Write a program that generates n random integers between 0 and 9 and displays the count for each number. (Hint: Use an array of ten integers, say count, to store the counts for the number of Os, 1s, ..., 9s.) A modular program is expected-use Methods. The program specifications are as below. In the main() method, declare an int array of size 10, named cnums. Implement a method fillCnums(int[] cnt) that initializes the array to zero. Implement a method countDigits(int[] cnt, int n) that accepts the cnums array and an integer n. The method should generate n random numbers in the range of 0 to 9. It should keep a count of how many times each number, 0 to 9; is generated in the array cnums. Implement a method printCnums(int[] cnt) to print the cnums array. Note, print "time" or "times" - which ever is appropriate. Use basic structured programming and procedural programming. Write a main() method that declares the cnums array. Invokes the countDigits() method with n = 10 followed by invoking the printCnums(). Then invokes the count Digits() method with n = 100 followed by printCnums(). And again, invokes the countDigits() method with n = 1000 followed by printCnums(). Make sure you invoke the fillCnums(int[] cnt) method at appropriate times. And write out the heading for each set, n = 10, 100, and 1000. Documentation. Includes your name, create date and purpose of lab.
Write a program that generates n random integers between 0 and 9 and displays the count for each number. (Hint: Use an array of ten integers, say count, to store the counts for the number of Os, 1s, ..., 9s.) A modular program is expected-use Methods. The program specifications are as below. In the main() method, declare an int array of size 10, named cnums. Implement a method fillCnums(int[] cnt) that initializes the array to zero. Implement a method countDigits(int[] cnt, int n) that accepts the cnums array and an integer n. The method should generate n random numbers in the range of 0 to 9. It should keep a count of how many times each number, 0 to 9; is generated in the array cnums. Implement a method printCnums(int[] cnt) to print the cnums array. Note, print "time" or "times" - which ever is appropriate. Use basic structured programming and procedural programming. Write a main() method that declares the cnums array. Invokes the countDigits() method with n = 10 followed by invoking the printCnums(). Then invokes the count Digits() method with n = 100 followed by printCnums(). And again, invokes the countDigits() method with n = 1000 followed by printCnums(). Make sure you invoke the fillCnums(int[] cnt) method at appropriate times. And write out the heading for each set, n = 10, 100, and 1000. Documentation. Includes your name, create date and purpose of lab.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Sample run:
command>java Lab01
Invoke countDigits() for n = 10 values
O occurs 2 times
1 occurs 2 times
2 occurs
0 time
3 occurs
0 time
4 occurs
0 time
5 occurs
0 time
6 occurs
1 time
7 occurs
2 times
8 occurs
2 times
9 occurs 1 time
Invoke countDigits() for n = 100 values
O occurs 5 times
1 occurs 11 times
2 occurs
6 times
3 occurs 17 times
4 occurs 11 times
5 occurs
8 times
6 occurs
9 times
7 occurs 10 times
8 occurs 14 times
9 occurs
9 times
Invoke countDigits() for n = 1000 values
O occurs 89 times
1 occurs 92 times
2 occurs 104 times
3 occurs 97 times
4 occurs 98 times
5 occurs 106 times
6 occurs 104 times
7 occurs 92 times
8 occurs 90 times
9 occurs 128 times
![Lab01 (Count single digits)
Write a program that generates n random integers between 0 and 9 and displays the count for each number. (Hint: Use an array of ten integers, say count, to store the counts for the
number of Os, 1s, ..., 9s.)
A modular program is expected - use Methods. The program specifications are as below.
In the main() method, declare an int array of size 10, named cnums.
Implement a method fillCnums(int[] cnt) that initializes the array to zero.
Implement a method countDigits(int[] cnt, int n) that accepts the cnums array and an integer n. The method should generate n random numbers in the range of 0 to 9.
It should keep a count of how many times each number, 0 to 9; is generated in the array cnums.
Implement a method printCnums(int[] cnt) to print the cnums array. Note, print "time" or "times" - which ever is appropriate.
Use basic structured programming and procedural programming.
Write a main() method that declares the cnums array. Invokes the countDigits() method with n = 10 followed by invoking the printCnums(). Then invokes the
count Digits() method with n = 100 followed by printCnums(). And again, invokes the countDigits() method with n = 1000 followed by printCnums().
Make sure you invoke the fillCnums(int[] cnt) method at appropriate times. And write out the heading for each set, n = 10, 100, and 1000.
Documentation. Includes your name, create date and purpose of lab.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3cafa2c1-a76b-449e-9796-7de6b3b95bcc%2Fae50c329-bce2-4ea6-b6d5-de4a92cae8d9%2Ff6secul_processed.png&w=3840&q=75)
Transcribed Image Text:Lab01 (Count single digits)
Write a program that generates n random integers between 0 and 9 and displays the count for each number. (Hint: Use an array of ten integers, say count, to store the counts for the
number of Os, 1s, ..., 9s.)
A modular program is expected - use Methods. The program specifications are as below.
In the main() method, declare an int array of size 10, named cnums.
Implement a method fillCnums(int[] cnt) that initializes the array to zero.
Implement a method countDigits(int[] cnt, int n) that accepts the cnums array and an integer n. The method should generate n random numbers in the range of 0 to 9.
It should keep a count of how many times each number, 0 to 9; is generated in the array cnums.
Implement a method printCnums(int[] cnt) to print the cnums array. Note, print "time" or "times" - which ever is appropriate.
Use basic structured programming and procedural programming.
Write a main() method that declares the cnums array. Invokes the countDigits() method with n = 10 followed by invoking the printCnums(). Then invokes the
count Digits() method with n = 100 followed by printCnums(). And again, invokes the countDigits() method with n = 1000 followed by printCnums().
Make sure you invoke the fillCnums(int[] cnt) method at appropriate times. And write out the heading for each set, n = 10, 100, and 1000.
Documentation. Includes your name, create date and purpose of lab.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Recommended textbooks for you
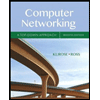
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
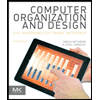
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
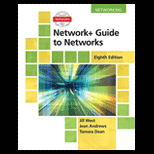
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
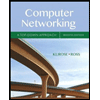
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
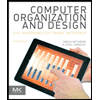
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
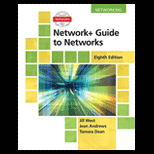
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
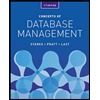
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
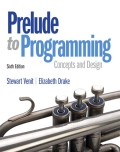
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
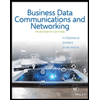
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY