Write a program in c++ language that simulates a magic square using 3 one- dimensional parallel arrays of integer type. Each one of the arrays corresponds to a row of the magic square. The program ask the user to enter the values of the magic square row by row and informs the user if the grid a magic square or not. The Lo Shu Magic Square is a grid with 3 rows and 3 columns. It has the following properties: - the grid contains the numbers 1-9 exactly - the sum of each row, each column and each diagonal all add up to the same number. Input for this project - values of the grid(row by row) Output for this project - whether or not the grid is magic square Global constants - const int ROWS = 3; - const int COLS = 3; -const int MIN = 1; -const int MAX = 9; Use following functions: - void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and as a size as arguments and fill the arrays out with values entered by the user. - void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - bool isMagicsquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) accepts 3 int arrays and a size as arguments and returns true if all the requirements of a magic square are met. Otherwise, it returns false. - bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int mon, int max) accepts 3 int arrays, a size, a min and a max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false. - bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max) accepts 3 int arrays, a size, a min and a max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false. -bool checkRowSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each of the rows are equal. Otherwise, it returns false. - bool checkColSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each column are equal. Otherwise, it returns false. - bool checkDiagSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each of the array’s diagonals are equal. Otherwise, it returns false. The output should be exactly the same as the picture below and the program should be coded in c++ language. Make sure to include all of those functions
Write a program in c++ language that simulates a magic square using 3 one- dimensional parallel arrays of integer type. Each one of the arrays corresponds to a row of the magic square. The program ask the user to enter the values of the magic square row by row and informs the user if the grid a magic square or not. The Lo Shu Magic Square is a grid with 3 rows and 3 columns. It has the following properties: - the grid contains the numbers 1-9 exactly - the sum of each row, each column and each diagonal all add up to the same number. Input for this project - values of the grid(row by row) Output for this project - whether or not the grid is magic square Global constants - const int ROWS = 3; - const int COLS = 3; -const int MIN = 1; -const int MAX = 9; Use following functions: - void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and as a size as arguments and fill the arrays out with values entered by the user. - void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - bool isMagicsquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) accepts 3 int arrays and a size as arguments and returns true if all the requirements of a magic square are met. Otherwise, it returns false. - bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int mon, int max) accepts 3 int arrays, a size, a min and a max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false. - bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max) accepts 3 int arrays, a size, a min and a max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false. -bool checkRowSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each of the rows are equal. Otherwise, it returns false. - bool checkColSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each column are equal. Otherwise, it returns false. - bool checkDiagSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each of the array’s diagonals are equal. Otherwise, it returns false. The output should be exactly the same as the picture below and the program should be coded in c++ language. Make sure to include all of those functions
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write a program in c++ language that simulates a magic square using 3 one- dimensional parallel arrays of integer type. Each one of the arrays corresponds to a row of the magic square. The program ask the user to enter the values of the magic square row by row and informs the user if the grid a magic square or not. The Lo Shu Magic Square is a grid with 3 rows and 3 columns. It has the following properties:
- the grid contains the numbers 1-9 exactly
- the sum of each row, each column and each diagonal all add up to the same number.
Input for this project
- values of the grid(row by row)
Output for this project
- whether or not the grid is magic square
Global constants
- const int ROWS = 3;
- const int COLS = 3;
-const int MIN = 1;
-const int MAX = 9;
Use following functions:
- void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and as a size as arguments and fill the arrays out with values entered by the user.
- void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size)
- bool isMagicsquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) accepts 3 int arrays and a size as arguments and returns true if all the requirements of a magic square are met. Otherwise, it returns false.
- bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int mon, int max) accepts 3 int arrays, a size, a min and a max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false.
- bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max) accepts 3 int arrays, a size, a min and a max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false.
-bool checkRowSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each of the rows are equal. Otherwise, it returns false.
- bool checkColSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each column are equal. Otherwise, it returns false.
- bool checkDiagSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size): accepts 3 int arrays and a size as arguments and returns true if the sum of the values in each of the array’s diagonals are equal. Otherwise, it returns false.
The output should be exactly the same as the picture below and the program should be coded in c++ language. Make sure to include all of those functions
![// Function prototypes
bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRange(int`arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max);
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRowSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkColsum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
void showArray (int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
int main()
{
/* Define a Lo Shu Magic Square using 3 parallel arrays corresponding to'each row
of the grid
int arrayRowl[COLS], arrayRow2 [COLst], arrayRow3[COLS];
// Your code goes here
return 0;
// Function definitions go here](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F44b0f74e-c602-4978-a736-701fbc7d130e%2F35d8d00c-8a7d-465c-9e53-cbdfd32ea050%2Fwy2fox6_processed.jpeg&w=3840&q=75)
Transcribed Image Text:// Function prototypes
bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRange(int`arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max);
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRowSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkColsum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
void showArray (int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
int main()
{
/* Define a Lo Shu Magic Square using 3 parallel arrays corresponding to'each row
of the grid
int arrayRowl[COLS], arrayRow2 [COLst], arrayRow3[COLS];
// Your code goes here
return 0;
// Function definitions go here

Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Recommended textbooks for you
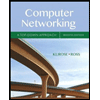
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
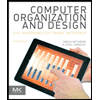
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
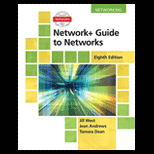
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
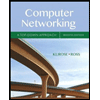
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
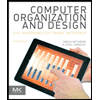
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
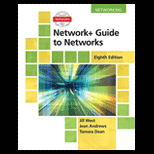
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
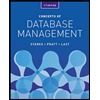
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
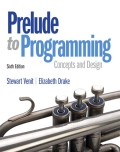
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
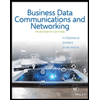
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY