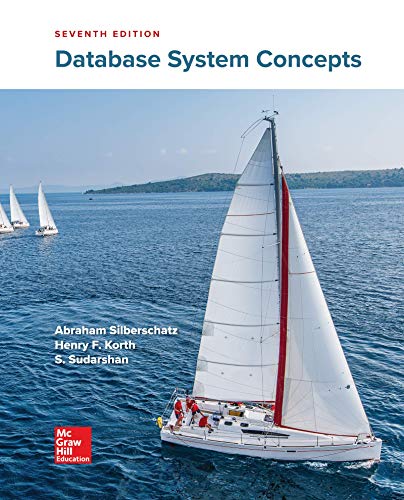
Concept explainers
Write a program that accepts two four-digit binary numbers, converts them to decimal values, adds them together, and prints both the decimal values and the result of the addition.
Requirements:
- Functionality. (80pts)
- No Syntax Errors. (80pts*)
- *Code that cannot be compiled due to syntax errors is nonfunctional code and will receive no points for this entire section.
- Clear and Easy-To-Use Interface. (10pts)
- Users should easily understand what the program does and how to use it.
- Users should be prompted for input and should be able to enter data easily.
- Users should be presented with output after major functions, operations, or calculations.
- All the above must apply for full credit.
- Users must be able to enter a 4-bit binary number in some way. (10pts)
- No error checking is needed here and you may assume that users will only enter 0’s and 1’s, and they will only enter 4 bits.
- Binary to Decimal Conversion (50pts)
- You may assume that users will only give numbers that add up to 15.
- See the section Hint for more details.
- Adding Values (10pts)
- Both decimal values must be added together and printed out.
- You may NOT use Integer.parseInt(<<STRING>>, 2) or any automatic converter (80pts*).
- *The use of specifically Integer.parseInt(<<STRING>>,2) will result in a 0 for this entire section.
- You may use Integer.parseInt(<<STRING>>).
- Coding Style. (10pts)
- Readable Code
- Meaningful identifiers for data and methods.
- Proper indentation that clearly identifies statements within the body of a class, a method, a branching statement, a loop statement, etc.
- All the above must apply for full credit.
- Comments. (10pts)
- Your name in the file. (5pts)
- At least 5 meaningful comments in addition to your name. These must describe the function of the code it is near. (5pts)
PLEASE WRITE IN JAVA USING ECLIPSE IED
Hint:
A simple way to convert a binary value to a decimal value.
- Multiply each binary digit by its corresponding base 2 placement value.
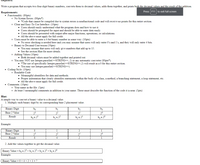

ANSWER:
we can convert binary to decimal in java using Integer.parseInt() and a custom method getDecimal().
Integer.parseInt(String s) takes 1 argument:
then Integer.ParseInt() will convert String of binary into integer and return it.
we will then convert this integer into decimal digit using a method int getDecimal(int binary).
code:
import java.util.*;
public class Main
{
//defining getDecimal method.
public static int getDecimal(int binary){
int decimal = 0;
int n = 0;
while(true){
if(binary == 0){
break;
} else {
int temp = binary%10;
decimal += temp*Math.pow(2, n);
binary = binary/10;
n++;
}
}
return decimal;
}
public static void main(String[] args) {
//taking 2 binary numbers as an input
Scanner sc= new Scanner(System.in);
System.out.println("Enter a 4-bit binary number");
String str1= sc.nextLine();
System.out.println("Enter another 4-bit binary number");
String str2= sc.nextLine();
//converting binary string number into binary integers.
