Write a program that computes weekly hours for each employee. Store the weekly hours for all employees in a two-dimensional array. Each row records an employee's seven-day work hours with seven columns. For example, the following array stores the work hours for eight employees. Display employees and the total hours of each employee in decreasing order of the total hours. Su M T W Sa Employee0 2 4 3 4 8 Employeel 7 3 4 3 4 Employee2 3 3 4 3 2 Employee39 3 4 7 1 Employee4 3 5 4 3 8 Employee53 4 4 6 4 Employee6 3 7 4 8 4 Employee7 6 3 5 9 2 9 Requirements: 1. Design a method that takes the 2-D array of weekly hours of all employees as an input parameter, calculates the sum of the total hours of all employees and returns the total hour results in an array. 2. Design a method to sort the above returned array in Requirement 1 in descending order by selection sort. • Important: when sorting the array, the program should keep track of which employee corresponds to how many work hours. Therefore, you should store the employee information in an array and return it as a result of your sort method. 3. Design a method to display the results of employees and their total hours in decreasing order. The sample output for the above example is as follows: C:\WINDOWS\system32\.cmd.exe Employee?: 41 hours Employee6: 37 hours Employee: 34 hours Employee 4: 32 hours Employee3: 31 hours Employee5: 28 houre Employeel: 28 hours Employee2: 20 hours Press any key to continue 4. Design a main method that uses the short hand initializer to initialize the 2-D array of weekly hours of all employees and invokes all the three methods in Requirements 1-3 in a sequence. RS R 5 3 3 نیا نیا نیا نبات 3 6 3 3 F43 F 8 4 2 4 3 487
Write a program that computes weekly hours for each employee. Store the weekly hours for all employees in a two-dimensional array. Each row records an employee's seven-day work hours with seven columns. For example, the following array stores the work hours for eight employees. Display employees and the total hours of each employee in decreasing order of the total hours. Su M T W Sa Employee0 2 4 3 4 8 Employeel 7 3 4 3 4 Employee2 3 3 4 3 2 Employee39 3 4 7 1 Employee4 3 5 4 3 8 Employee53 4 4 6 4 Employee6 3 7 4 8 4 Employee7 6 3 5 9 2 9 Requirements: 1. Design a method that takes the 2-D array of weekly hours of all employees as an input parameter, calculates the sum of the total hours of all employees and returns the total hour results in an array. 2. Design a method to sort the above returned array in Requirement 1 in descending order by selection sort. • Important: when sorting the array, the program should keep track of which employee corresponds to how many work hours. Therefore, you should store the employee information in an array and return it as a result of your sort method. 3. Design a method to display the results of employees and their total hours in decreasing order. The sample output for the above example is as follows: C:\WINDOWS\system32\.cmd.exe Employee?: 41 hours Employee6: 37 hours Employee: 34 hours Employee 4: 32 hours Employee3: 31 hours Employee5: 28 houre Employeel: 28 hours Employee2: 20 hours Press any key to continue 4. Design a main method that uses the short hand initializer to initialize the 2-D array of weekly hours of all employees and invokes all the three methods in Requirements 1-3 in a sequence. RS R 5 3 3 نیا نیا نیا نبات 3 6 3 3 F43 F 8 4 2 4 3 487
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section10.3: Pointer Arithmetic
Problem 4E
Related questions
Question
Need help writing the program and getting the same display result. Attatching the instruction with images.
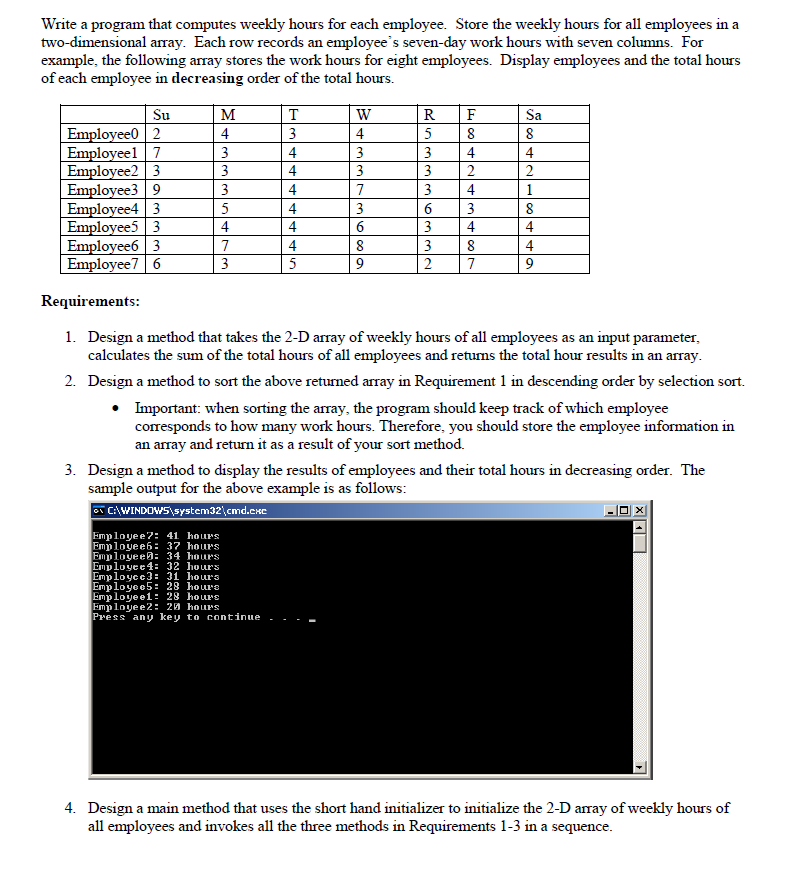
Transcribed Image Text:Write a program that computes weekly hours for each employee. Store the weekly hours for all employees in a
two-dimensional array. Each row records an employee's seven-day work hours with seven columns. For
example, the following array stores the work hours for eight employees. Display employees and the total hours
of each employee in decreasing order of the total hours.
Su
M
T
W
R
F
Sa
Employee0 2
4
3
4
5
8
8
Employeel 7
3
4
3
3
4
4
Employee2 3
3
4
3
3
2
2
Employee3 9
3
4
3
4
1
Employee4 3
4
3
6
3
8
Employee5 3
4
6
3
4
4
Employee6 3
7
4
8
3
8
4
Employee7 6
3
5
9
2 7
9
Requirements:
1. Design a method that takes the 2-D array of weekly hours of all employees as an input parameter,
calculates the sum of the total hours of all employees and returns the total hour results in an array.
2. Design a method to sort the above returned array in Requirement 1 in descending order by selection sort.
• Important: when sorting the array, the program should keep track of which employee
corresponds to how many work hours. Therefore, you should store the employee information in
an array and return it as a result of your sort method.
3. Design a method to display the results of employees and their total hours in decreasing order. The
sample output for the above example is as follows:
C:\WINDOWS\system32\cmd.exe
Employee?: 41 hours
Employee6: 37 hours
Employee: 34 hours
Employee 4: 32 hours
Employee3: 31 hours
Employee5: 28 hours
Employee1: 28 hours
Employee2: 20 hours
Press any key to continue
4. Design a main method that uses the short hand initializer to initialize the 2-D array of weekly hours of
all employees and invokes all the three methods in Requirements 1-3 in a sequence.
5
4
7
|L
![package homework;
public class HW8 {
public static void main(String[] args) {
int[][] workHours = {
{2, 4, 3, 4, 5, 8, 8},
{7, 3, 4, 3, 3, 4, 4),
(3, 3, 4, 3, 3, 2, 2),
{9, 3, 4, 7, 3, 4, 1},
{3, 5, 4, 3, 3, 8},
(3, 4, 4, 6, 3, 4, 4),
{3, 7, 4, 8, 3, 8, 4},
{6, 3, 5, 9, 2, 7, 9)
};
int[] sumArray = calculatesum (workHours);
int[] indexArray = decreasingsort (sumArray);
displayArray (indexArray, sumArray);
}
public static int[] calculatesum (int[][] array) {
//Please write your code here
}
public static int[] decreasingsort (int[] array) {
//Please write your code here
}
public static void display Array (int[] indexArray, int[] array) {
/*Please write your code here*/
System.out.println("Employee" + indexArray[i] + + array[i] +
"hours");
}
Finally, your code's formatting should look exactly like the output mentioned below and should be able to
run/compile.
Employee7: 41 hours
Employee6: 37 hours
Employeee: 34 hours
Employee4: 32 hours
Employee3: 31 hours
Employees: 28 hours
Employee1: 28 hours
Employee2: 20 hours](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2Fff825fc3-8162-400a-9c7b-e762c004489f%2Fsavvuy_processed.png&w=3840&q=75)
Transcribed Image Text:package homework;
public class HW8 {
public static void main(String[] args) {
int[][] workHours = {
{2, 4, 3, 4, 5, 8, 8},
{7, 3, 4, 3, 3, 4, 4),
(3, 3, 4, 3, 3, 2, 2),
{9, 3, 4, 7, 3, 4, 1},
{3, 5, 4, 3, 3, 8},
(3, 4, 4, 6, 3, 4, 4),
{3, 7, 4, 8, 3, 8, 4},
{6, 3, 5, 9, 2, 7, 9)
};
int[] sumArray = calculatesum (workHours);
int[] indexArray = decreasingsort (sumArray);
displayArray (indexArray, sumArray);
}
public static int[] calculatesum (int[][] array) {
//Please write your code here
}
public static int[] decreasingsort (int[] array) {
//Please write your code here
}
public static void display Array (int[] indexArray, int[] array) {
/*Please write your code here*/
System.out.println("Employee" + indexArray[i] + + array[i] +
"hours");
}
Finally, your code's formatting should look exactly like the output mentioned below and should be able to
run/compile.
Employee7: 41 hours
Employee6: 37 hours
Employeee: 34 hours
Employee4: 32 hours
Employee3: 31 hours
Employees: 28 hours
Employee1: 28 hours
Employee2: 20 hours
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
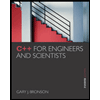
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
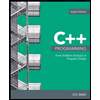
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
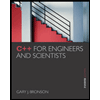
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
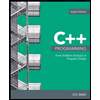
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning