Write a Java program for Zeller’s Algorithm, which can be used to determine the day of the week for any date in the past, present, or future. The program should request the user to enter the date values (month, day, and year). Next, the program should apply the algorithm (see below), and display the appropriate day of the week. The program should loop until the user enters 0 for a month. Use the pre-test while loop with sentinel-value structure while (month!=0). In addition, validate that the month is between 0 and 12; and that day is between 1 and 31. Use the while loop structure for error checking.
Write a Java program for Zeller’s Algorithm, which can be used to determine the day of the week for any date in the past, present, or future. The program should request the user to enter the date values (month, day, and year). Next, the program should apply the algorithm (see below), and display the appropriate day of the week. The program should loop until the user enters 0 for a month. Use the pre-test while loop with sentinel-value structure while (month!=0). In addition, validate that the month is between 0 and 12; and that day is between 1 and 31. Use the while loop structure for error checking.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a Java
The program should request the user to enter the date values (month, day, and year).
Next, the program should apply the algorithm (see below), and display the appropriate day of the week.
The program should loop until the user enters 0 for a month. Use the pre-test while loop with sentinel-value structure while (month!=0).
In addition, validate that the month is between 0 and 12; and that day is between 1 and 31. Use the while loop structure for error checking.
![Write a Java program for Zeller's Algorithm, which can be used to determine the day of the week for any date
in the past, present, or future.
The program should request the user to enter the date values (month, day, and year). Next, the program should
apply the algorithm (see below), and display the appropriate day of the week. The program should loop until the
user enters 0 for a month. Use the pre-test while loop with sentinel-value structure.
In addition, validate that the month is between 0 and 12; and that day is between 1 and 31. Use the while loop
structure for error checking.
Algorithm:
The formula is:
G=([2.6M – .2 ] +K +D+ [D/4] + [C/4] – 2C) mod 7
where:
M: is the month number
K: is the day of the month
C: is the century number
D: is the year number
explanations:
M: March is considered month 1 and February is considered month 12. Therefore,
January and February are considered to be part of the previous year.
C: Is the first two digits of the year
D: Is the last two digits of the year
G: If this value is less than 0, add a value of 7 to G
You need to cast the following expression as an integer: [2.6 M – .2]
examples:
Month
Day
Year
M
K
D
76
7 (not 8)
7
4
1776
2008
4
17
1
17
11
17
20](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb27e094d-c3af-4ccc-a444-1f89e096dd09%2Fafcc4d30-579f-440f-83f6-9230752e5ba4%2F1tbozga_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write a Java program for Zeller's Algorithm, which can be used to determine the day of the week for any date
in the past, present, or future.
The program should request the user to enter the date values (month, day, and year). Next, the program should
apply the algorithm (see below), and display the appropriate day of the week. The program should loop until the
user enters 0 for a month. Use the pre-test while loop with sentinel-value structure.
In addition, validate that the month is between 0 and 12; and that day is between 1 and 31. Use the while loop
structure for error checking.
Algorithm:
The formula is:
G=([2.6M – .2 ] +K +D+ [D/4] + [C/4] – 2C) mod 7
where:
M: is the month number
K: is the day of the month
C: is the century number
D: is the year number
explanations:
M: March is considered month 1 and February is considered month 12. Therefore,
January and February are considered to be part of the previous year.
C: Is the first two digits of the year
D: Is the last two digits of the year
G: If this value is less than 0, add a value of 7 to G
You need to cast the following expression as an integer: [2.6 M – .2]
examples:
Month
Day
Year
M
K
D
76
7 (not 8)
7
4
1776
2008
4
17
1
17
11
17
20
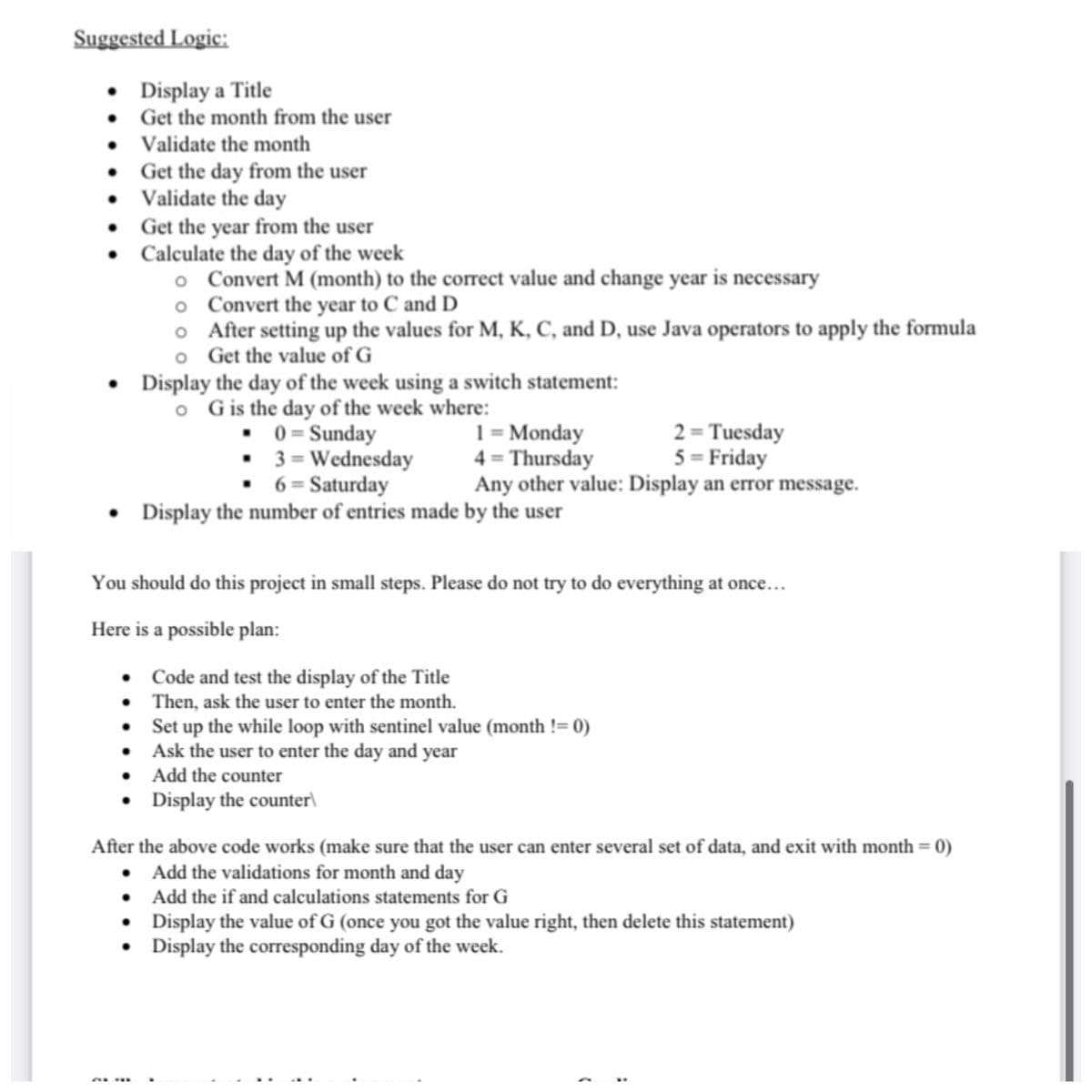
Transcribed Image Text:Suggested Logic:
• Display a Title
• Get the month from the user
• Validate the month
• Get the day from the user
• Validate the day
• Get the year from the user
Calculate the day of the week
o Convert M (month) to the correct value and change year is necessary
o Convert the year to C and D
o After setting up the values for M, K, C, and D, use Java operators to apply the formula
o Get the value of G
• Display the day of the week using a switch statement:
o Gis the day of the week where:
• 0 = Sunday
• 3 = Wednesday
• 6= Saturday
1 = Monday
4 = Thursday
Any other value: Display an error message.
2 = Tuesday
5 = Friday
• Display the number of entries made by the user
You should do this project in small steps. Please do not try to do everything at once...
Here is a possible plan:
• Code and test the display of the Title
Then, ask the user to enter the month.
Set up the while loop with sentinel value (month != 0)
Ask the user to enter the day and year
• Add the counter
• Display the counter\
After the above code works (make sure that the user can enter several set of data, and exit with month = 0)
• Add the validations for month and day
• Add the if and calculations statements for G
• Display the value of G (once you got the value right, then delete this statement)
• Display the corresponding day of the week.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
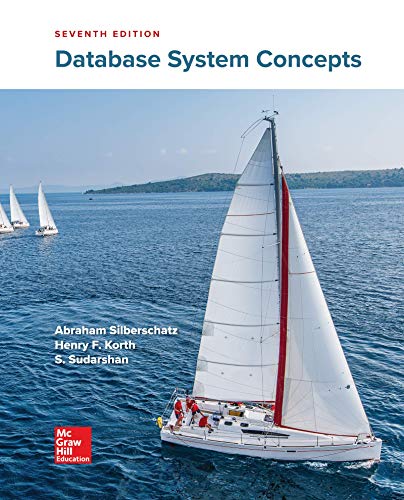
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
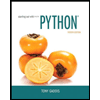
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
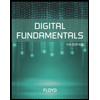
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
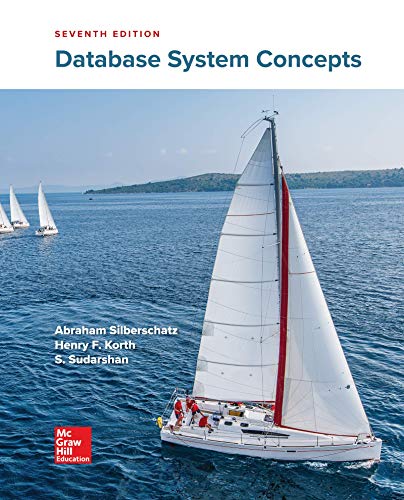
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
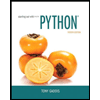
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
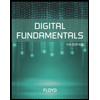
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
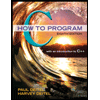
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
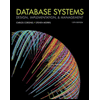
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
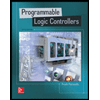
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education