int bin1=Integer.parseInt(str1);
int bin2=Integer.parseInt(str2);
// converting binary digits into decimal digits:
int dec1=getDecimal(bin1);
int dec2=getDecimal(bin2);
//printing out the decimal digits and their sum:
System.out.println("The first number is "+ dec1);
System.out.println("The second number is "+ dec2);
System.out.println("Added together is "+ (dec1+dec2));
}
}
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- please help with this questionarrow_forwardHow is an application "debugged" in the context of code creation?arrow_forwardOpenGL Programming Help (please provide a screenshot that it works) Write a program that creates a three-dimensional figure of the University logo "IU" that animates. The In response to the menu selection, one of the two letters will spin about a vertical axis. When the user clicks on the right mouse button and selects the menu option to spin the “U”, the “I” should stop spinning and the “U” should spin in the same manner. At no point should both letters spin at the same time. This should use a display listarrow_forward
- Calculating the area of a rectangleWrite a Linux Bash shell script to prompt a user for two numbers, representing width and height of a rectangle and to then calculate and display the value of the area of the rectangle.The user should be prompted to input the data both in square centimetres and in square inches ( 1 inch = 2.54 cm).The user should be prompted to display the area either in square metres and in square inchesThe user should have the opportunity to either quit the program or input different data. use #!/bin/basharrow_forwardObject-Oriented Programming OvalDraw Plus (Java code) Summary: Create a graphical application with Java that that draws an oval Prerequisites: Java, VS Code, and Terminal Create a graphical Java application that runs on Microsoft Windows and MacOS that draws an oval centered in the main application window when the programming starts. The oval should automatically resize and reposition itself when the window is resized. In this activity you will start with our OvalDraw application in our sample code, review the code, and then make incremental improvements to the application. Be sure to make the resulting application uniquely your own by adding standard comments at the top application (i.e. your name, class, etc.), changing the names of variables, and adding small features. Finally, be sure to save your work as you will often be asked to submit it as part of an assignment. Be sure to review the example OvalDraw project in the Java section of our example code repository. Requirement 1:…arrow_forwardIdentify the correct statement. Group of answer choices An array is passed to a method by passing the array's values A method cannot modify the elements of an array argument An array is converted to another data type and passed to a method An array is passed to a method by passing a reference to the arrayarrow_forward
- C++ Nested loops: Print seats. Given numRows and numColumns, print a list of all seats in a theater. Rows are numbered, columns lettered, as in 1A or 3E. Print a space after each seat, including after the last. Ex: numRows = 2 and numColumns = 3 prints:1A 1B 1C 2A 2B 2C #include <iostream>using namespace std; int main() { int numRows; int numColumns; int currentRow; int currentColumn; char currentColumnLetter; cin >> numRows; cin >> numColumns; /* Your solution goes here */ cout << endl; return 0;}arrow_forward9. Please help me answer this engineering questionarrow_forwardThe code box below defines a variable route as a list of directions to navigate a maze. Each instruction is one of the following four basic commands: higher move one step in the positive y direction • lower. move one step in the negative y direction • left: move one step in the negative x direction • right: move one step in the positive x direction Define a function step that takes two arguments, a location (as a tuple of x and y coordinates) and an instruction (higher, lower, left, right) as a string. Given the provided location, it should return the new location (as a tuple of x and y coordinates) when following the specified instruction. If the instruction is invalid, the old location should be returned. Use the function step to determine the final position when starting from the point (0, -4) and following all instructions in the list route. Assign this final position to the variable final_point.arrow_forward
- using Java GUI Interface languagearrow_forwardProject Description: The goal of this project is to write a program that can read data from any publicly available IoT source device and display it clearly to the user. The program will need to be written in Python (or any other programming language of your choice) and will need to include the following features: 1. Data Reading: The program should be able to read data from any publicly available IoT source device, such as a camera or any other sensor. The type of device is up to you, but they should be able to provide a valid URL for the device. The program should read the data from the device at regular intervals and store it in a data structure. 2. Data Display: The program should display the data that it reads from the IoT source device in a clear and easy-to-read format. The display could be in the form of a text-based output or a graphical interface, depending on your preference. The display should include information about the source sensor, as well as the data that was read…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
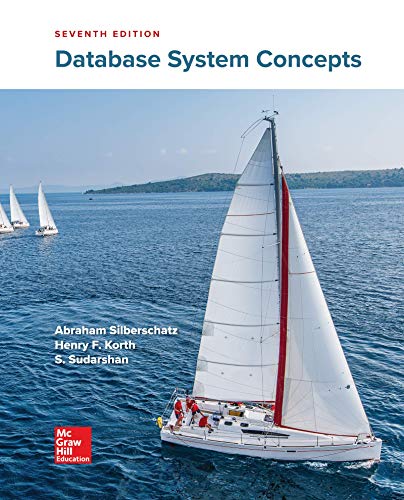
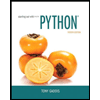
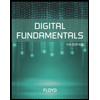
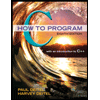
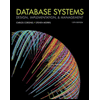
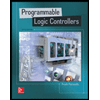