adjusted
to fit the range from 1 to 6. [For example, if the two old values were 5 and 2, the two new
values would be 1 (5+2-6=1) and 5 (2+3=5). If the two old values were 1 and 5, the two
new ones would be 3 (1+2=3) and 2 (5+3-6=2).] The main method should print the two
new values, adjusted if necessary, then call method outcome again with these new values. Once
again, the main method will print the result of the method call.
4. The main method is to keep statistics about the various results. At the very end, print these statistics.
For example, keep track of how many times the original pair of dice gave a winner, a loser, or a
draw. Do the same for the new pair. Keep track of the amount of double winners, double losers,
etc.
5. Finally, the main method will continue with step 1 with a new pair of dice. At step 1, if the
user types in a special combination (you must determine what this combination is, and you
must explain it to the person using the program), the program will halt.
Data: 32
31
34
36
51
56
55
52
Make up a total of about 15-20 games. Make sure that you include each of the three possible
winning sums; make sure that you include each of the three losing sums; be sure to have one set
with two winners, one with two losers, and one with a win and a loss. Also include at least one
set of invalid data. Be sure that the output of your program is clear enough to allow someone to
follow the game. You may want to place all the data in a file.
You may not use any class variables. All values should be passed to methods using
parameters. All output it to go to a file.
Extra Credit:
Investigate the use of Java's built in random number method. In addition to submitting the
above, modify the program so that it plays a predetermined number of games by itself, without
any user intervention.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
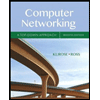
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
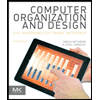
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
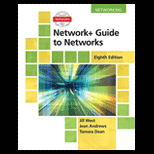
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
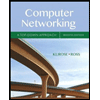
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
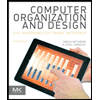
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
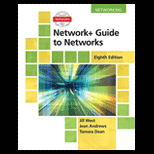
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
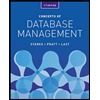
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
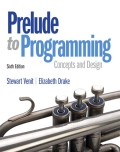
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
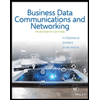
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY