Write a program that allows a user to input the directory name and perform the following file operations. • Create a subdirectory with the specified name. • Delete a subdirectory with the specified name • Go to the specified director . Retrieve the current working directory.
Write a program that allows a user to input the directory name and perform the following file operations. • Create a subdirectory with the specified name. • Delete a subdirectory with the specified name • Go to the specified director . Retrieve the current working directory.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
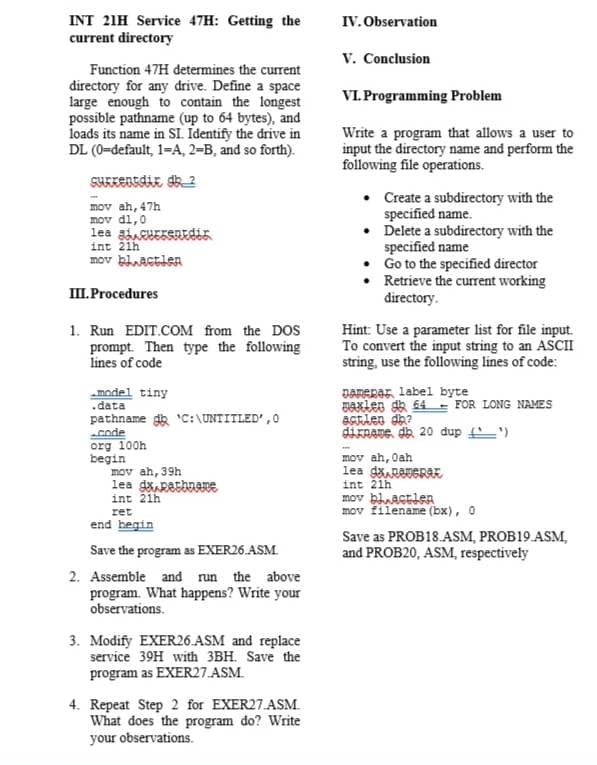
Transcribed Image Text:INT 21H Service 47H: Getting the
current directory
Function 47H determines the current
directory for any drive. Define a space
large enough to contain the longest
possible pathname (up to 64 bytes), and
loads its name in SI. Identify the drive in
DL (0-default, 1-A, 2-B, and so forth).
SHAKEBEdik d
mov ah, 47h
mov d1,0
lea
int 21h
mov bl.actlen
CHEESEDÍK
III. Procedures
1. Run EDIT.COM from the DOS
prompt. Then type the following
lines of code
model tiny
.data
pathname d 'C:\UNTITLED',0
code
org 100h
begin
mov ah, 39h
lea dx.pathname
int 21h
ret
end begin
Save the program as EXER26.ASM
2. Assemble and run the above
program. What happens? Write your
observations.
3. Modify EXER26.ASM and replace
service 39H with 3BH. Save the
program as EXER27.ASM.
4. Repeat Step 2 for EXER27.ASM.
What does the program do? Write
your observations.
IV. Observation
V. Conclusion
VI. Programming Problem
Write a program that allows a user to
input the directory name and perform the
following file operations.
•
•
•
.
Create a subdirectory with the
specified name.
Delete a subdirectory with the
specified name
Go to the specified director
Retrieve the current working
directory.
Hint: Use a parameter list for file input.
To convert the input string to an ASCII
string, use the following lines of code:
namenar label byte
maxien d 64
FOR LONG NAMES
actlen db?
dirname, do 20 dup (¹)
mov ah, Oah
lea daeRAK
int 21h
mov blactlen
mov filename (bx), 0
Save as PROB18.ASM, PROB19 ASM,
and PROB20, ASM, respectively
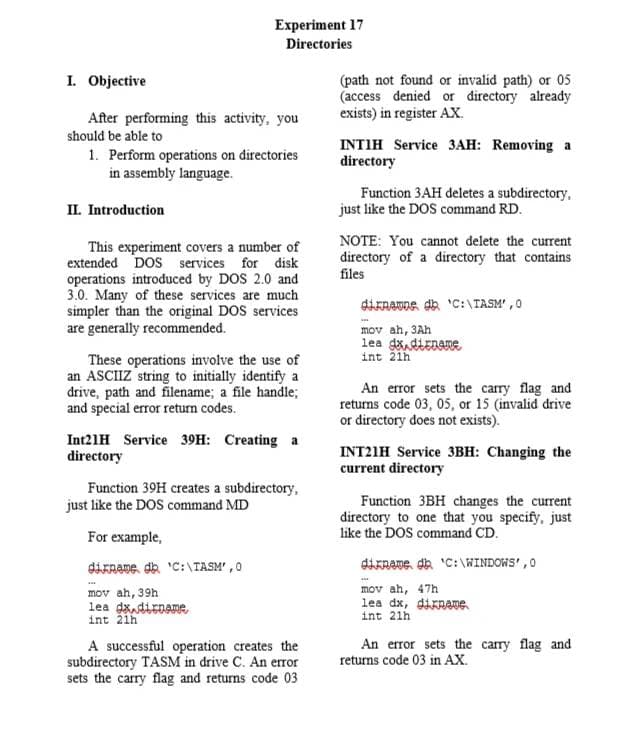
Transcribed Image Text:Experiment 17
Directories
I. Objective
After performing this activity, you
should be able to
1. Perform operations on directories
in assembly language.
II. Introduction
This experiment covers a number of
extended DOS services for disk
operations introduced by DOS 2.0 and
3.0. Many of these services are much
simpler than the original DOS services
are generally recommended.
These operations involve the use of
an ASCIIZ string to initially identify a
drive, path and filename; a file handle;
and special error return codes.
Int21H Service 39H: Creating a
directory
Function 39H creates a subdirectory,
just like the DOS command MD
For example,
diname, dh 'C:\TASM',0
mov ah, 39h
lea dx dirname
int 21h
A successful operation creates the
subdirectory TASM in drive C. An error
sets the carry flag and returns code 03
(path not found or invalid path) or 05
(access denied or directory already
exists) in register AX.
INTIH Service 3AH: Removing a
directory
Function 3AH deletes a subdirectory.
just like the DOS command RD.
NOTE: You cannot delete the current
directory of a directory that contains
files
dinamos dh 'C:\TASM',0
mov ah, 3Ah
lea dx.diename
int 21h
An error sets the carry flag and
returns code 03, 05, or 15 (invalid drive
or directory does not exists).
INT21H Service 3BH: Changing the
current directory
Function 3BH changes the current
directory to one that you specify, just
like the DOS command CD.
dirname, dh 'C:\WINDOWS', 0
mov ah, 47h
lea dx, diname.
int 21h
An error sets the carry flag and
returns code 03 in AX.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
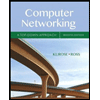
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
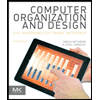
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
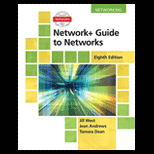
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
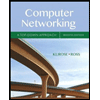
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
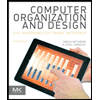
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
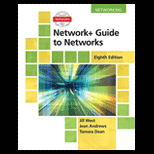
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
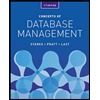
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
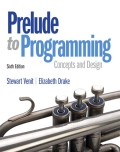
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
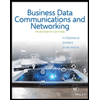
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY