write a java program for Driving license test..The local SAAQ (Société de l’Aassurance automobile du Québec) has asked you to write a program that grades the written portion of the driver’s license test. The exam booklet has 5 multiple choice questions. Here are the correct answers (answer key) for questions numbered: 1. B 2. D 3. A 4. A 5. C Write a class named DriverExam whose objects, each of which maintains the data of a candidate, and the following are the private fields (field name in bold font): • a static constant integer field (totalQs) initialized to value 5 for total number of questions. It should be used instead of hard coding
write a java program for Driving license test..The local SAAQ (Société de l’Aassurance automobile du Québec) has asked you to write a program that grades the written portion of the driver’s license test. The exam booklet has 5 multiple choice questions. Here are the correct answers (answer key) for questions numbered:
1. B 2. D 3. A 4. A 5. C
Write a class named DriverExam whose objects, each of which maintains the data of a candidate, and the following are the private fields (field name in bold font):
• a static constant integer field (totalQs) initialized to value 5 for total number of questions. It should
be used instead of hard coding because SAAQ can easily increase questions in future.
• a static constant array of characters (answer) initialized to correct answer key given above.
• a static integer field (candidates) initialized to value 0 for the number of candidates taking exam
which should be incremented upon creating an object of DriverExam for a candidate.
• a string field (name) to hold the name of candidate.
• an integer field (sin) to hold the “Social Insurance Number (S.I.N.)” of candidate,
• a character array to hold actual answers of candidate (candidateAnswer) for the questions, and
• an integer field (score) initialized to value 0 for the number of questions answered correctly by
candidate.
The class should implement (i.e., define and write code) the following methods:
• DriverExam(String name, int sin) with two parameters for name and sin (S.I.N.) of candidate,
• get_totalQs() returns the value of totalQs,
• passed() returns true if the student passed the exam (i.e., 60% or more questions are answered
correctly), or false if the student failed. The method should first compare the candidate’s answers
with answer key and update the score field, then test the score field to return the result.
• get_name() returns the name of candidate,
• get_score() returns the score of candidate (i.e., the number of questions answered correctly),
• set_candidateAnswer(int question, char answer) sets an answer in candidateAnswer array for a specified question.
Write a driver program that uses DriverExam class, creates an array of “n” objects of DriverExam (e.g., integer “n=2” for two candidates) allows them to take the exam. The exam booklets are provided to candidates, so they can directly answer the multiple-choice questions (MCQs) one by one.
Use a for loop for conducting exams of candidates (i.e., DriverExam objects), prompting each candidate. Your program should ask each candidate to enter first name, last name, S.I.N., and “answers to MCQs in a for loop”. Once a candidate completes answering, the results should be printed. Your program should call appropriate methods. Please refer to the following screen shot for details.
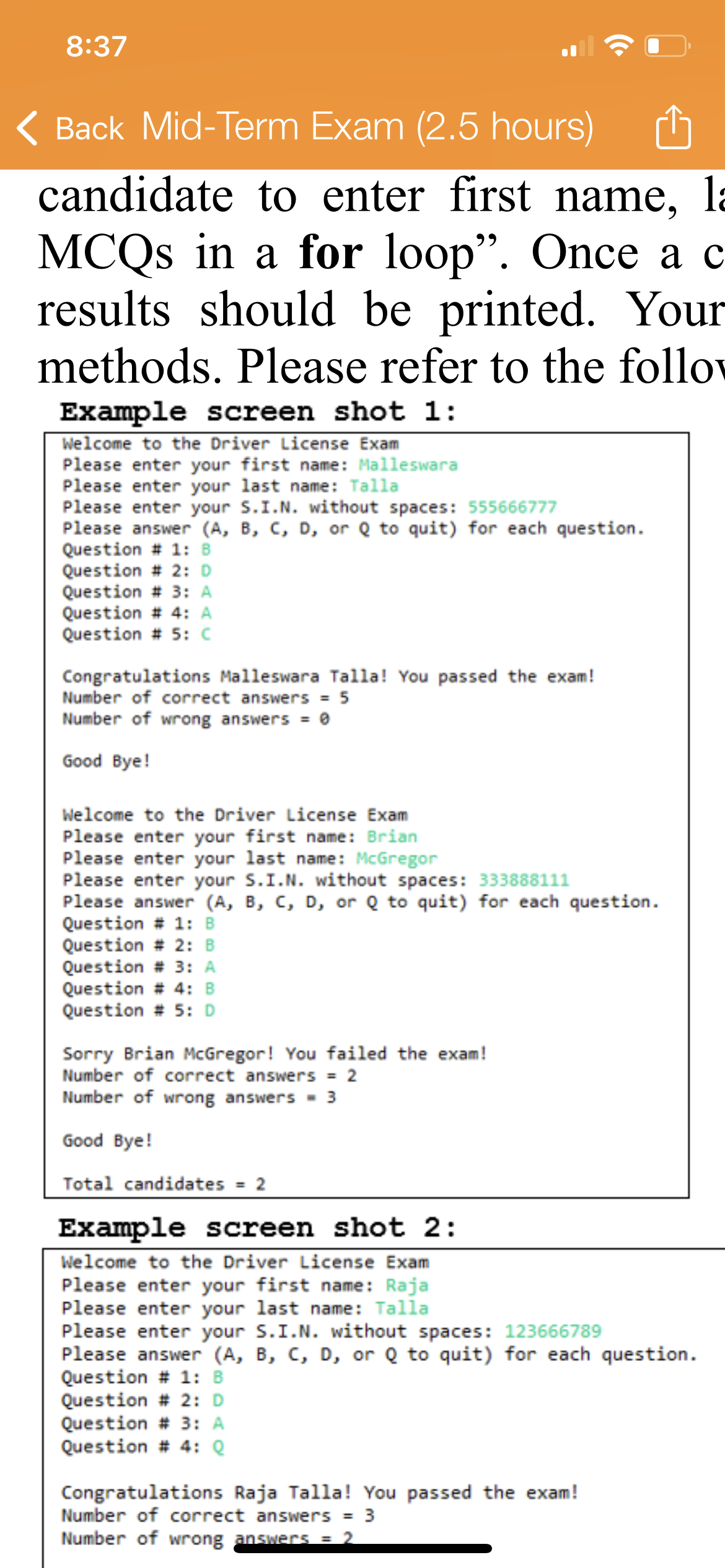
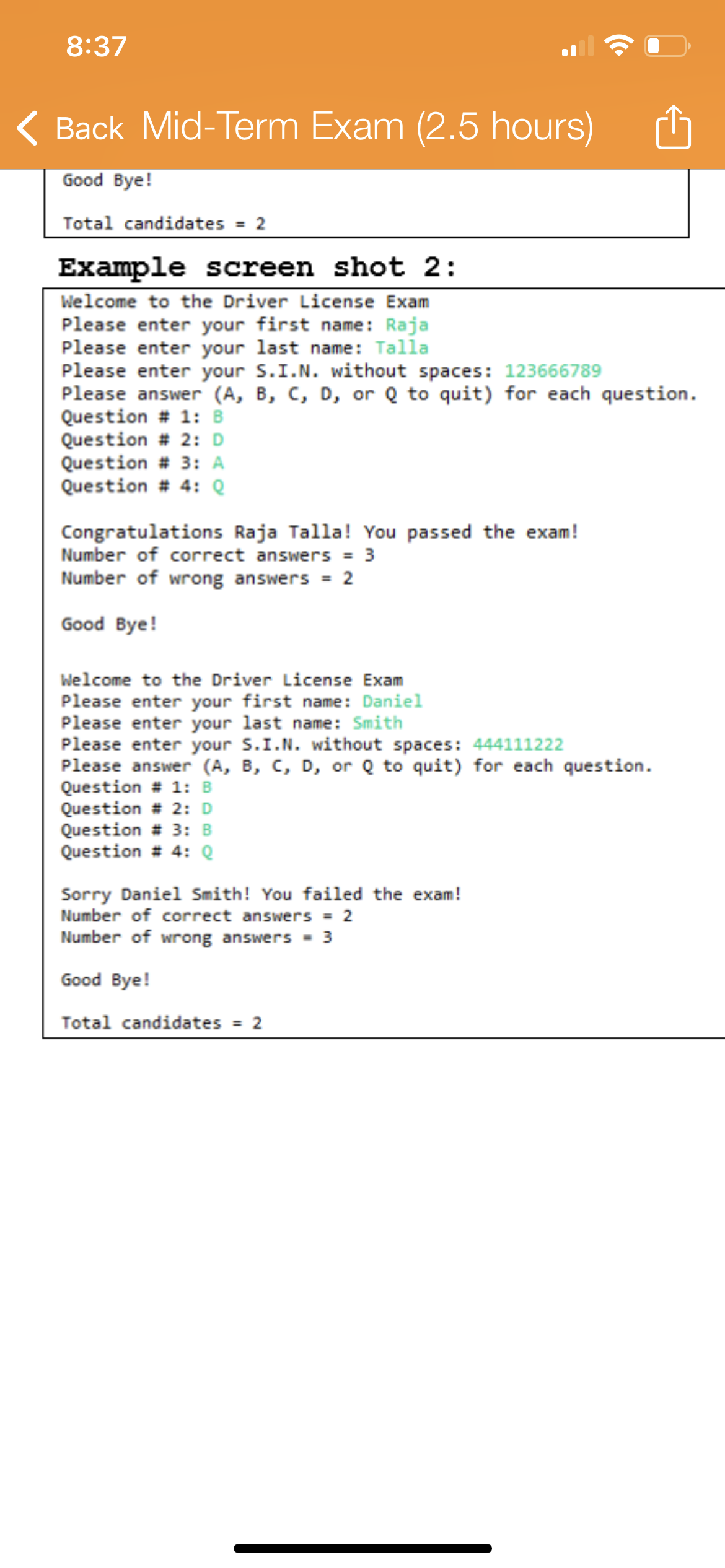

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

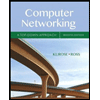
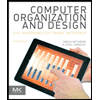
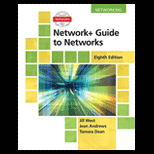
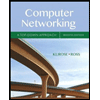
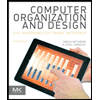
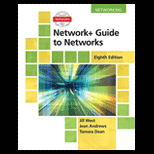
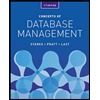
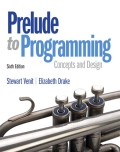
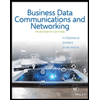