Write a program that calculates the monthly pay for a salesperson. A person's pay is equal to his or her salary, plus a service bonus (if the person qualifies), plus a commission (if the person qualifies). 1.) Call a function named: getinfo() - The function prompts the user for an employee's salary, monthly sales, and number of years employed. (see output) Enter your monthly salary: 2000 Enter your sales for this month: 8500 Enter the number of years employed: // user enters 2000 // user enters 8500 // user enters 6 6 The input values are read and held in variables in main(). Note: How can a function get more than one value back to main()? 2.) Call a function named calcServiceBonus() - The function calculates the employee's service bonus based on years employed. o If the employee has worked for less than 5 years, there is no service bonus. o However, if an employee has worked at least 5 years, the employee receives a bonus, which is equal to the number of years employed multiplied by $25. The service bonus is returned to main(). 3.) Call a function named calcCommission() The function calculates the employee's commission based on the person's monthly sales. o If the sales amount is at least $5,000, the commission is equal to the sales amount multiplied by 6%. o Otherwise, no commission is paid. o The commission is returned to main(). 4.) Call a function named calcPay() The function calculates the total pay for the employee. The total pay is equal to the monthly salary, plus the service bonus, plus the commission. - The total pay is returned to main(). /* output: Salary: 2000.00 150.00 5.) Call a function named displayPay() The function displays the employee's total pay. Service Bonus: Commission: 510.00 Total Pay: 2660.00 6.) Make sure to format the output as shown.
Write a program that calculates the monthly pay for a salesperson. A person's pay is equal to his or her salary, plus a service bonus (if the person qualifies), plus a commission (if the person qualifies). 1.) Call a function named: getinfo() - The function prompts the user for an employee's salary, monthly sales, and number of years employed. (see output) Enter your monthly salary: 2000 Enter your sales for this month: 8500 Enter the number of years employed: // user enters 2000 // user enters 8500 // user enters 6 6 The input values are read and held in variables in main(). Note: How can a function get more than one value back to main()? 2.) Call a function named calcServiceBonus() - The function calculates the employee's service bonus based on years employed. o If the employee has worked for less than 5 years, there is no service bonus. o However, if an employee has worked at least 5 years, the employee receives a bonus, which is equal to the number of years employed multiplied by $25. The service bonus is returned to main(). 3.) Call a function named calcCommission() The function calculates the employee's commission based on the person's monthly sales. o If the sales amount is at least $5,000, the commission is equal to the sales amount multiplied by 6%. o Otherwise, no commission is paid. o The commission is returned to main(). 4.) Call a function named calcPay() The function calculates the total pay for the employee. The total pay is equal to the monthly salary, plus the service bonus, plus the commission. - The total pay is returned to main(). /* output: Salary: 2000.00 150.00 5.) Call a function named displayPay() The function displays the employee's total pay. Service Bonus: Commission: 510.00 Total Pay: 2660.00 6.) Make sure to format the output as shown.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section: Chapter Questions
Problem 3PP
Related questions
Question
Program in C++
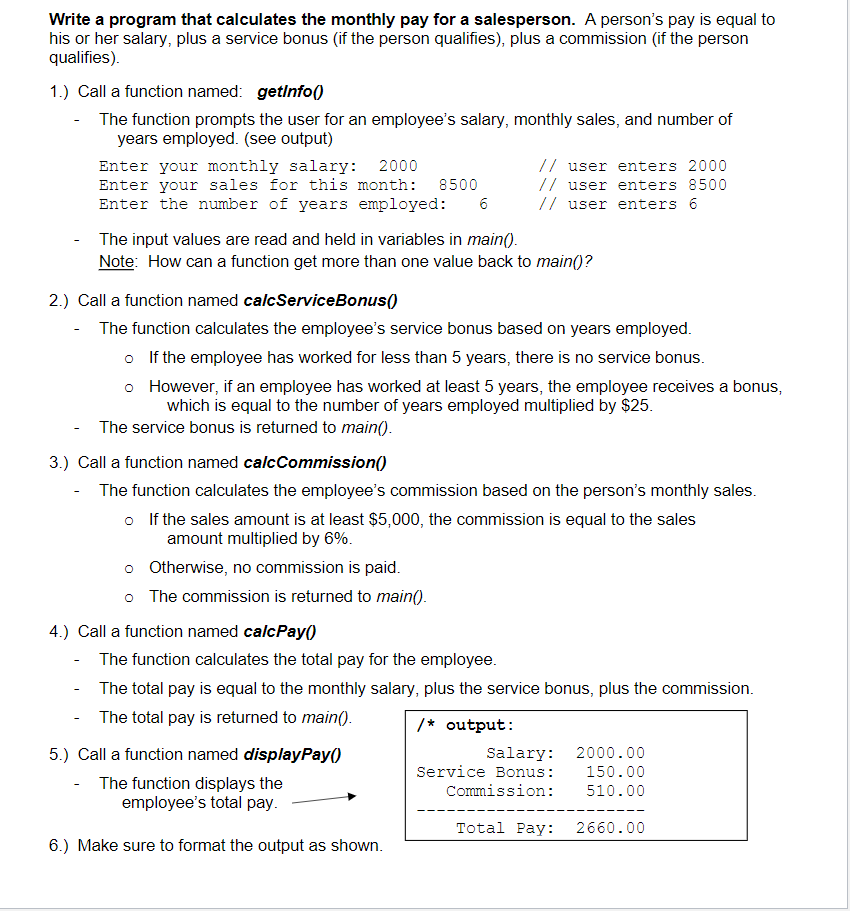
Transcribed Image Text:Write a program that calculates the monthly pay for a salesperson. A person's pay is equal to
his or her salary, plus a service bonus (if the person qualifies), plus a commission (if the person
qualifies).
1.) Call a function named: getlnfo()
The function prompts the user for an employee's salary, monthly sales, and number of
years employed. (see output)
Enter your monthly salary: 2000
Enter your sales for this month: 8500
Enter the number of years employed:
// user enters 2000
// user enters 8500
// user enters 6
6
The input values are read and held in variables in main().
Note: How can a function get more than one value back to main()?
2.) Call a function named calcServiceBonus()
The function calculates the employee's service bonus based on years employed.
o If the employee has worked for less than 5 years, there is no service bonus.
o However, if an employee has worked at least 5 years, the employee receives a bonus,
which is equal to the number of years employed multiplied by $25.
The service bonus is returned to main().
3.) Call a function named calcCommission()
The function calculates the employee's commission based on the person's monthly sales.
o If the sales amount is at least $5,000, the commission is equal to the sales
amount multiplied by 6%.
o Otherwise, no commission is paid.
o The commission is returned to main().
4.) Call a function named calcPay0
The function calculates the total pay for the employee.
The total pay is equal to the monthly salary, plus the service bonus, plus the commission.
- The total pay is returned to main().
/* output:
5.) Call a function named displayPay()
Salary: 2000.00
Service Bonus:
150.00
The function displays the
employee's total pay.
Commission:
510.00
Total Pay:
2660.00
6.) Make sure to format the output as shown.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
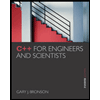
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
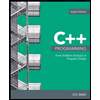
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
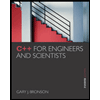
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
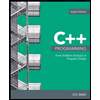
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning