Write a method called prioritizeQueue to give priority for vaccination for elderly persons. The method is to be in a class called VaccinationQueue and has two parameters q1 and q2 type ArrayQueue. The q1 and q2 data are of type Integer. Assume that initially q1 is not empty and q2 is empty. The method will insert from q1 those elements towards the beginning of the queue (front) q2 whose data is greater than or equal to 45 and will insert those elements whose data is less than 45 and greater than or equal to 18 towards the end (rear) of q2. Any data item less than 18 of q1 will not be inserted in q2. It returns the number of elements added to q2. Class ArrayQueue and all its methods including iterator are available for use. You can also create temporary queues. You are not allowed to use arrays or any other data structure. Example: Before method call: front rear q1: 14 25 50 70 35 45 19 10 21 q2: empty After method call: front rear q1: 14 25 50 70 35 45 19 10 21 q2: 50 70 45 25 35 19 21 It will return 7 as 7 elements are added from q1 to q2. Method heading: public class VaccinationQueue { public static int prioritizeQueue(ArrayQueue q1, ArrayQueue q2) {
Write a method called prioritizeQueue to give priority for vaccination for elderly persons. The method is to be in a class called VaccinationQueue and has two parameters q1 and q2 type ArrayQueue. The q1 and q2 data are of type Integer. Assume that initially q1 is not empty and q2 is empty. The method will insert from q1 those elements towards the beginning of the queue (front) q2 whose data is greater than or equal to 45 and will insert those elements whose data is less than 45 and greater than or equal to 18 towards the end (rear) of q2. Any data item less than 18 of q1 will not be inserted in q2. It returns the number of elements added to q2.
Class ArrayQueue and all its methods including iterator are available for use. You can also create temporary queues. You are not allowed to use arrays or any other data structure.
Example:
Before method call:
front rear
q1: 14 25 50 70 35 45 19 10 21
q2: empty
After method call:
front rear
q1: 14 25 50 70 35 45 19 10 21
q2: 50 70 45 25 35 19 21
It will return 7 as 7 elements are added from q1 to q2.
Method heading:
public class VaccinationQueue
{
public static int prioritizeQueue(ArrayQueue<Integer> q1,
ArrayQueue<Integer> q2)
{
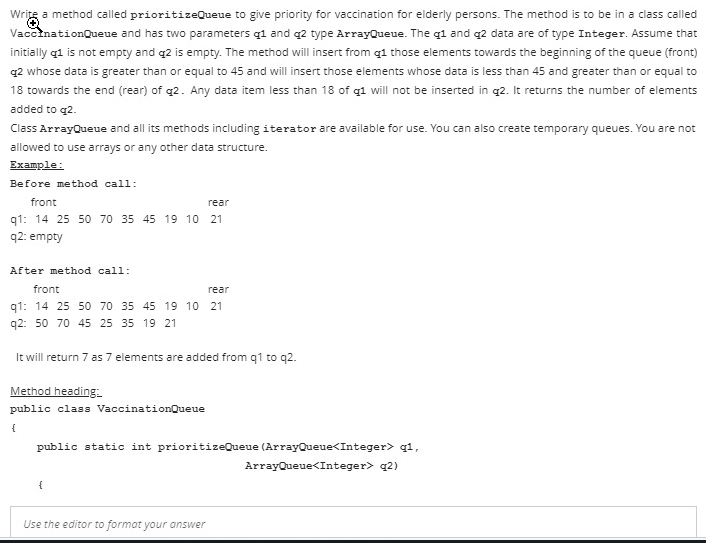

Step by step
Solved in 2 steps

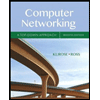
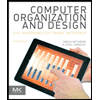
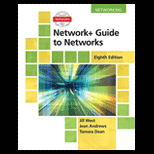
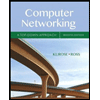
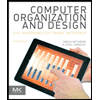
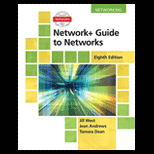
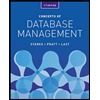
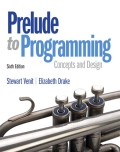
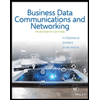