Write a function called safeAdd(d, k, v, unsafe=False) that takes either three or four input parameters when called. Here, d is a dictionary, k is a key and v is a value. The function will add the key:value pair k:v to the dictionary d if the key k is NOT in the dictionary. If the key is in the dictionary, the function will display the current value for that key, the new value you passed into the function and ask the user if they wish to proceed and overwrite the existing value. If the optional fourth parameter is True, then just add the key:value pair without checking if the key is already present. Sample run (user input is highlighted) >>> d = {'A' :1} >>> safeAdd(d, 'B', 3} >>> display (d) А, 1 В, з >>> safeAdd (d, 'B', 2) The key B is already in the dictionary. Current value is 3. Do you want to replace this value with 2? [yes/no] yes >>> display(d) А, 1 В, 2 >>> safeAdd(d, 'A', 12) The key A is already in the dictionary. Current value is 1. Do you want to replace this value with 12? [yes/no] no >>> display(d) А, 1 В, 2 >>> safeAdd(d,'A', 22, unsafe=True) >>> d['A'] 22
Write a function called safeAdd(d, k, v, unsafe=False) that takes either three or four input parameters when called. Here, d is a dictionary, k is a key and v is a value. The function will add the key:value pair k:v to the dictionary d if the key k is NOT in the dictionary. If the key is in the dictionary, the function will display the current value for that key, the new value you passed into the function and ask the user if they wish to proceed and overwrite the existing value. If the optional fourth parameter is True, then just add the key:value pair without checking if the key is already present. Sample run (user input is highlighted) >>> d = {'A' :1} >>> safeAdd(d, 'B', 3} >>> display (d) А, 1 В, з >>> safeAdd (d, 'B', 2) The key B is already in the dictionary. Current value is 3. Do you want to replace this value with 2? [yes/no] yes >>> display(d) А, 1 В, 2 >>> safeAdd(d, 'A', 12) The key A is already in the dictionary. Current value is 1. Do you want to replace this value with 12? [yes/no] no >>> display(d) А, 1 В, 2 >>> safeAdd(d,'A', 22, unsafe=True) >>> d['A'] 22
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 3E
Related questions
Question
100%
![Problem 2 [Safe Add]
Write a function called safeAdd(d, k, v, unsafe=False) that takes either three or
four input parameters when called. Here, d is a dictionary, k is a key and v is a value. The
function will add the key:value pair k:v to the dictionary d if the key k is NOT in the
dictionary.
If the key is in the dictionary, the function will display the current value for that key, the
new value you passed into the function and ask the user if they wish to proceed and
overwrite the existing value.
If the optional fourth parameter is True, then just add the key:value pair without checking
if the key is already present.
Sample run (user input is highlighted)
>>> d =
{'A':1}
>>> safeAdd(d, 'B', 3}
>>> display(d)
А, 1
В, 3
>>> safeAdd(d, 'B', 2)
The key B is already in the dictionary.
Current value is 3. Do you want to replace this value with 2? [yes/no]
yes
>>> display(d)
А, 1
В, 2
>>> safeAdd(d, 'A', 12)
The key A is already in the dictionary.
Current value is 1. Do you want to replace this value with 12? [yes/no]
no
>>> display(d)
А, 1
В, 2
>>> safeAdd(d,'A', 22, unsafe=True)
>>> d[ 'A']
22](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe21a53e2-9c72-4f63-8672-f88205df8096%2Ffe95d8d1-b4d3-4a8b-b42e-38f9691ebcab%2Fgsmf3c4v_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Problem 2 [Safe Add]
Write a function called safeAdd(d, k, v, unsafe=False) that takes either three or
four input parameters when called. Here, d is a dictionary, k is a key and v is a value. The
function will add the key:value pair k:v to the dictionary d if the key k is NOT in the
dictionary.
If the key is in the dictionary, the function will display the current value for that key, the
new value you passed into the function and ask the user if they wish to proceed and
overwrite the existing value.
If the optional fourth parameter is True, then just add the key:value pair without checking
if the key is already present.
Sample run (user input is highlighted)
>>> d =
{'A':1}
>>> safeAdd(d, 'B', 3}
>>> display(d)
А, 1
В, 3
>>> safeAdd(d, 'B', 2)
The key B is already in the dictionary.
Current value is 3. Do you want to replace this value with 2? [yes/no]
yes
>>> display(d)
А, 1
В, 2
>>> safeAdd(d, 'A', 12)
The key A is already in the dictionary.
Current value is 1. Do you want to replace this value with 12? [yes/no]
no
>>> display(d)
А, 1
В, 2
>>> safeAdd(d,'A', 22, unsafe=True)
>>> d[ 'A']
22
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
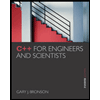
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
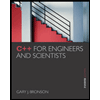
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr