Write a function that takes a match, mismatch and gap score, as well as two ungapped sequences as input and returns a Needleman-Wunsch scoring matrix with all the positions filled in. Use the function to obtain a scoring matrix for the two sequences and print the matrix. Hint: it may be easier to first initialize the matrix with first row and column of gap scores, then fill out the rest of the matrix. The max() function will return the maximum value from a list of values. For example max (1,7,3) will return 7. # Make a score matrix with these two sequences seqa = "CGTATGCTAGCTTATTTGC" seqb = "TAACTAGCGATTGCGC" # And these match, mismatch and gap penalties match = 1 mismatch = -1 gap = -2 # Answer def needleman_wunsch_full(seqa, seqb, match, mismatch, gap) : # length of two sequences n = len(seqa) m = len(seqb) # Initialize scoring matrix with zeros and gap scores for first row and column score = [0] * (n+1) for i in range(n+1): score[i] = [0] * (m+1) return score s = needleman_wunsch_full(seqa, seqb, match, mismatch, gap) plotmatrix(s)
Write a function that takes a match, mismatch and gap score, as well as two ungapped sequences as input and returns a Needleman-Wunsch scoring matrix with all the positions filled in. Use the function to obtain a scoring matrix for the two sequences and print the matrix. Hint: it may be easier to first initialize the matrix with first row and column of gap scores, then fill out the rest of the matrix. The max() function will return the maximum value from a list of values. For example max (1,7,3) will return 7. # Make a score matrix with these two sequences seqa = "CGTATGCTAGCTTATTTGC" seqb = "TAACTAGCGATTGCGC" # And these match, mismatch and gap penalties match = 1 mismatch = -1 gap = -2 # Answer def needleman_wunsch_full(seqa, seqb, match, mismatch, gap) : # length of two sequences n = len(seqa) m = len(seqb) # Initialize scoring matrix with zeros and gap scores for first row and column score = [0] * (n+1) for i in range(n+1): score[i] = [0] * (m+1) return score s = needleman_wunsch_full(seqa, seqb, match, mismatch, gap) plotmatrix(s)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Help with code
![Write a function that takes a match, mismatch and gap score, as well as two ungapped sequences as input and returns a Needleman-Wunsch scoring matrix
with all the positions filled in. Use the function to obtain a scoring matrix for the two sequences and print the matrix. Hint: it may be easier to first initialize the
matrix with first row and column of gap scores, then fill out the rest of the matrix.
The max() function will return the maximum value from a list of values. For example max(1,7,3) will return 7.
# Make a score matrix with these two sequences
seqa =
seqb
# And these match, mismatch and gap penalties
CGTATGCTAGCTTATTTGC"
"ТААСТАGCGATTGCGC"
match = 1
mismatch
-1
%3D
gар 3D -2
# Answer
def needleman_wunsch_full(seqa, seqb, match, mismatch, gap):
# length of two sequences
len(seqa)
len (seqb)
# Initialize scoring matrix with zeros and gap scores for first row and column
n
m
%3D
[0] * (n+1)
for i in range(n+1):
Score
score[i]
[0] * (m+1)
%3D
return score
needleman_wunsch_full(seqa, seqb, match, mismatch, gap)
plotmatrix(s)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F13be7c48-f046-4560-b6fd-c6b33f33e9e1%2F874ca4c5-586b-4e6e-be2e-1a74bcff0550%2F7ptokuq_processed.png&w=3840&q=75)
Transcribed Image Text:Write a function that takes a match, mismatch and gap score, as well as two ungapped sequences as input and returns a Needleman-Wunsch scoring matrix
with all the positions filled in. Use the function to obtain a scoring matrix for the two sequences and print the matrix. Hint: it may be easier to first initialize the
matrix with first row and column of gap scores, then fill out the rest of the matrix.
The max() function will return the maximum value from a list of values. For example max(1,7,3) will return 7.
# Make a score matrix with these two sequences
seqa =
seqb
# And these match, mismatch and gap penalties
CGTATGCTAGCTTATTTGC"
"ТААСТАGCGATTGCGC"
match = 1
mismatch
-1
%3D
gар 3D -2
# Answer
def needleman_wunsch_full(seqa, seqb, match, mismatch, gap):
# length of two sequences
len(seqa)
len (seqb)
# Initialize scoring matrix with zeros and gap scores for first row and column
n
m
%3D
[0] * (n+1)
for i in range(n+1):
Score
score[i]
[0] * (m+1)
%3D
return score
needleman_wunsch_full(seqa, seqb, match, mismatch, gap)
plotmatrix(s)
Expert Solution

Step 1
The given code is slightly difficult to follow, we thus give our implementation of the same in python
Step by step
Solved in 2 steps

Recommended textbooks for you
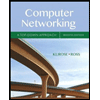
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
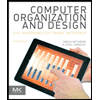
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
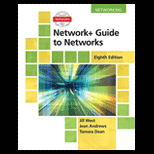
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
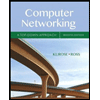
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
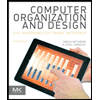
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
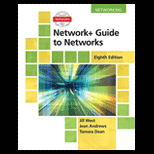
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
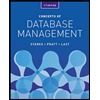
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
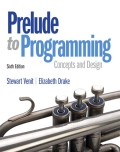
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
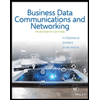
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY