Write a e a program that plays a number guessing game with a Human user. The Human user will think of a number between LOWER LIMIT and UPPER LIMIT, inclusive. (LOWER LIMIT and UPPER LIMIT will be global constants, typically 1 and 100, but your program must still work if they are set to so different. You may assume that LOWER LIMIT < UPPER LIMIT.) The program will make guesses and the user will tell the program to guess higher or lower. A sample run of the program might look like this: Ready to play (y/n)? Think of a number between 1 and 100. My guess is 50. Enter 1 if your number is lower, h if it is higher, 'e' if it is correct: My guess is 75. Enter 1 if your number is lover, h if it is higher, 'e' if it is correct: h My guess is 88. Enter 1 if your number is lower, 'h' if it is higher, 'e' if it is correct: 1 My guess is 81. Enter 1 if your number is lower, 'h' if it is higher, 'e' if it is correct: e Great! Do you want to play again (y/n)? y Think of a number between 1 and 100. My quess is 50. Enter 1 it your number is lower, 'h' if it is higher, 'e' if it is correct: 1 Hy guess is 25. Enter 1: if your number is lower, h if it is higher, e if it is correct: h My guess is 37: Enter 1 if your number is lover, 'h' if it is higher, 'e' if it is correct: e Great! Do you want to play again (y/n)? n over, The strategy that the program will use goes like this: Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50. The user responds by saying "higher" On the second guess the possible values are $1 to 100. The midpoint is 75. The user responds by saying "higher" On the third guess the possible values are 76 to 100. The midpoint is 88. The user responds by saying "lower"
Write a e a program that plays a number guessing game with a Human user. The Human user will think of a number between LOWER LIMIT and UPPER LIMIT, inclusive. (LOWER LIMIT and UPPER LIMIT will be global constants, typically 1 and 100, but your program must still work if they are set to so different. You may assume that LOWER LIMIT < UPPER LIMIT.) The program will make guesses and the user will tell the program to guess higher or lower. A sample run of the program might look like this: Ready to play (y/n)? Think of a number between 1 and 100. My guess is 50. Enter 1 if your number is lower, h if it is higher, 'e' if it is correct: My guess is 75. Enter 1 if your number is lover, h if it is higher, 'e' if it is correct: h My guess is 88. Enter 1 if your number is lower, 'h' if it is higher, 'e' if it is correct: 1 My guess is 81. Enter 1 if your number is lower, 'h' if it is higher, 'e' if it is correct: e Great! Do you want to play again (y/n)? y Think of a number between 1 and 100. My quess is 50. Enter 1 it your number is lower, 'h' if it is higher, 'e' if it is correct: 1 Hy guess is 25. Enter 1: if your number is lower, h if it is higher, e if it is correct: h My guess is 37: Enter 1 if your number is lover, 'h' if it is higher, 'e' if it is correct: e Great! Do you want to play again (y/n)? n over, The strategy that the program will use goes like this: Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50. The user responds by saying "higher" On the second guess the possible values are $1 to 100. The midpoint is 75. The user responds by saying "higher" On the third guess the possible values are 76 to 100. The midpoint is 88. The user responds by saying "lower"
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 20PE
Related questions
Question
Provide full C++ code

Transcribed Image Text:Write a program that plays a number guessing game with a Human user. The Human user will think of a number between LOWER_LIMIT and UPPER_LIMIT, inclusive. (LOWER_LIMIT and UPPER_LIMIT will be global constants, typically 1 and 100, but your program must still work if they are set to something
different. You may assume that LOWER_LIMIT < UPPER_LIMIT.) The program will make guesses and the user will tell the program to guess higher or lower.
A sample run of the program might look like this:
Ready to play (y/n)? y
Think of a number between 1 and 100.
My guess is 50. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: h
My guess is 75. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: h
My guess is 88. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: 1
My guess is 81. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: c
Great! Do you want to play again (y/n)? y
Think of a number between 1 and 100.
My guess is 50. Enter '1' if your number is
My guess is 25. Enter '1' if your number is
My guess is 37. Enter '1' if your number is lower, 'h' if it is higher, 'c' if it is correct: c
Great! Do you want to play again (y/n)? n
The strategy that the program will use goes like this: Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81:
On the first guess, the possible values are 1 to 100. The midpoint is 50.
The user responds by saying "higher"
On the second guess the possible values are 51 to 100. The midpoint is 75.
The user responds by saying "higher"
lower, 'h' if it is
lower, 'h' if it is
On the third guess the possible values are 76 to 100. The midpoint is 88.
The user responds by saying "lower"
On the fourth guess the possible values are 76 to 87. The midpoint is 81.
The user responds "correct"
Additional Requirements
int main() {
The purpose of the assignment is to practice writing functions. Although it would be possible to write the entire program in the main function, your solution should be heavily structured. Most of the point penalties given on this assignment will be for not following the instructions below carefully. The main
function must look exactly like this. Copy and paste this code into your file, and don't edit it:
char response;
cout << "Ready to play (y/n)? ";
cin >> response;
higher, 'c' if it is correct: 1
higher, 'c' if it is correct: h
while (response ==
'y') {
playOneGame ( ) ;
cout << "Great! Do you want to play again (y/n)? ";
cin >> response;
}
The playOneGame() function must implement a complete guessing game using the range LOWER_LIMIT to UPPER_LIMIT.
In addition, you must implement the following helper functions to be invoked inside your playOneGame() function:
void getUserResponseToGuess(int guess, char& result)
The getUserResponseToGuess() function should prompt the user to enter 'h', 'I', or 'c' (as shown in the sample output). It should set its "result" parameter equal to whatever the user enters in response. It should do this ONE time, and should not do anything else. Note that printing the guess is part of the
prompt.
int getMidpoint (int low, int high)
The getMidpoint() function should accept two integers, and it should return the midpoint of the two integers. If there are two values in the middle of the range then you should consistently chose the smaller of the two.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
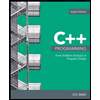
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
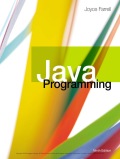
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
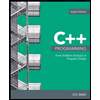
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
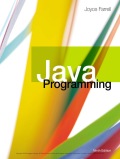
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
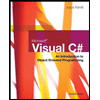
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,