Write a program that does the following: • Read in a height in feet and inches (feet should be an integer, while inches should be a float). • Output the equivalent height in meters (as a float). You must use at least three functions: 1. One for input. a. Name the function: input b. This function should prompt the User for the feet and inches within the body of the function. c. It will then return them as By Reference arguments through the parameter list. d. The datatype of the function will be void since the data is returned through the parameter list. e. In both the function and the function prototype name the feet variable intFeet and the inches variable intinches. calculating. a. Name the function: convert 2. One for b. This function should receive the feet and inches through the parameter list as By Value arguments and return the converted metric value back through the function name. c. The datatype of the function will be float since the converted value is in meters and is returned through the function name. d. Declare a variable within the function that the converted value will be stored in. i. This variable will be a float. ii. Name this value: convertedValue e. In both the function and the function prototype name the feet variable intFeet and the inches variable intinches. 3. One for output. a. Name the function: printResult b. This function will receive the metric value through the parameter list and print out the value. c. The datatype of the function will be void since nothing is returned by the function. d. In both the function and the function prototype name the metric variable metricHeight. In main: • Declare the following variables: o feet: This will be an integer. o inches: This will be a float. o meters: This will be a float. • Call the input function and send it feet and inches. • Call the convert function and send it feet and inches. o Assign the value returned by convert to meters • Call the printResult function and send it meters. Include: 1. A loop that lets the user repeat this computation for new input values. 2. When each computation is finished, the program will ask the user whether he/she wants to compute again. Note: One foot = 0.3048 meters. One inch is equivalent to 2.54 centimeters.
Write a program that does the following: • Read in a height in feet and inches (feet should be an integer, while inches should be a float). • Output the equivalent height in meters (as a float). You must use at least three functions: 1. One for input. a. Name the function: input b. This function should prompt the User for the feet and inches within the body of the function. c. It will then return them as By Reference arguments through the parameter list. d. The datatype of the function will be void since the data is returned through the parameter list. e. In both the function and the function prototype name the feet variable intFeet and the inches variable intinches. calculating. a. Name the function: convert 2. One for b. This function should receive the feet and inches through the parameter list as By Value arguments and return the converted metric value back through the function name. c. The datatype of the function will be float since the converted value is in meters and is returned through the function name. d. Declare a variable within the function that the converted value will be stored in. i. This variable will be a float. ii. Name this value: convertedValue e. In both the function and the function prototype name the feet variable intFeet and the inches variable intinches. 3. One for output. a. Name the function: printResult b. This function will receive the metric value through the parameter list and print out the value. c. The datatype of the function will be void since nothing is returned by the function. d. In both the function and the function prototype name the metric variable metricHeight. In main: • Declare the following variables: o feet: This will be an integer. o inches: This will be a float. o meters: This will be a float. • Call the input function and send it feet and inches. • Call the convert function and send it feet and inches. o Assign the value returned by convert to meters • Call the printResult function and send it meters. Include: 1. A loop that lets the user repeat this computation for new input values. 2. When each computation is finished, the program will ask the user whether he/she wants to compute again. Note: One foot = 0.3048 meters. One inch is equivalent to 2.54 centimeters.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 12PE: Write a program that takes as input five numbers and outputs the mean (average) and standard...
Related questions
Question
C++ Please
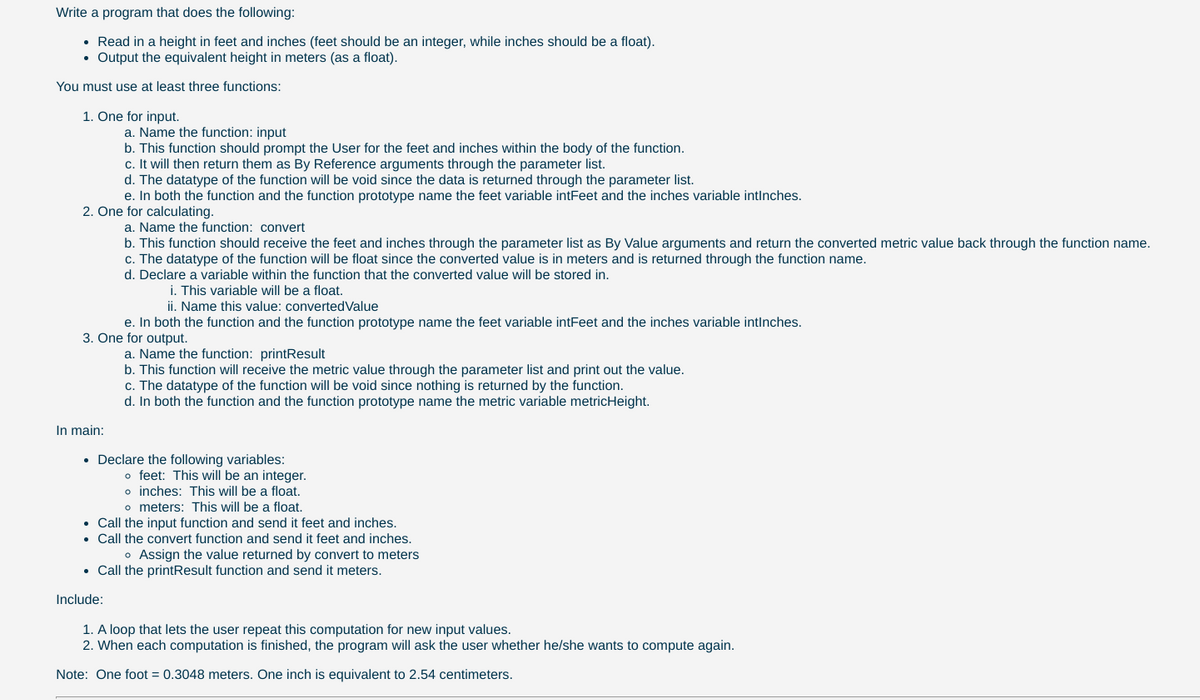
Transcribed Image Text:Write a program that does the following:
• Read in a height in feet and inches (feet should be an integer, while inches should be a float).
• Output the equivalent height in meters (as a float).
You must use at least three functions:
1. One for input.
a. Name the function: input
b. This function should prompt the User for the feet and inches within the body of the function.
c. It will then return them as By Reference arguments through the parameter list.
d. The datatype of the function will be void since the data is returned through the parameter list.
e. In both the function and the function prototype name the feet variable intFeet and the inches variable intinches.
2. One for calculating.
a. Name the function: convert
b. This function should receive the feet and inches through the parameter list as By Value arguments and return the converted metric value back through the function name.
c. The datatype of the function will be float since the converted value is in meters and is returned through the function name.
d. Declare a variable within the function that the converted value will be stored in.
i. This variable will be a float.
ii. Name this value: convertedValue
e. In both the function and the function prototype name the feet variable intFeet and the inches variable intlnches.
3. One for output.
a. Name the function: printResult
b. This function will receive the metric value through the parameter list and print out the value.
datatype of the
will
void since nothing
eturned by the functi
C.
d. In both the function and the function prototype name the metric variable metricHeight.
In main:
• Declare the following variables:
o feet: This will be an integer.
o inches: This will be a float.
o meters: This will be a float.
Call the input function and send it feet and inches.
Call the convert function and send it feet and inches.
o Assign the value returned by convert to meters
• Call the printResult function and send it meters.
Include:
1. A loop that lets the user repeat this computation for new input values.
2. When each computation is finished, the program will ask the user whether he/she wants to compute again.
Note: One foot = 0.3048 meters. One inch is equivalent to 2.54 centimeters.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
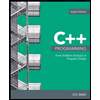
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
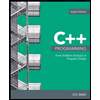
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning