Write a program in C++ that reads HAND_SIZE cards from the user, then analyzes the cards and prints out the type of poker hand that they represent. (HAND_SIZE will be a global constant, typically 5, but your program must still work if it is set to something other than 5.) Poker hands are categorized according to the following hand-types: Straight flush, four of a kind, full house, straight, flush, three of a kind, two pairs, pair, high card. To simplify the program we will ignore card suits, and face cards. The values that the user inputs will be integer values from LOWEST_NUM to HIGHEST_NUM. (These will be global constants. We'll use LOWEST_NUM = 2 and HIGHEST_NUM = 9, but your program must work if these are set to something different.) When your program runs it should start by collecting HAND_SIZE integer values from the user and placing the integers into an array that has HAND_SIZE elements. It might look like this: Enter 5 numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 3 (This is a pair, since there are two eights) No input validation is required for this assignment. You can assume that the user will always enter valid data (numbers between LOWEST_NUM and HIGHEST_NUM). Since we are ignoring card suits there won't be any flushes. Your program should be able to recognize the following hand categories, listed from least valuable to most valuable: Hand Type Description Example High Card There are no matching cards, and the hand is not a straight 2, 5, 3, 8, 7 Pair Two of the cards are identical 2, 5, 3, 5, 7 Two Pair Two different pairs 2, 5, 3, 5, 3 Three of a kind Three matching cards 5, 5, 3, 5, 7 Straight 5 consecutive cards 3, 5, 6, 4, 7 Full House A pair and three of a kind 5, 7, 5, 7, 7 Four of a kind Four or more matching cards 2, 5, 5, 5, 5 A note on straights: a hand is a straight regardless of the order. So the values 3, 4, 5, 6, 7 represent a straight, but so do the values 7, 4, 5, 6, 3. Also, a straight requires just 5 consecutive cards, even if HAND_SIZE is larger than 5. Your program should read in HAND_SIZE values and then print out the appropriate hand-type. If a hand matches more than one description, the program should print out the most valuable hand type.
Write a program in C++ that reads HAND_SIZE cards from the user, then analyzes the cards and prints out the type of poker hand that they represent. (HAND_SIZE will be a global constant, typically 5, but your program must still work if it is set to something other than 5.)
Poker hands are categorized according to the following hand-types: Straight flush, four of a kind, full house, straight, flush, three of a kind, two pairs, pair, high card.
To simplify the program we will ignore card suits, and face cards. The values that the user inputs will be integer values from LOWEST_NUM to HIGHEST_NUM. (These will be global constants. We'll use LOWEST_NUM = 2 and HIGHEST_NUM = 9, but your program must work if these are set to something different.) When your program runs it should start by collecting HAND_SIZE integer values from the user and placing the integers into an array that has HAND_SIZE elements. It might look like this:
Enter 5 numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 3
(This is a pair, since there are two eights)
No input validation is required for this assignment. You can assume that the user will always enter valid data (numbers between LOWEST_NUM and HIGHEST_NUM).
Since we are ignoring card suits there won't be any flushes. Your program should be able to recognize the following hand categories, listed from least valuable to most valuable:
Hand Type | Description | Example |
High Card | There are no matching cards, and the hand is not a straight | 2, 5, 3, 8, 7 |
Pair | Two of the cards are identical | 2, 5, 3, 5, 7 |
Two Pair | Two different pairs | 2, 5, 3, 5, 3 |
Three of a kind | Three matching cards | 5, 5, 3, 5, 7 |
Straight | 5 consecutive cards | 3, 5, 6, 4, 7 |
Full House | A pair and three of a kind | 5, 7, 5, 7, 7 |
Four of a kind | Four or more matching cards | 2, 5, 5, 5, 5 |
A note on straights: a hand is a straight regardless of the order. So the values 3, 4, 5, 6, 7 represent a straight, but so do the values 7, 4, 5, 6, 3. Also, a straight requires just 5 consecutive cards, even if HAND_SIZE is larger than 5.
Your program should read in HAND_SIZE values and then print out the appropriate hand-type. If a hand matches more than one description, the program should print out the most valuable hand type.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

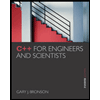
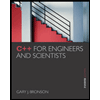