Write a main class that implements our Parking office class below (main class for parking office class). Use java to write your code. The main class should implement most methods of parking office. Sample input and output should be generated on your own intuitively I have included all the codes for the other classes in our system. The parking lot office code is included as a scrrenshot.
Write a main class that implements our Parking office class below (main class for parking office class). Use java to write your code.
The main class should implement most methods of parking office.
Sample input and output should be generated on your own intuitively
I have included all the codes for the other classes in our system. The parking lot office code is included as a scrrenshot.
Parking office class
public class ParkingOffice {
String name;
String address;
String phone;
List<Customer> customers;
List<Car> cars;
List<ParkingLot> lots;
List<ParkingCharge> charges;
public ParkingOffice(){
customers = new ArrayList<>();
cars = new ArrayList<>();
lots = new ArrayList<>();
charges = new ArrayList<>();
}
public Customer register() {
Customer cust = new Customer(name,address,phone);
customers.add(cust);
return cust;
}
public Car register(Customer c,String licence, CarType t) {
Car car = new Car(c,licence,t);
cars.add(car);
return car;
}
public Customer getCustomer(String name) {
for(Customer cust : customers)
if(cust.getName().equals(name))
return cust;
return null;
}
public double addCharge(ParkingCharge p) {
charges.add(p);
return p.amount;
}
public String[] getCustomerIds(){
String[] stringArray1 = new String[5];
for(int i=0;i<5;i++){
stringArray1[i] = customers.get(i).customerID;
}
return stringArray1;
}
public String [] getPermitID () {
String[] stringArray2 = new String[5];
for(int i=0;i<5;i++){
stringArray2[i] = charges.get(i).permitID;
}
return stringArray2;
}
public List<String> getPermitIds(Customer c){
List<String> permitid = new ArrayList<String>();
for(Car car:cars){
if(car.owner == c.customerId)
permitid.add(car.permit);
}
return permitid;
}
}
Car class
import java.time.LocalDate;
enum CarType {
COMPACT, SUV;
}
public class Car {
//declaring instance valriables
public String permit;
private LocalDate permitExpiration;
private String license;
private CarType type;
public String owner;
Car(Customer c, String licence, CarType t) {
}
Car() {
}
//getters and setters
public String getPermit() {
return permit;
}
public void setPermit(String permit) {
this.permit = permit;
}
public LocalDate getPermitExpiration() {
return permitExpiration;
}
public void setPermitExpiration(LocalDate permitExpiration) {
this.permitExpiration = permitExpiration;
}
public String getLicense() {
return license;
}
public void setLicense(String license) {
this.license = license;
}
public CarType getType() {
return type;
}
public void setType(CarType type) {
this.type = type;
}
public String getOwner() {
return owner;
}
public void setOwner(String owner) {
this.owner = owner;
}
//toString method
@Override
public String toString() {
return "Car [permit=" + permit + ", permitExpiration=" + permitExpiration + ", license=" + license + ", type="
+ type + ", owner=" + owner + "]";
}
@Override
public boolean equals(Object car) {
if (car == this) {
return true;
}
if (!(car instanceof Car)) {
return false;
}
Car c = (Car) car;
return ((Car) car).license.equals(c);
}
@Override
public int hashCode() {
return Integer.parseInt(this.license);
}
}
Customer Class
import java.util.ArrayList;
import java.util.List;
public class Customer {
//declaring instance variables
public String customerId;
public String name;
public String address;
public String phoneNumber;
//decaring additional variable
private final List<Car> cars=new ArrayList<>();
String customer;
String customerID;
Customer(String name, String address, String phone) {
}
public Car register(String license, CarType type) {
Car c=new Car();
c.setLicense(license);
c.setType(type);
cars.add(c); //add this new Car to cars List of customer
return c;
}
//getter method for Car
public List<Car> getCars() {
return cars;
}
//getters and setters
public String getCustomerId() {
return customerId;
}
public void setCustomerId(String customerId) {
this.customerId = customerId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
//toString method
@Override
public String toString() {
return "Customer [customerId=" + customerId + ", name=" + name + ", address=" + address + ", phoneNumber="
+ phoneNumber + "]";
}
}
Parking Charge Class
class Instant{
public int hours;
public int minutes;
}
// ParkingCharge class Definition
class ParkingCharge{
public String permitID;
public String lotID;
public double amount;
public Instant incurredTime = new Instant();
ParkingCharge (String permitID, String lotID, double amount) {
}
ParkingCharge() {
}
// toString class definition
@Override
public String toString(){
String permitDetails = "\nPermit ID: "+permitID;
String lotDetails = "\nLot ID: "+lotID;
String incurredDetails="\nDuration: "+incurredTime.hours+" HH "+incurredTime.minutes+" MM";
// lets say 1 minutes of parking charge is 1 dollar
amount = incurredTime.hours*60 + incurredTime.minutes;
String amountDetails = "\nParking Charge: "+amount;
return permitDetails+lotDetails+incurredDetails+amountDetails;
}
}
![dasses
public class ParkingLot {
//declaring instance variables
private String lotId;
private String address;
private int capacity;
//decalring additional variables
private List<Car> cars=new ArrayList<> ();
public void entry (Car c) {
cars.add (c);
public void exit (Car c) {
cars.remove (c) ;
public String getLotId () {
return lotId;
public void setLotId (String lotId) {
this.lotId = lotId;
public String getAddress () {
return address;
public void setAddress (String address) {
this.address = address;
public int getCapacity () {
return capacity;
}
public void setCapacity (int capacity) {
this.capacity - саpаcity;
public List<Car> getCars () {
return cars;
}
public void setCars (List<Car> cars) {
this.cars = cars;
Coverride
public String tostring () {
return "ParkingLot [1otId=" + lotId + ", address=" + address + ", capacity=" + capacity + ", cars=" + cars
+ "]";
P P OP O](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0148e73e-0d28-4d98-a412-189d898de2e8%2F7ad0b837-ecec-4345-9e4c-8913dda17ca9%2Fn27vjgn_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

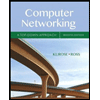
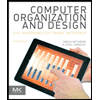
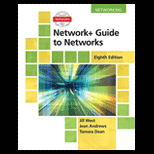
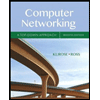
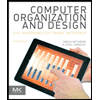
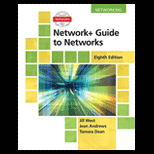
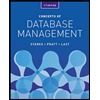
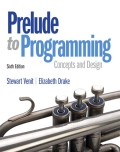
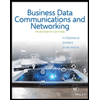